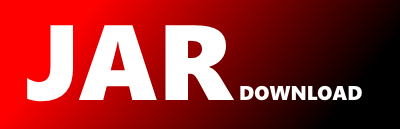
com.aliyun.sdk.service.polardb20170801.models.CreateStoragePlanRequest Maven / Gradle / Ivy
Show all versions of alibabacloud-polardb20170801 Show documentation
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.sdk.service.polardb20170801.models;
import com.aliyun.sdk.gateway.pop.*;
import darabonba.core.*;
import darabonba.core.async.*;
import darabonba.core.sync.*;
import darabonba.core.client.*;
import darabonba.core.RequestModel;
import darabonba.core.TeaModel;
import com.aliyun.sdk.gateway.pop.models.*;
/**
*
* {@link CreateStoragePlanRequest} extends {@link RequestModel}
*
* CreateStoragePlanRequest
*/
public class CreateStoragePlanRequest extends Request {
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("ClientToken")
private String clientToken;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("OwnerAccount")
private String ownerAccount;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("OwnerId")
private Long ownerId;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("Period")
@com.aliyun.core.annotation.Validation(required = true)
private String period;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("ResourceOwnerAccount")
private String resourceOwnerAccount;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("ResourceOwnerId")
private Long resourceOwnerId;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("StorageClass")
@com.aliyun.core.annotation.Validation(required = true)
private String storageClass;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("StorageType")
@com.aliyun.core.annotation.Validation(required = true)
private String storageType;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("UsedTime")
@com.aliyun.core.annotation.Validation(required = true)
private String usedTime;
private CreateStoragePlanRequest(Builder builder) {
super(builder);
this.clientToken = builder.clientToken;
this.ownerAccount = builder.ownerAccount;
this.ownerId = builder.ownerId;
this.period = builder.period;
this.resourceOwnerAccount = builder.resourceOwnerAccount;
this.resourceOwnerId = builder.resourceOwnerId;
this.storageClass = builder.storageClass;
this.storageType = builder.storageType;
this.usedTime = builder.usedTime;
}
public static Builder builder() {
return new Builder();
}
public static CreateStoragePlanRequest create() {
return builder().build();
}
@Override
public Builder toBuilder() {
return new Builder(this);
}
/**
* @return clientToken
*/
public String getClientToken() {
return this.clientToken;
}
/**
* @return ownerAccount
*/
public String getOwnerAccount() {
return this.ownerAccount;
}
/**
* @return ownerId
*/
public Long getOwnerId() {
return this.ownerId;
}
/**
* @return period
*/
public String getPeriod() {
return this.period;
}
/**
* @return resourceOwnerAccount
*/
public String getResourceOwnerAccount() {
return this.resourceOwnerAccount;
}
/**
* @return resourceOwnerId
*/
public Long getResourceOwnerId() {
return this.resourceOwnerId;
}
/**
* @return storageClass
*/
public String getStorageClass() {
return this.storageClass;
}
/**
* @return storageType
*/
public String getStorageType() {
return this.storageType;
}
/**
* @return usedTime
*/
public String getUsedTime() {
return this.usedTime;
}
public static final class Builder extends Request.Builder {
private String clientToken;
private String ownerAccount;
private Long ownerId;
private String period;
private String resourceOwnerAccount;
private Long resourceOwnerId;
private String storageClass;
private String storageType;
private String usedTime;
private Builder() {
super();
}
private Builder(CreateStoragePlanRequest request) {
super(request);
this.clientToken = request.clientToken;
this.ownerAccount = request.ownerAccount;
this.ownerId = request.ownerId;
this.period = request.period;
this.resourceOwnerAccount = request.resourceOwnerAccount;
this.resourceOwnerId = request.resourceOwnerId;
this.storageClass = request.storageClass;
this.storageType = request.storageType;
this.usedTime = request.usedTime;
}
/**
* The client token that is used to ensure the idempotence of the request. You can use the client to generate the value. Make sure that the value is unique among different requests. The token can only contain ASCII characters and cannot exceed 64 characters in length.
*
* example:
* 6000170000591aed949d0f5********************
*/
public Builder clientToken(String clientToken) {
this.putQueryParameter("ClientToken", clientToken);
this.clientToken = clientToken;
return this;
}
/**
* OwnerAccount.
*/
public Builder ownerAccount(String ownerAccount) {
this.putQueryParameter("OwnerAccount", ownerAccount);
this.ownerAccount = ownerAccount;
return this;
}
/**
* OwnerId.
*/
public Builder ownerId(Long ownerId) {
this.putQueryParameter("OwnerId", ownerId);
this.ownerId = ownerId;
return this;
}
/**
* The unit of the subscription duration for the storage plan. Valid values:
*
* - Month
* - Year
*
* This parameter is required.
*
* example:
* Month
*/
public Builder period(String period) {
this.putQueryParameter("Period", period);
this.period = period;
return this;
}
/**
* ResourceOwnerAccount.
*/
public Builder resourceOwnerAccount(String resourceOwnerAccount) {
this.putQueryParameter("ResourceOwnerAccount", resourceOwnerAccount);
this.resourceOwnerAccount = resourceOwnerAccount;
return this;
}
/**
* ResourceOwnerId.
*/
public Builder resourceOwnerId(Long resourceOwnerId) {
this.putQueryParameter("ResourceOwnerId", resourceOwnerId);
this.resourceOwnerId = resourceOwnerId;
return this;
}
/**
* The capacity of the storage plan. Unit: GB. Valid values: 50, 100, 200, 300, 500, 1000, 2000, 3000, 5000, 10000, 15000, 20000, 25000, 30000, 50000, 100000, and 200000
* This parameter is required.
*
* example:
* 500
*/
public Builder storageClass(String storageClass) {
this.putQueryParameter("StorageClass", storageClass);
this.storageClass = storageClass;
return this;
}
/**
* The type of the storage plan. Valid values:
*
* - Mainland: The storage plan is used inside the Chinese mainland.
* - Overseas: The storage plan is used outside the Chinese mainland.
*
* This parameter is required.
*
* example:
* Mainland
*/
public Builder storageType(String storageType) {
this.putQueryParameter("StorageType", storageType);
this.storageType = storageType;
return this;
}
/**
* The subscription duration of the storage plan.
*
* - If Period is set to Month, the value ranges from 1 to 9.
* - If Period is set to Year, the value can be 1, 2, 3, or 5.
*
* This parameter is required.
*
* example:
* 3
*/
public Builder usedTime(String usedTime) {
this.putQueryParameter("UsedTime", usedTime);
this.usedTime = usedTime;
return this;
}
@Override
public CreateStoragePlanRequest build() {
return new CreateStoragePlanRequest(this);
}
}
}