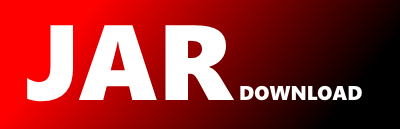
com.aliyun.sdk.service.ens20171110.models.DescribeEnsRouteTablesResponseBody Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of alibabacloud-ens20171110 Show documentation
Show all versions of alibabacloud-ens20171110 Show documentation
Alibaba Cloud Ens (20171110) Async SDK for Java
The newest version!
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.sdk.service.ens20171110.models;
import com.aliyun.sdk.gateway.pop.*;
import darabonba.core.*;
import darabonba.core.async.*;
import darabonba.core.sync.*;
import darabonba.core.client.*;
import darabonba.core.RequestModel;
import darabonba.core.TeaModel;
import com.aliyun.sdk.gateway.pop.models.*;
/**
*
* {@link DescribeEnsRouteTablesResponseBody} extends {@link TeaModel}
*
* DescribeEnsRouteTablesResponseBody
*/
public class DescribeEnsRouteTablesResponseBody extends TeaModel {
@com.aliyun.core.annotation.NameInMap("PageNumber")
private Integer pageNumber;
@com.aliyun.core.annotation.NameInMap("PageSize")
private Integer pageSize;
@com.aliyun.core.annotation.NameInMap("RequestId")
private String requestId;
@com.aliyun.core.annotation.NameInMap("RouteTables")
private java.util.List routeTables;
@com.aliyun.core.annotation.NameInMap("TotalCount")
private Integer totalCount;
private DescribeEnsRouteTablesResponseBody(Builder builder) {
this.pageNumber = builder.pageNumber;
this.pageSize = builder.pageSize;
this.requestId = builder.requestId;
this.routeTables = builder.routeTables;
this.totalCount = builder.totalCount;
}
public static Builder builder() {
return new Builder();
}
public static DescribeEnsRouteTablesResponseBody create() {
return builder().build();
}
/**
* @return pageNumber
*/
public Integer getPageNumber() {
return this.pageNumber;
}
/**
* @return pageSize
*/
public Integer getPageSize() {
return this.pageSize;
}
/**
* @return requestId
*/
public String getRequestId() {
return this.requestId;
}
/**
* @return routeTables
*/
public java.util.List getRouteTables() {
return this.routeTables;
}
/**
* @return totalCount
*/
public Integer getTotalCount() {
return this.totalCount;
}
public static final class Builder {
private Integer pageNumber;
private Integer pageSize;
private String requestId;
private java.util.List routeTables;
private Integer totalCount;
/**
* The page number.
*
* example:
* 1
*/
public Builder pageNumber(Integer pageNumber) {
this.pageNumber = pageNumber;
return this;
}
/**
* The number of entries per page.
*
* example:
* 30
*/
public Builder pageSize(Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* The ID of the request.
*
* example:
* DC51ACB0-460D-5CA0-BA2D-E1F3B5547AE9
*/
public Builder requestId(String requestId) {
this.requestId = requestId;
return this;
}
/**
* The information about the route tables.
*/
public Builder routeTables(java.util.List routeTables) {
this.routeTables = routeTables;
return this;
}
/**
* The total number of entries returned.
*
* example:
* 10
*/
public Builder totalCount(Integer totalCount) {
this.totalCount = totalCount;
return this;
}
public DescribeEnsRouteTablesResponseBody build() {
return new DescribeEnsRouteTablesResponseBody(this);
}
}
/**
*
* {@link DescribeEnsRouteTablesResponseBody} extends {@link TeaModel}
*
* DescribeEnsRouteTablesResponseBody
*/
public static class RouteTables extends TeaModel {
@com.aliyun.core.annotation.NameInMap("CreationTime")
private String creationTime;
@com.aliyun.core.annotation.NameInMap("EnsRegionId")
private String ensRegionId;
@com.aliyun.core.annotation.NameInMap("NetworkId")
private String networkId;
@com.aliyun.core.annotation.NameInMap("RouteTableId")
private String routeTableId;
@com.aliyun.core.annotation.NameInMap("RouteTableName")
private String routeTableName;
@com.aliyun.core.annotation.NameInMap("Status")
private String status;
@com.aliyun.core.annotation.NameInMap("Type")
private String type;
@com.aliyun.core.annotation.NameInMap("VSwitchIds")
private java.util.List vSwitchIds;
private RouteTables(Builder builder) {
this.creationTime = builder.creationTime;
this.ensRegionId = builder.ensRegionId;
this.networkId = builder.networkId;
this.routeTableId = builder.routeTableId;
this.routeTableName = builder.routeTableName;
this.status = builder.status;
this.type = builder.type;
this.vSwitchIds = builder.vSwitchIds;
}
public static Builder builder() {
return new Builder();
}
public static RouteTables create() {
return builder().build();
}
/**
* @return creationTime
*/
public String getCreationTime() {
return this.creationTime;
}
/**
* @return ensRegionId
*/
public String getEnsRegionId() {
return this.ensRegionId;
}
/**
* @return networkId
*/
public String getNetworkId() {
return this.networkId;
}
/**
* @return routeTableId
*/
public String getRouteTableId() {
return this.routeTableId;
}
/**
* @return routeTableName
*/
public String getRouteTableName() {
return this.routeTableName;
}
/**
* @return status
*/
public String getStatus() {
return this.status;
}
/**
* @return type
*/
public String getType() {
return this.type;
}
/**
* @return vSwitchIds
*/
public java.util.List getVSwitchIds() {
return this.vSwitchIds;
}
public static final class Builder {
private String creationTime;
private String ensRegionId;
private String networkId;
private String routeTableId;
private String routeTableName;
private String status;
private String type;
private java.util.List vSwitchIds;
/**
* The time when the route table was created. The time follows the ISO 8601 standard in the yyyy-MM-ddTHH:mm:ssZ format. The time is displayed in UTC.
*
* example:
* 2024-03-08T08:35:18Z
*/
public Builder creationTime(String creationTime) {
this.creationTime = creationTime;
return this;
}
/**
* The ID of the edge node.
*
* example:
* cn-beijing-15
*/
public Builder ensRegionId(String ensRegionId) {
this.ensRegionId = ensRegionId;
return this;
}
/**
* The ID of the network.
*
* example:
* n-5v9lufsezl4g****
*/
public Builder networkId(String networkId) {
this.networkId = networkId;
return this;
}
/**
* The ID of the route table.
*
* example:
* rt-5xde2bit9****
*/
public Builder routeTableId(String routeTableId) {
this.routeTableId = routeTableId;
return this;
}
/**
* RouteTableName.
*/
public Builder routeTableName(String routeTableName) {
this.routeTableName = routeTableName;
return this;
}
/**
* The status. Valid values:
*
* - Available: The route table is available.
*
*
* example:
* Available
*/
public Builder status(String status) {
this.status = status;
return this;
}
/**
* The type of the route table. Valid values:
*
* - Custom: custom route table.
* - System: system route table.
*
*
* example:
* System
*/
public Builder type(String type) {
this.type = type;
return this;
}
/**
* The vSwitches that are associated with the route table.
*/
public Builder vSwitchIds(java.util.List vSwitchIds) {
this.vSwitchIds = vSwitchIds;
return this;
}
public RouteTables build() {
return new RouteTables(this);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy