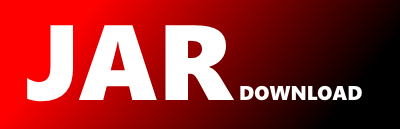
com.aliyun.sdk.service.ecs20140526.models.StopInstanceRequest Maven / Gradle / Ivy
Show all versions of alibabacloud-ecs20140526 Show documentation
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.sdk.service.ecs20140526.models;
import com.aliyun.sdk.gateway.pop.*;
import darabonba.core.*;
import darabonba.core.async.*;
import darabonba.core.sync.*;
import darabonba.core.client.*;
import darabonba.core.RequestModel;
import darabonba.core.TeaModel;
import com.aliyun.sdk.gateway.pop.models.*;
/**
*
* {@link StopInstanceRequest} extends {@link RequestModel}
*
* StopInstanceRequest
*/
public class StopInstanceRequest extends Request {
@com.aliyun.core.annotation.Host
@com.aliyun.core.annotation.NameInMap("SourceRegionId")
private String sourceRegionId;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("ConfirmStop")
private Boolean confirmStop;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("DryRun")
private Boolean dryRun;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("ForceStop")
private Boolean forceStop;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("Hibernate")
private Boolean hibernate;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("InstanceId")
@com.aliyun.core.annotation.Validation(required = true)
private String instanceId;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("OwnerAccount")
private String ownerAccount;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("OwnerId")
private Long ownerId;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("ResourceOwnerAccount")
private String resourceOwnerAccount;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("ResourceOwnerId")
private Long resourceOwnerId;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("StoppedMode")
private String stoppedMode;
private StopInstanceRequest(Builder builder) {
super(builder);
this.sourceRegionId = builder.sourceRegionId;
this.confirmStop = builder.confirmStop;
this.dryRun = builder.dryRun;
this.forceStop = builder.forceStop;
this.hibernate = builder.hibernate;
this.instanceId = builder.instanceId;
this.ownerAccount = builder.ownerAccount;
this.ownerId = builder.ownerId;
this.resourceOwnerAccount = builder.resourceOwnerAccount;
this.resourceOwnerId = builder.resourceOwnerId;
this.stoppedMode = builder.stoppedMode;
}
public static Builder builder() {
return new Builder();
}
public static StopInstanceRequest create() {
return builder().build();
}
@Override
public Builder toBuilder() {
return new Builder(this);
}
/**
* @return sourceRegionId
*/
public String getSourceRegionId() {
return this.sourceRegionId;
}
/**
* @return confirmStop
*/
public Boolean getConfirmStop() {
return this.confirmStop;
}
/**
* @return dryRun
*/
public Boolean getDryRun() {
return this.dryRun;
}
/**
* @return forceStop
*/
public Boolean getForceStop() {
return this.forceStop;
}
/**
* @return hibernate
*/
public Boolean getHibernate() {
return this.hibernate;
}
/**
* @return instanceId
*/
public String getInstanceId() {
return this.instanceId;
}
/**
* @return ownerAccount
*/
public String getOwnerAccount() {
return this.ownerAccount;
}
/**
* @return ownerId
*/
public Long getOwnerId() {
return this.ownerId;
}
/**
* @return resourceOwnerAccount
*/
public String getResourceOwnerAccount() {
return this.resourceOwnerAccount;
}
/**
* @return resourceOwnerId
*/
public Long getResourceOwnerId() {
return this.resourceOwnerId;
}
/**
* @return stoppedMode
*/
public String getStoppedMode() {
return this.stoppedMode;
}
public static final class Builder extends Request.Builder {
private String sourceRegionId;
private Boolean confirmStop;
private Boolean dryRun;
private Boolean forceStop;
private Boolean hibernate;
private String instanceId;
private String ownerAccount;
private Long ownerId;
private String resourceOwnerAccount;
private Long resourceOwnerId;
private String stoppedMode;
private Builder() {
super();
}
private Builder(StopInstanceRequest request) {
super(request);
this.sourceRegionId = request.sourceRegionId;
this.confirmStop = request.confirmStop;
this.dryRun = request.dryRun;
this.forceStop = request.forceStop;
this.hibernate = request.hibernate;
this.instanceId = request.instanceId;
this.ownerAccount = request.ownerAccount;
this.ownerId = request.ownerId;
this.resourceOwnerAccount = request.resourceOwnerAccount;
this.resourceOwnerId = request.resourceOwnerId;
this.stoppedMode = request.stoppedMode;
}
/**
* SourceRegionId.
