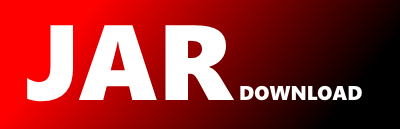
com.aliyun.sdk.service.polardb20170801.models.DescribeScheduleTasksResponseBody Maven / Gradle / Ivy
Show all versions of alibabacloud-polardb20170801 Show documentation
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.sdk.service.polardb20170801.models;
import com.aliyun.sdk.gateway.pop.*;
import darabonba.core.*;
import darabonba.core.async.*;
import darabonba.core.sync.*;
import darabonba.core.client.*;
import darabonba.core.RequestModel;
import darabonba.core.TeaModel;
import com.aliyun.sdk.gateway.pop.models.*;
/**
*
* {@link DescribeScheduleTasksResponseBody} extends {@link TeaModel}
*
* DescribeScheduleTasksResponseBody
*/
public class DescribeScheduleTasksResponseBody extends TeaModel {
@com.aliyun.core.annotation.NameInMap("Data")
private Data data;
@com.aliyun.core.annotation.NameInMap("Message")
private String message;
@com.aliyun.core.annotation.NameInMap("RequestId")
private String requestId;
@com.aliyun.core.annotation.NameInMap("Success")
private Boolean success;
private DescribeScheduleTasksResponseBody(Builder builder) {
this.data = builder.data;
this.message = builder.message;
this.requestId = builder.requestId;
this.success = builder.success;
}
public static Builder builder() {
return new Builder();
}
public static DescribeScheduleTasksResponseBody create() {
return builder().build();
}
/**
* @return data
*/
public Data getData() {
return this.data;
}
/**
* @return message
*/
public String getMessage() {
return this.message;
}
/**
* @return requestId
*/
public String getRequestId() {
return this.requestId;
}
/**
* @return success
*/
public Boolean getSuccess() {
return this.success;
}
public static final class Builder {
private Data data;
private String message;
private String requestId;
private Boolean success;
/**
* The result data.
*/
public Builder data(Data data) {
this.data = data;
return this;
}
/**
* The message that is returned for the request.
*
* If the request is successful, Successful is returned. If the request fails, an error message such as an error code is returned.
*
*
* example:
* Successful
*/
public Builder message(String message) {
this.message = message;
return this;
}
/**
* The request ID.
*
* example:
* 936C7025-27A5-4CB1-BB31-540E1F0CCA12
*/
public Builder requestId(String requestId) {
this.requestId = requestId;
return this;
}
/**
* Indicates whether the request is successful.
*
* example:
* true
*/
public Builder success(Boolean success) {
this.success = success;
return this;
}
public DescribeScheduleTasksResponseBody build() {
return new DescribeScheduleTasksResponseBody(this);
}
}
/**
*
* {@link DescribeScheduleTasksResponseBody} extends {@link TeaModel}
*
* DescribeScheduleTasksResponseBody
*/
public static class TimerInfos extends TeaModel {
@com.aliyun.core.annotation.NameInMap("Action")
private String action;
@com.aliyun.core.annotation.NameInMap("CrontabJobId")
private String crontabJobId;
@com.aliyun.core.annotation.NameInMap("DBClusterId")
private String DBClusterId;
@com.aliyun.core.annotation.NameInMap("DbClusterDescription")
private String dbClusterDescription;
@com.aliyun.core.annotation.NameInMap("DbClusterStatus")
private String dbClusterStatus;
@com.aliyun.core.annotation.NameInMap("OrderId")
private String orderId;
@com.aliyun.core.annotation.NameInMap("PlannedEndTime")
private String plannedEndTime;
@com.aliyun.core.annotation.NameInMap("PlannedFlashingOffTime")
private String plannedFlashingOffTime;
@com.aliyun.core.annotation.NameInMap("PlannedStartTime")
private String plannedStartTime;
@com.aliyun.core.annotation.NameInMap("PlannedTime")
private String plannedTime;
@com.aliyun.core.annotation.NameInMap("Region")
