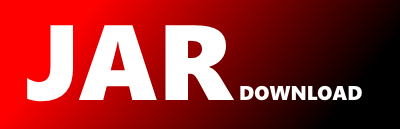
com.aliyun.sdk.service.ecs20140526.models.StartInstanceRequest Maven / Gradle / Ivy
Show all versions of alibabacloud-ecs20140526 Show documentation
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.sdk.service.ecs20140526.models;
import com.aliyun.sdk.gateway.pop.*;
import darabonba.core.*;
import darabonba.core.async.*;
import darabonba.core.sync.*;
import darabonba.core.client.*;
import darabonba.core.RequestModel;
import darabonba.core.TeaModel;
import com.aliyun.sdk.gateway.pop.models.*;
/**
*
* {@link StartInstanceRequest} extends {@link RequestModel}
*
* StartInstanceRequest
*/
public class StartInstanceRequest extends Request {
@com.aliyun.core.annotation.Host
@com.aliyun.core.annotation.NameInMap("SourceRegionId")
private String sourceRegionId;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("DryRun")
private Boolean dryRun;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("InitLocalDisk")
private Boolean initLocalDisk;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("InstanceId")
@com.aliyun.core.annotation.Validation(required = true)
private String instanceId;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("OwnerAccount")
private String ownerAccount;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("OwnerId")
private Long ownerId;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("ResourceOwnerAccount")
private String resourceOwnerAccount;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("ResourceOwnerId")
private Long resourceOwnerId;
private StartInstanceRequest(Builder builder) {
super(builder);
this.sourceRegionId = builder.sourceRegionId;
this.dryRun = builder.dryRun;
this.initLocalDisk = builder.initLocalDisk;
this.instanceId = builder.instanceId;
this.ownerAccount = builder.ownerAccount;
this.ownerId = builder.ownerId;
this.resourceOwnerAccount = builder.resourceOwnerAccount;
this.resourceOwnerId = builder.resourceOwnerId;
}
public static Builder builder() {
return new Builder();
}
public static StartInstanceRequest create() {
return builder().build();
}
@Override
public Builder toBuilder() {
return new Builder(this);
}
/**
* @return sourceRegionId
*/
public String getSourceRegionId() {
return this.sourceRegionId;
}
/**
* @return dryRun
*/
public Boolean getDryRun() {
return this.dryRun;
}
/**
* @return initLocalDisk
*/
public Boolean getInitLocalDisk() {
return this.initLocalDisk;
}
/**
* @return instanceId
*/
public String getInstanceId() {
return this.instanceId;
}
/**
* @return ownerAccount
*/
public String getOwnerAccount() {
return this.ownerAccount;
}
/**
* @return ownerId
*/
public Long getOwnerId() {
return this.ownerId;
}
/**
* @return resourceOwnerAccount
*/
public String getResourceOwnerAccount() {
return this.resourceOwnerAccount;
}
/**
* @return resourceOwnerId
*/
public Long getResourceOwnerId() {
return this.resourceOwnerId;
}
public static final class Builder extends Request.Builder {
private String sourceRegionId;
private Boolean dryRun;
private Boolean initLocalDisk;
private String instanceId;
private String ownerAccount;
private Long ownerId;
private String resourceOwnerAccount;
private Long resourceOwnerId;
private Builder() {
super();
}
private Builder(StartInstanceRequest request) {
super(request);
this.sourceRegionId = request.sourceRegionId;
this.dryRun = request.dryRun;
this.initLocalDisk = request.initLocalDisk;
this.instanceId = request.instanceId;
this.ownerAccount = request.ownerAccount;
this.ownerId = request.ownerId;
this.resourceOwnerAccount = request.resourceOwnerAccount;
this.resourceOwnerId = request.resourceOwnerId;
}
/**
* SourceRegionId.
*/
public Builder sourceRegionId(String sourceRegionId) {
this.putHostParameter("SourceRegionId", sourceRegionId);
this.sourceRegionId = sourceRegionId;
return this;
}
/**
* Specifies whether to perform only a dry run, without performing the actual request. Valid values:
*
* - true: performs only a dry run. The system checks the AccessKey pair, the permissions of the RAM user, and the required parameters. If the request fails the dry run, an error message is returned. If the request passes the dry run, the DryRunOperation error code is returned.
* - false: performs a dry run and performs the actual request. If the request passes the dry run, a 2xx HTTP status code is returned and the operation is performed.
*
* Default value: false.
*
* example:
* true
*/
public Builder dryRun(Boolean dryRun) {
this.putQueryParameter("DryRun", dryRun);
this.dryRun = dryRun;
return this;
}
/**
* Specifies whether to restore the ECS instance to the initial health state on startup if a local disk fails. This parameter is applicable to ECS instances that are equipped with local disks, such as d1, i1, and i2 instances. Valid values:
*
* true: restores the ECS instance to the initial health state on startup.
* **
* Warning: After the ECS instance is restored to the initial health state, data stored on the local disks of the instance is lost.
*
* false: does not restore the ECS instance to the initial health state on startup. The instance remains in the current state.
*
*
* Default value: false.
*
* example:
* true
*/
public Builder initLocalDisk(Boolean initLocalDisk) {
this.putQueryParameter("InitLocalDisk", initLocalDisk);
this.initLocalDisk = initLocalDisk;
return this;
}
/**
* The ID of the instance that you want to start.
* This parameter is required.
*
* example:
* i-bp67acfmxazb4p****
*/
public Builder instanceId(String instanceId) {
this.putQueryParameter("InstanceId", instanceId);
this.instanceId = instanceId;
return this;
}
/**
* OwnerAccount.
*/
public Builder ownerAccount(String ownerAccount) {
this.putQueryParameter("OwnerAccount", ownerAccount);
this.ownerAccount = ownerAccount;
return this;
}
/**
* OwnerId.
*/
public Builder ownerId(Long ownerId) {
this.putQueryParameter("OwnerId", ownerId);
this.ownerId = ownerId;
return this;
}
/**
* ResourceOwnerAccount.
*/
public Builder resourceOwnerAccount(String resourceOwnerAccount) {
this.putQueryParameter("ResourceOwnerAccount", resourceOwnerAccount);
this.resourceOwnerAccount = resourceOwnerAccount;
return this;
}
/**
* ResourceOwnerId.
*/
public Builder resourceOwnerId(Long resourceOwnerId) {
this.putQueryParameter("ResourceOwnerId", resourceOwnerId);
this.resourceOwnerId = resourceOwnerId;
return this;
}
@Override
public StartInstanceRequest build() {
return new StartInstanceRequest(this);
}
}
}