*/
public Builder sourceRegionId(String sourceRegionId) {
this.putHostParameter("SourceRegionId", sourceRegionId);
this.sourceRegionId = sourceRegionId;
return this;
}
/**
* This parameter will be removed in the future and is retained only to ensure compatibility. We recommend that you ignore this parameter.
*
* example:
* true
*/
public Builder confirmStop(Boolean confirmStop) {
this.putQueryParameter("ConfirmStop", confirmStop);
this.confirmStop = confirmStop;
return this;
}
/**
* Specifies whether to perform only a dry run, without performing the actual request. Valid values:
*
* - true: performs only a dry run. The system checks the request for potential issues, including missing parameter values, incorrect request syntax, service limits, and available ECS resources. If the request fails the dry run, an error message is returned. If the request passes the dry run, the
DryRunOperation
error code is returned.
* - false: performs a dry run and performs the actual request.
*
* Default value: false.
*
* example:
* true
*/
public Builder dryRun(Boolean dryRun) {
this.putQueryParameter("DryRun", dryRun);
this.dryRun = dryRun;
return this;
}
/**
* Specifies whether to forcefully stop the ECS instance. Valid values:
*
* - true: forcefully stops the ECS instance. If you set ForceStop to true, this operation is equivalent to a power-off operation. Cache data that is not written to storage devices on the instance is lost.
* - false: normally stops the ECS instance.
*
* Default value: false.
*
* example:
* false
*/
public Builder forceStop(Boolean forceStop) {
this.putQueryParameter("ForceStop", forceStop);
this.forceStop = forceStop;
return this;
}
/**
*
* This parameter is in invitational preview and is not publicly available.
*
*
* example:
* hide
*/
public Builder hibernate(Boolean hibernate) {
this.putQueryParameter("Hibernate", hibernate);
this.hibernate = hibernate;
return this;
}
/**
* The ID of the instance.
* This parameter is required.
*
* example:
* i-bp67acfmxazb4ph****
*/
public Builder instanceId(String instanceId) {
this.putQueryParameter("InstanceId", instanceId);
this.instanceId = instanceId;
return this;
}
/**
* OwnerAccount.
*/
public Builder ownerAccount(String ownerAccount) {
this.putQueryParameter("OwnerAccount", ownerAccount);
this.ownerAccount = ownerAccount;
return this;
}
/**
* OwnerId.
*/
public Builder ownerId(Long ownerId) {
this.putQueryParameter("OwnerId", ownerId);
this.ownerId = ownerId;
return this;
}
/**
* ResourceOwnerAccount.
*/
public Builder resourceOwnerAccount(String resourceOwnerAccount) {
this.putQueryParameter("ResourceOwnerAccount", resourceOwnerAccount);
this.resourceOwnerAccount = resourceOwnerAccount;
return this;
}
/**
* ResourceOwnerId.
*/
public Builder resourceOwnerId(Long resourceOwnerId) {
this.putQueryParameter("ResourceOwnerId", resourceOwnerId);
this.resourceOwnerId = resourceOwnerId;
return this;
}
/**
* The stop mode of the pay-as-you-go instance. Valid values:
*
* - StopCharging: economical mode. For information about how
StopCharging
takes effect, see the "Conditions for enabling economical mode" section in Economical mode.
* - KeepCharging: standard mode. You continue to be charged for instances that are stopped in standard mode.
*
* Default value: If the conditions for enabling the economical mode are met and you have enabled this mode in the ECS console, the default value is StopCharging. For more information, see the "Enable economical mode" section in Economical mode
. Otherwise, the default value is KeepCharging
.
*
* example:
* KeepCharging
*/
public Builder stoppedMode(String stoppedMode) {
this.putQueryParameter("StoppedMode", stoppedMode);
this.stoppedMode = stoppedMode;
return this;
}
@Override
public StopInstanceRequest build() {
return new StopInstanceRequest(this);
}
}
}