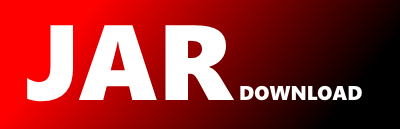
com.aliyun.sdk.service.polardb20170801.models.DescribeActivationCodesResponseBody Maven / Gradle / Ivy
Show all versions of alibabacloud-polardb20170801 Show documentation
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.sdk.service.polardb20170801.models;
import com.aliyun.sdk.gateway.pop.*;
import darabonba.core.*;
import darabonba.core.async.*;
import darabonba.core.sync.*;
import darabonba.core.client.*;
import darabonba.core.RequestModel;
import darabonba.core.TeaModel;
import com.aliyun.sdk.gateway.pop.models.*;
/**
*
* {@link DescribeActivationCodesResponseBody} extends {@link TeaModel}
*
* DescribeActivationCodesResponseBody
*/
public class DescribeActivationCodesResponseBody extends TeaModel {
@com.aliyun.core.annotation.NameInMap("Items")
private java.util.List items;
@com.aliyun.core.annotation.NameInMap("PageNumber")
private Integer pageNumber;
@com.aliyun.core.annotation.NameInMap("PageRecordCount")
private Integer pageRecordCount;
@com.aliyun.core.annotation.NameInMap("RequestId")
private String requestId;
@com.aliyun.core.annotation.NameInMap("TotalRecordCount")
private Integer totalRecordCount;
private DescribeActivationCodesResponseBody(Builder builder) {
this.items = builder.items;
this.pageNumber = builder.pageNumber;
this.pageRecordCount = builder.pageRecordCount;
this.requestId = builder.requestId;
this.totalRecordCount = builder.totalRecordCount;
}
public static Builder builder() {
return new Builder();
}
public static DescribeActivationCodesResponseBody create() {
return builder().build();
}
/**
* @return items
*/
public java.util.List getItems() {
return this.items;
}
/**
* @return pageNumber
*/
public Integer getPageNumber() {
return this.pageNumber;
}
/**
* @return pageRecordCount
*/
public Integer getPageRecordCount() {
return this.pageRecordCount;
}
/**
* @return requestId
*/
public String getRequestId() {
return this.requestId;
}
/**
* @return totalRecordCount
*/
public Integer getTotalRecordCount() {
return this.totalRecordCount;
}
public static final class Builder {
private java.util.List items;
private Integer pageNumber;
private Integer pageRecordCount;
private String requestId;
private Integer totalRecordCount;
/**
* The queried activation codes.
*/
public Builder items(java.util.List items) {
this.items = items;
return this;
}
/**
* The page number.
*
* example:
* 1
*/
public Builder pageNumber(Integer pageNumber) {
this.pageNumber = pageNumber;
return this;
}
/**
* The number of entries returned on the current page.
*
* example:
* 1
*/
public Builder pageRecordCount(Integer pageRecordCount) {
this.pageRecordCount = pageRecordCount;
return this;
}
/**
* The request ID.
*
* example:
* 65D7ACE6-4A61-4B6E-B357-8CB24A******
*/
public Builder requestId(String requestId) {
this.requestId = requestId;
return this;
}
/**
* The total number of entries returned.
*
* example:
* 1
*/
public Builder totalRecordCount(Integer totalRecordCount) {
this.totalRecordCount = totalRecordCount;
return this;
}
public DescribeActivationCodesResponseBody build() {
return new DescribeActivationCodesResponseBody(this);
}
}
/**
*
* {@link DescribeActivationCodesResponseBody} extends {@link TeaModel}
*
* DescribeActivationCodesResponseBody
*/
public static class Items extends TeaModel {
@com.aliyun.core.annotation.NameInMap("ActivateAt")
private String activateAt;
@com.aliyun.core.annotation.NameInMap("Description")
private String description;
@com.aliyun.core.annotation.NameInMap("ExpireAt")
private String expireAt;
@com.aliyun.core.annotation.NameInMap("GmtCreated")
private String gmtCreated;
@com.aliyun.core.annotation.NameInMap("GmtModified")
private String gmtModified;
@com.aliyun.core.annotation.NameInMap("Id")
private Integer id;
@com.aliyun.core.annotation.NameInMap("MacAddress")
private String macAddress;
@com.aliyun.core.annotation.NameInMap("Name")
private String name;
@com.aliyun.core.annotation.NameInMap("SystemIdentifier")
private String systemIdentifier;
private Items(Builder builder) {
this.activateAt = builder.activateAt;
this.description = builder.description;
this.expireAt = builder.expireAt;
this.gmtCreated = builder.gmtCreated;
this.gmtModified = builder.gmtModified;
this.id = builder.id;
this.macAddress = builder.macAddress;
this.name = builder.name;
this.systemIdentifier = builder.systemIdentifier;
}
public static Builder builder() {
return new Builder();
}
public static Items create() {
return builder().build();
}
/**
* @return activateAt
*/
public String getActivateAt() {
return this.activateAt;
}
/**
* @return description
*/
public String getDescription() {
return this.description;
}
/**
* @return expireAt
*/
public String getExpireAt() {
return this.expireAt;
}
/**
* @return gmtCreated
*/
public String getGmtCreated() {
return this.gmtCreated;
}
/**
* @return gmtModified
*/
public String getGmtModified() {
return this.gmtModified;
}
/**
* @return id
*/
public Integer getId() {
return this.id;
}
/**
* @return macAddress
*/
public String getMacAddress() {
return this.macAddress;
}
/**
* @return name
*/
public String getName() {
return this.name;
}
/**
* @return systemIdentifier
*/
public String getSystemIdentifier() {
return this.systemIdentifier;
}
public static final class Builder {
private String activateAt;
private String description;
private String expireAt;
private String gmtCreated;
private String gmtModified;
private Integer id;
private String macAddress;
private String name;
private String systemIdentifier;
/**
* The time when the activation code takes effect.
*
* example:
* 2024-10-16 16:46:20
*/
public Builder activateAt(String activateAt) {
this.activateAt = activateAt;
return this;
}
/**
* The description of the activation code.
*
* example:
* testCode
*/
public Builder description(String description) {
this.description = description;
return this;
}
/**
* The time when the activation code expires.
*
* example:
* 2054-10-16 16:46:20
*/
public Builder expireAt(String expireAt) {
this.expireAt = expireAt;
return this;
}
/**
* The time when the activation code was generated.
*
* example:
* 2024-10-16 16:46:20
*/
public Builder gmtCreated(String gmtCreated) {
this.gmtCreated = gmtCreated;
return this;
}
/**
* The time when the activation code was updated.
*
* example:
* 2024-10-16 16:46:20
*/
public Builder gmtModified(String gmtModified) {
this.gmtModified = gmtModified;
return this;
}
/**
* The activation code ID.
*
* example:
* 123
*/
public Builder id(Integer id) {
this.id = id;
return this;
}
/**
* The media access control (MAC) address used in the generation of the activation code.
*
* example:
* 12:34:56:78:98:00
*/
public Builder macAddress(String macAddress) {
this.macAddress = macAddress;
return this;
}
/**
* The name of the activation code.
*
* example:
* testName
*/
public Builder name(String name) {
this.name = name;
return this;
}
/**
* The unique identifier of the database.
*
* example:
* 1234567890123456
*/
public Builder systemIdentifier(String systemIdentifier) {
this.systemIdentifier = systemIdentifier;
return this;
}
public Items build() {
return new Items(this);
}
}
}
}