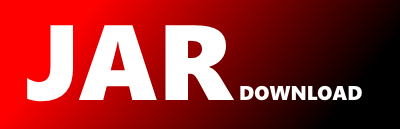
com.aliyun.sdk.service.ens20171110.models.ModifyInstanceAttributeRequest Maven / Gradle / Ivy
Show all versions of alibabacloud-ens20171110 Show documentation
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.sdk.service.ens20171110.models;
import com.aliyun.sdk.gateway.pop.*;
import darabonba.core.*;
import darabonba.core.async.*;
import darabonba.core.sync.*;
import darabonba.core.client.*;
import darabonba.core.RequestModel;
import darabonba.core.TeaModel;
import com.aliyun.sdk.gateway.pop.models.*;
/**
*
* {@link ModifyInstanceAttributeRequest} extends {@link RequestModel}
*
* ModifyInstanceAttributeRequest
*/
public class ModifyInstanceAttributeRequest extends Request {
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("HostName")
private String hostName;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("InstanceId")
@com.aliyun.core.annotation.Validation(required = true)
private String instanceId;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("InstanceName")
private String instanceName;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("Password")
private String password;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("UserData")
private String userData;
private ModifyInstanceAttributeRequest(Builder builder) {
super(builder);
this.hostName = builder.hostName;
this.instanceId = builder.instanceId;
this.instanceName = builder.instanceName;
this.password = builder.password;
this.userData = builder.userData;
}
public static Builder builder() {
return new Builder();
}
public static ModifyInstanceAttributeRequest create() {
return builder().build();
}
@Override
public Builder toBuilder() {
return new Builder(this);
}
/**
* @return hostName
*/
public String getHostName() {
return this.hostName;
}
/**
* @return instanceId
*/
public String getInstanceId() {
return this.instanceId;
}
/**
* @return instanceName
*/
public String getInstanceName() {
return this.instanceName;
}
/**
* @return password
*/
public String getPassword() {
return this.password;
}
/**
* @return userData
*/
public String getUserData() {
return this.userData;
}
public static final class Builder extends Request.Builder {
private String hostName;
private String instanceId;
private String instanceName;
private String password;
private String userData;
private Builder() {
super();
}
private Builder(ModifyInstanceAttributeRequest request) {
super(request);
this.hostName = request.hostName;
this.instanceId = request.instanceId;
this.instanceName = request.instanceName;
this.password = request.password;
this.userData = request.userData;
}
/**
* The hostname of the Elastic Compute Service (ECS) instance. The value can be 2 to 64 characters in length. You can use periods (.) to separate the value into multiple segments. Each segment can contain letters, digits, hyphens (-), and periods. Consecutive periods or hyphens are not allowed. The name cannot start or end with a period (.) or a hyphen (-).
*
* example:
* testHostName
*/
public Builder hostName(String hostName) {
this.putQueryParameter("HostName", hostName);
this.hostName = hostName;
return this;
}
/**
* The ID of the instance for which you want to modify attributes. You can specify only one ID.
* This parameter is required.
*
* example:
* i-instanc****
*/
public Builder instanceId(String instanceId) {
this.putQueryParameter("InstanceId", instanceId);
this.instanceId = instanceId;
return this;
}
/**
* The name of the instance.
* The name must be 2 to 128 characters in length. It must start with a letter but cannot start with http://
or https://
. The name can contain letters, digits, colons (:), underscores (_), and hyphens (-).
*
* example:
* i-instanceidname
*/
public Builder instanceName(String instanceName) {
this.putQueryParameter("InstanceName", instanceName);
this.instanceName = instanceName;
return this;
}
/**
* The password of the instance.
*
* example:
* yourPassword
*/
public Builder password(String password) {
this.putQueryParameter("Password", password);
this.password = password;
return this;
}
/**
* The user data of the instance. User data must be encoded in Base64.
* The size of your UserData cannot exceed 16 KB. We recommend that you do not pass in confidential information such as passwords and private keys in the plaintext format. If you must pass in confidential information, we recommend that you encrypt and Base64-encode the information before you pass it in. Then you can decode and decrypt the information in the same way within the instance.
*
* example:
* /9j/4AAQSkZJRgABAQAAAQABAAD/2wBDAAgGBgcGBQgHBwcJCQgK****
*/
public Builder userData(String userData) {
this.putQueryParameter("UserData", userData);
this.userData = userData;
return this;
}
@Override
public ModifyInstanceAttributeRequest build() {
return new ModifyInstanceAttributeRequest(this);
}
}
}