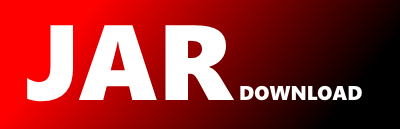
com.aliyun.sdk.service.ens20171110.models.DescribeNetworksResponseBody Maven / Gradle / Ivy
Show all versions of alibabacloud-ens20171110 Show documentation
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.sdk.service.ens20171110.models;
import com.aliyun.sdk.gateway.pop.*;
import darabonba.core.*;
import darabonba.core.async.*;
import darabonba.core.sync.*;
import darabonba.core.client.*;
import darabonba.core.RequestModel;
import darabonba.core.TeaModel;
import com.aliyun.sdk.gateway.pop.models.*;
/**
*
* {@link DescribeNetworksResponseBody} extends {@link TeaModel}
*
* DescribeNetworksResponseBody
*/
public class DescribeNetworksResponseBody extends TeaModel {
@com.aliyun.core.annotation.NameInMap("Networks")
private Networks networks;
@com.aliyun.core.annotation.NameInMap("PageNumber")
private Integer pageNumber;
@com.aliyun.core.annotation.NameInMap("PageSize")
private Integer pageSize;
@com.aliyun.core.annotation.NameInMap("RequestId")
private String requestId;
@com.aliyun.core.annotation.NameInMap("TotalCount")
private Integer totalCount;
private DescribeNetworksResponseBody(Builder builder) {
this.networks = builder.networks;
this.pageNumber = builder.pageNumber;
this.pageSize = builder.pageSize;
this.requestId = builder.requestId;
this.totalCount = builder.totalCount;
}
public static Builder builder() {
return new Builder();
}
public static DescribeNetworksResponseBody create() {
return builder().build();
}
/**
* @return networks
*/
public Networks getNetworks() {
return this.networks;
}
/**
* @return pageNumber
*/
public Integer getPageNumber() {
return this.pageNumber;
}
/**
* @return pageSize
*/
public Integer getPageSize() {
return this.pageSize;
}
/**
* @return requestId
*/
public String getRequestId() {
return this.requestId;
}
/**
* @return totalCount
*/
public Integer getTotalCount() {
return this.totalCount;
}
public static final class Builder {
private Networks networks;
private Integer pageNumber;
private Integer pageSize;
private String requestId;
private Integer totalCount;
/**
* The list of networks.
*/
public Builder networks(Networks networks) {
this.networks = networks;
return this;
}
/**
* The page number of the returned page.
*
* example:
* 1
*/
public Builder pageNumber(Integer pageNumber) {
this.pageNumber = pageNumber;
return this;
}
/**
* The number of entries returned on each page.
*
* example:
* 2
*/
public Builder pageSize(Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* The request ID.
*
* example:
* 473469C7-AA6F-4DC5-B3DB-A3DC0DE3C83E
*/
public Builder requestId(String requestId) {
this.requestId = requestId;
return this;
}
/**
* The total number of entries in the list.
*
* example:
* 3
*/
public Builder totalCount(Integer totalCount) {
this.totalCount = totalCount;
return this;
}
public DescribeNetworksResponseBody build() {
return new DescribeNetworksResponseBody(this);
}
}
/**
*
* {@link DescribeNetworksResponseBody} extends {@link TeaModel}
*
* DescribeNetworksResponseBody
*/
public static class VSwitchIds extends TeaModel {
@com.aliyun.core.annotation.NameInMap("VSwitchId")
private java.util.List vSwitchId;
private VSwitchIds(Builder builder) {
this.vSwitchId = builder.vSwitchId;
}
public static Builder builder() {
return new Builder();
}
public static VSwitchIds create() {
return builder().build();
}
/**
* @return vSwitchId
*/
public java.util.List getVSwitchId() {
return this.vSwitchId;
}
public static final class Builder {
private java.util.List vSwitchId;
/**
* VSwitchId.
