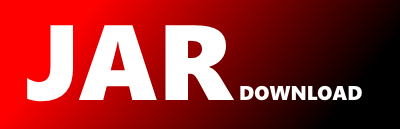
com.aliyun.sdk.service.ecs20140526.models.RenewElasticityAssurancesRequest Maven / Gradle / Ivy
Show all versions of alibabacloud-ecs20140526 Show documentation
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.sdk.service.ecs20140526.models;
import com.aliyun.sdk.gateway.pop.*;
import darabonba.core.*;
import darabonba.core.async.*;
import darabonba.core.sync.*;
import darabonba.core.client.*;
import darabonba.core.RequestModel;
import darabonba.core.TeaModel;
import com.aliyun.sdk.gateway.pop.models.*;
/**
*
* {@link RenewElasticityAssurancesRequest} extends {@link RequestModel}
*
* RenewElasticityAssurancesRequest
*/
public class RenewElasticityAssurancesRequest extends Request {
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("PrivatePoolOptions")
private PrivatePoolOptions privatePoolOptions;
@com.aliyun.core.annotation.Host
@com.aliyun.core.annotation.NameInMap("SourceRegionId")
private String sourceRegionId;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("AutoPay")
private Boolean autoPay;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("ClientToken")
private String clientToken;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("OwnerAccount")
private String ownerAccount;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("OwnerId")
private Long ownerId;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("Period")
private Integer period;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("PeriodUnit")
private String periodUnit;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("RegionId")
private String regionId;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("ResourceOwnerAccount")
private String resourceOwnerAccount;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("ResourceOwnerId")
private Long resourceOwnerId;
private RenewElasticityAssurancesRequest(Builder builder) {
super(builder);
this.privatePoolOptions = builder.privatePoolOptions;
this.sourceRegionId = builder.sourceRegionId;
this.autoPay = builder.autoPay;
this.clientToken = builder.clientToken;
this.ownerAccount = builder.ownerAccount;
this.ownerId = builder.ownerId;
this.period = builder.period;
this.periodUnit = builder.periodUnit;
this.regionId = builder.regionId;
this.resourceOwnerAccount = builder.resourceOwnerAccount;
this.resourceOwnerId = builder.resourceOwnerId;
}
public static Builder builder() {
return new Builder();
}
public static RenewElasticityAssurancesRequest create() {
return builder().build();
}
@Override
public Builder toBuilder() {
return new Builder(this);
}
/**
* @return privatePoolOptions
*/
public PrivatePoolOptions getPrivatePoolOptions() {
return this.privatePoolOptions;
}
/**
* @return sourceRegionId
*/
public String getSourceRegionId() {
return this.sourceRegionId;
}
/**
* @return autoPay
*/
public Boolean getAutoPay() {
return this.autoPay;
}
/**
* @return clientToken
*/
public String getClientToken() {
return this.clientToken;
}
/**
* @return ownerAccount
*/
public String getOwnerAccount() {
return this.ownerAccount;
}
/**
* @return ownerId
*/
public Long getOwnerId() {
return this.ownerId;
}
/**
* @return period
*/
public Integer getPeriod() {
return this.period;
}
/**
* @return periodUnit
*/
public String getPeriodUnit() {
return this.periodUnit;
}
/**
* @return regionId
*/
public String getRegionId() {
return this.regionId;
}
/**
* @return resourceOwnerAccount
*/
public String getResourceOwnerAccount() {
return this.resourceOwnerAccount;
}
/**
* @return resourceOwnerId
*/
public Long getResourceOwnerId() {
return this.resourceOwnerId;
}
public static final class Builder extends Request.Builder {
private PrivatePoolOptions privatePoolOptions;
private String sourceRegionId;
private Boolean autoPay;
private String clientToken;
private String ownerAccount;
private Long ownerId;
private Integer period;
private String periodUnit;
private String regionId;
private String resourceOwnerAccount;
private Long resourceOwnerId;
private Builder() {
super();
}
private Builder(RenewElasticityAssurancesRequest request) {
super(request);
this.privatePoolOptions = request.privatePoolOptions;
this.sourceRegionId = request.sourceRegionId;
this.autoPay = request.autoPay;
this.clientToken = request.clientToken;
this.ownerAccount = request.ownerAccount;
this.ownerId = request.ownerId;
this.period = request.period;
this.periodUnit = request.periodUnit;
this.regionId = request.regionId;
this.resourceOwnerAccount = request.resourceOwnerAccount;
this.resourceOwnerId = request.resourceOwnerId;
}
/**
* PrivatePoolOptions.
*/
public Builder privatePoolOptions(PrivatePoolOptions privatePoolOptions) {
this.putQueryParameter("PrivatePoolOptions", privatePoolOptions);
this.privatePoolOptions = privatePoolOptions;
return this;
}
/**
* SourceRegionId.
*/
public Builder sourceRegionId(String sourceRegionId) {
this.putHostParameter("SourceRegionId", sourceRegionId);
this.sourceRegionId = sourceRegionId;
return this;
}
/**
* Specifies whether to enable automatic payment. Valid values:
*
* - true
* - false
*
* Default value: true.
*
* example:
* true
*/
public Builder autoPay(Boolean autoPay) {
this.putQueryParameter("AutoPay", autoPay);
this.autoPay = autoPay;
return this;
}
/**
* The client token that is used to ensure the idempotence of the request. You can use the client to generate the token, but you must make sure that the token is unique among different requests.
* The token
can contain only ASCII characters and cannot exceed 64 characters in length. For more information, see How to ensure idempotence.
*
* example:
* 123e4567-e89b-12d3-a456-426655440000
*/
public Builder clientToken(String clientToken) {
this.putQueryParameter("ClientToken", clientToken);
this.clientToken = clientToken;
return this;
}
/**
* OwnerAccount.
*/
public Builder ownerAccount(String ownerAccount) {
this.putQueryParameter("OwnerAccount", ownerAccount);
this.ownerAccount = ownerAccount;
return this;
}
/**
* OwnerId.
*/
public Builder ownerId(Long ownerId) {
this.putQueryParameter("OwnerId", ownerId);
this.ownerId = ownerId;
return this;
}
/**
* The renewal duration. The unit of the renewal duration is determined by the PeriodUnit
value. Valid values:
*
* - Valid values if you set
PeriodUnit
to Month
: 1, 2, 3, 4, 5, 6, 7, 8, and 9.
* - Valid values if you set
PeriodUnit
to Year
: 1, 2, and 3.
*
* Default value: 1.
*
* example:
* 1
*/
public Builder period(Integer period) {
this.putQueryParameter("Period", period);
this.period = period;
return this;
}
/**
* The unit of the renewal duration. Valid values:
*
* - Month
* - Year
*
* Default value: Year.
*
* example:
* Year
*/
public Builder periodUnit(String periodUnit) {
this.putQueryParameter("PeriodUnit", periodUnit);
this.periodUnit = periodUnit;
return this;
}
/**
* The region ID of the elasticity assurance.
* You can call the DescribeRegions operation to query the most recent region list.
*
* example:
* cn-hangzhou
*/
public Builder regionId(String regionId) {
this.putQueryParameter("RegionId", regionId);
this.regionId = regionId;
return this;
}
/**
* ResourceOwnerAccount.
*/
public Builder resourceOwnerAccount(String resourceOwnerAccount) {
this.putQueryParameter("ResourceOwnerAccount", resourceOwnerAccount);
this.resourceOwnerAccount = resourceOwnerAccount;
return this;
}
/**
* ResourceOwnerId.
*/
public Builder resourceOwnerId(Long resourceOwnerId) {
this.putQueryParameter("ResourceOwnerId", resourceOwnerId);
this.resourceOwnerId = resourceOwnerId;
return this;
}
@Override
public RenewElasticityAssurancesRequest build() {
return new RenewElasticityAssurancesRequest(this);
}
}
/**
*
* {@link RenewElasticityAssurancesRequest} extends {@link TeaModel}
*
* RenewElasticityAssurancesRequest
*/
public static class PrivatePoolOptions extends TeaModel {
@com.aliyun.core.annotation.NameInMap("Id")
private java.util.List id;
private PrivatePoolOptions(Builder builder) {
this.id = builder.id;
}
public static Builder builder() {
return new Builder();
}
public static PrivatePoolOptions create() {
return builder().build();
}
/**
* @return id
*/
public java.util.List getId() {
return this.id;
}
public static final class Builder {
private java.util.List id;
/**
* The IDs of elasticity assurances.
* Limits: You can renew up to 20 elasticity assurances at a time.
* You can call the DescribeElasticityAssurances operation to query the elasticity assurances that you purchased.
*/
public Builder id(java.util.List id) {
this.id = id;
return this;
}
public PrivatePoolOptions build() {
return new PrivatePoolOptions(this);
}
}
}
}