private String region;
@com.aliyun.core.annotation.NameInMap("Status")
private String status;
@com.aliyun.core.annotation.NameInMap("TaskCancel")
private Boolean taskCancel;
@com.aliyun.core.annotation.NameInMap("TaskId")
private String taskId;
private TimerInfos(Builder builder) {
this.action = builder.action;
this.crontabJobId = builder.crontabJobId;
this.DBClusterId = builder.DBClusterId;
this.dbClusterDescription = builder.dbClusterDescription;
this.dbClusterStatus = builder.dbClusterStatus;
this.orderId = builder.orderId;
this.plannedEndTime = builder.plannedEndTime;
this.plannedFlashingOffTime = builder.plannedFlashingOffTime;
this.plannedStartTime = builder.plannedStartTime;
this.plannedTime = builder.plannedTime;
this.region = builder.region;
this.status = builder.status;
this.taskCancel = builder.taskCancel;
this.taskId = builder.taskId;
}
public static Builder builder() {
return new Builder();
}
public static TimerInfos create() {
return builder().build();
}
/**
* @return action
*/
public String getAction() {
return this.action;
}
/**
* @return crontabJobId
*/
public String getCrontabJobId() {
return this.crontabJobId;
}
/**
* @return DBClusterId
*/
public String getDBClusterId() {
return this.DBClusterId;
}
/**
* @return dbClusterDescription
*/
public String getDbClusterDescription() {
return this.dbClusterDescription;
}
/**
* @return dbClusterStatus
*/
public String getDbClusterStatus() {
return this.dbClusterStatus;
}
/**
* @return orderId
*/
public String getOrderId() {
return this.orderId;
}
/**
* @return plannedEndTime
*/
public String getPlannedEndTime() {
return this.plannedEndTime;
}
/**
* @return plannedFlashingOffTime
*/
public String getPlannedFlashingOffTime() {
return this.plannedFlashingOffTime;
}
/**
* @return plannedStartTime
*/
public String getPlannedStartTime() {
return this.plannedStartTime;
}
/**
* @return plannedTime
*/
public String getPlannedTime() {
return this.plannedTime;
}
/**
* @return region
*/
public String getRegion() {
return this.region;
}
/**
* @return status
*/
public String getStatus() {
return this.status;
}
/**
* @return taskCancel
*/
public Boolean getTaskCancel() {
return this.taskCancel;
}
/**
* @return taskId
*/
public String getTaskId() {
return this.taskId;
}
public static final class Builder {
private String action;
private String crontabJobId;
private String DBClusterId;
private String dbClusterDescription;
private String dbClusterStatus;
private String orderId;
private String plannedEndTime;
private String plannedFlashingOffTime;
private String plannedStartTime;
private String plannedTime;
private String region;
private String status;
private Boolean taskCancel;
private String taskId;
/**
* The type of the scheduled tasks.
*
* example:
* CreateDBNodes
*/
public Builder action(String action) {
this.action = action;
return this;
}
/**
* The ID of the scheduled task.
*
* example:
* 86293c29-a03d-4872-b625-***********
*/
public Builder crontabJobId(String crontabJobId) {
this.crontabJobId = crontabJobId;
return this;
}
/**
* The cluster ID.
*
* example:
* pc-**************
*/
public Builder DBClusterId(String DBClusterId) {
this.DBClusterId = DBClusterId;
return this;
}
/**
* The description of the cluster.
*
* example:
* test_cluster
*/
public Builder dbClusterDescription(String dbClusterDescription) {
this.dbClusterDescription = dbClusterDescription;
return this;
}
/**
* The state of the cluster.
*
* example:
* Running
*/
public Builder dbClusterStatus(String dbClusterStatus) {
this.dbClusterStatus = dbClusterStatus;
return this;
}
/**
* The ID of the order.
*
* This parameter is returned only when you set the Action
parameter to CreateDBNodes or ModifyDBNodeClass.