*/
public Builder vSwitchId(java.util.List vSwitchId) {
this.vSwitchId = vSwitchId;
return this;
}
public VSwitchIds build() {
return new VSwitchIds(this);
}
}
}
/**
*
* {@link DescribeNetworksResponseBody} extends {@link TeaModel}
*
* DescribeNetworksResponseBody
*/
public static class Network extends TeaModel {
@com.aliyun.core.annotation.NameInMap("CidrBlock")
private String cidrBlock;
@com.aliyun.core.annotation.NameInMap("CreatedTime")
private String createdTime;
@com.aliyun.core.annotation.NameInMap("Description")
private String description;
@com.aliyun.core.annotation.NameInMap("EnsRegionId")
private String ensRegionId;
@com.aliyun.core.annotation.NameInMap("NetworkAclId")
private String networkAclId;
@com.aliyun.core.annotation.NameInMap("NetworkId")
private String networkId;
@com.aliyun.core.annotation.NameInMap("NetworkName")
private String networkName;
@com.aliyun.core.annotation.NameInMap("RouterTableId")
private String routerTableId;
@com.aliyun.core.annotation.NameInMap("Status")
private String status;
@com.aliyun.core.annotation.NameInMap("VSwitchIds")
private VSwitchIds vSwitchIds;
private Network(Builder builder) {
this.cidrBlock = builder.cidrBlock;
this.createdTime = builder.createdTime;
this.description = builder.description;
this.ensRegionId = builder.ensRegionId;
this.networkAclId = builder.networkAclId;
this.networkId = builder.networkId;
this.networkName = builder.networkName;
this.routerTableId = builder.routerTableId;
this.status = builder.status;
this.vSwitchIds = builder.vSwitchIds;
}
public static Builder builder() {
return new Builder();
}
public static Network create() {
return builder().build();
}
/**
* @return cidrBlock
*/
public String getCidrBlock() {
return this.cidrBlock;
}
/**
* @return createdTime
*/
public String getCreatedTime() {
return this.createdTime;
}
/**
* @return description
*/
public String getDescription() {
return this.description;
}
/**
* @return ensRegionId
*/
public String getEnsRegionId() {
return this.ensRegionId;
}
/**
* @return networkAclId
*/
public String getNetworkAclId() {
return this.networkAclId;
}
/**
* @return networkId
*/
public String getNetworkId() {
return this.networkId;
}
/**
* @return networkName
*/
public String getNetworkName() {
return this.networkName;
}
/**
* @return routerTableId
*/
public String getRouterTableId() {
return this.routerTableId;
}
/**
* @return status
*/
public String getStatus() {
return this.status;
}
/**
* @return vSwitchIds
*/
public VSwitchIds getVSwitchIds() {
return this.vSwitchIds;
}
public static final class Builder {
private String cidrBlock;
private String createdTime;
private String description;
private String ensRegionId;
private String networkAclId;
private String networkId;
private String networkName;
private String routerTableId;
private String status;
private VSwitchIds vSwitchIds;
/**
* The IPv4 CIDR block of the network.
*
* example:
* 10.0.xx.xx/24
*/
public Builder cidrBlock(String cidrBlock) {
this.cidrBlock = cidrBlock;
return this;
}
/**
* The timestamp when the instance was created. Unit: milliseconds.
*
* example:
* 2020-06-16T06:33:15Z
*/
public Builder createdTime(String createdTime) {
this.createdTime = createdTime;
return this;
}
/**
* The description of the network.
*
* example:
* exampleDescription
*/
public Builder description(String description) {
this.description = description;
return this;
}
/**
* The ID of the edge node.
*
* example:
* cn-beijing
*/
public Builder ensRegionId(String ensRegionId) {
this.ensRegionId = ensRegionId;
return this;
}
/**
* The ID of the network access control list (ACL).
*
* example:
* nacl-a2do9e413e0spxscd****
*/
public Builder networkAclId(String networkAclId) {
this.networkAclId = networkAclId;
return this;
}
/**
* The ID of the network.
*
* example:
* n-5***
*/
public Builder networkId(String networkId) {
this.networkId = networkId;
return this;
}
/**
* The name of the network.
*
* example:
* example
*/
public Builder networkName(String networkName) {
this.networkName = networkName;
return this;
}
/**
* The route table ID.
*
* example:
* rtb-5**
*/
public Builder routerTableId(String routerTableId) {
this.routerTableId = routerTableId;
return this;
}
/**
* The status of the network. Valid values:
*
* - Pending
* - Available
*
*
* example:
* Available
*/
public Builder status(String status) {
this.status = status;
return this;
}
/**
* The list of vSwitches in the network.
*/
public Builder vSwitchIds(VSwitchIds vSwitchIds) {
this.vSwitchIds = vSwitchIds;
return this;
}
public Network build() {
return new Network(this);
}
}
}
/**
*
* {@link DescribeNetworksResponseBody} extends {@link TeaModel}
*
* DescribeNetworksResponseBody
*/
public static class Networks extends TeaModel {
@com.aliyun.core.annotation.NameInMap("Network")
private java.util.List network;
private Networks(Builder builder) {
this.network = builder.network;
}
public static Builder builder() {
return new Builder();
}
public static Networks create() {
return builder().build();
}
/**
* @return network
*/
public java.util.List getNetwork() {
return this.network;
}
public static final class Builder {
private java.util.List network;
/**
* Network.
*/
public Builder network(java.util.List network) {
this.network = network;
return this;
}
public Networks build() {
return new Networks(this);
}
}
}
}