*
*
* example:
* 208161753******
*/
public Builder orderId(String orderId) {
this.orderId = orderId;
return this;
}
/**
* The latest start time of the task that you specified when you created the scheduled task. The time is displayed in UTC.
*
* example:
* 2021-01-28T12:30Z
*/
public Builder plannedEndTime(String plannedEndTime) {
this.plannedEndTime = plannedEndTime;
return this;
}
/**
* PlannedFlashingOffTime.
*/
public Builder plannedFlashingOffTime(String plannedFlashingOffTime) {
this.plannedFlashingOffTime = plannedFlashingOffTime;
return this;
}
/**
* The earliest start time of the task that you specified when you created the scheduled task. The time is displayed in UTC.
*
* example:
* 2021-01-28T12:00Z
*/
public Builder plannedStartTime(String plannedStartTime) {
this.plannedStartTime = plannedStartTime;
return this;
}
/**
* The expected start time of the task. The time is displayed in UTC.
*
* example:
* 2021-01-28T12:16Z
*/
public Builder plannedTime(String plannedTime) {
this.plannedTime = plannedTime;
return this;
}
/**
* The ID of the region in which the scheduled task runs.
*
* example:
* cn-hangzhou
*/
public Builder region(String region) {
this.region = region;
return this;
}
/**
* The state of the scheduled task.
*
* example:
* finish
*/
public Builder status(String status) {
this.status = status;
return this;
}
/**
* Indicates whether the scheduled task can be canceled. Valid values:
*
* - true
* - false
*
*
* example:
* true
*/
public Builder taskCancel(Boolean taskCancel) {
this.taskCancel = taskCancel;
return this;
}
/**
* The ID of the task.
*
* example:
* 53879cdb-9a00-428e-acaf-ff4cff******
*/
public Builder taskId(String taskId) {
this.taskId = taskId;
return this;
}
public TimerInfos build() {
return new TimerInfos(this);
}
}
}
/**
*
* {@link DescribeScheduleTasksResponseBody} extends {@link TeaModel}
*
* DescribeScheduleTasksResponseBody
*/
public static class Data extends TeaModel {
@com.aliyun.core.annotation.NameInMap("PageNumber")
private Integer pageNumber;
@com.aliyun.core.annotation.NameInMap("PageSize")
private Integer pageSize;
@com.aliyun.core.annotation.NameInMap("TimerInfos")
private java.util.List timerInfos;
@com.aliyun.core.annotation.NameInMap("TotalRecordCount")
private Integer totalRecordCount;
private Data(Builder builder) {
this.pageNumber = builder.pageNumber;
this.pageSize = builder.pageSize;
this.timerInfos = builder.timerInfos;
this.totalRecordCount = builder.totalRecordCount;
}
public static Builder builder() {
return new Builder();
}
public static Data create() {
return builder().build();
}
/**
* @return pageNumber
*/
public Integer getPageNumber() {
return this.pageNumber;
}
/**
* @return pageSize
*/
public Integer getPageSize() {
return this.pageSize;
}
/**
* @return timerInfos
*/
public java.util.List getTimerInfos() {
return this.timerInfos;
}
/**
* @return totalRecordCount
*/
public Integer getTotalRecordCount() {
return this.totalRecordCount;
}
public static final class Builder {
private Integer pageNumber;
private Integer pageSize;
private java.util.List timerInfos;
private Integer totalRecordCount;
/**
* The page number of the page returned.
*
* example:
* 1
*/
public Builder pageNumber(Integer pageNumber) {
this.pageNumber = pageNumber;
return this;
}
/**
* The number of entries returned per page.
*
* example:
* 30
*/
public Builder pageSize(Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* The details of the scheduled tasks.
*/
public Builder timerInfos(java.util.List timerInfos) {
this.timerInfos = timerInfos;
return this;
}
/**
* The total number of entries returned.
*
* example:
* 1
*/
public Builder totalRecordCount(Integer totalRecordCount) {
this.totalRecordCount = totalRecordCount;
return this;
}
public Data build() {
return new Data(this);
}
}
}
}