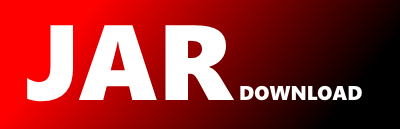
com.aliyun.sdk.service.polardb20170801.models.ModifyActiveOperationTasksRequest Maven / Gradle / Ivy
Show all versions of alibabacloud-polardb20170801 Show documentation
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.sdk.service.polardb20170801.models;
import com.aliyun.sdk.gateway.pop.*;
import darabonba.core.*;
import darabonba.core.async.*;
import darabonba.core.sync.*;
import darabonba.core.client.*;
import darabonba.core.RequestModel;
import darabonba.core.TeaModel;
import com.aliyun.sdk.gateway.pop.models.*;
/**
*
* {@link ModifyActiveOperationTasksRequest} extends {@link RequestModel}
*
* ModifyActiveOperationTasksRequest
*/
public class ModifyActiveOperationTasksRequest extends Request {
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("ImmediateStart")
private Integer immediateStart;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("OwnerAccount")
private String ownerAccount;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("OwnerId")
private Long ownerId;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("RegionId")
@com.aliyun.core.annotation.Validation(required = true)
private String regionId;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("ResourceOwnerAccount")
private String resourceOwnerAccount;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("ResourceOwnerId")
private Long resourceOwnerId;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("SecurityToken")
private String securityToken;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("SwitchTime")
private String switchTime;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("TaskIds")
@com.aliyun.core.annotation.Validation(required = true)
private String taskIds;
private ModifyActiveOperationTasksRequest(Builder builder) {
super(builder);
this.immediateStart = builder.immediateStart;
this.ownerAccount = builder.ownerAccount;
this.ownerId = builder.ownerId;
this.regionId = builder.regionId;
this.resourceOwnerAccount = builder.resourceOwnerAccount;
this.resourceOwnerId = builder.resourceOwnerId;
this.securityToken = builder.securityToken;
this.switchTime = builder.switchTime;
this.taskIds = builder.taskIds;
}
public static Builder builder() {
return new Builder();
}
public static ModifyActiveOperationTasksRequest create() {
return builder().build();
}
@Override
public Builder toBuilder() {
return new Builder(this);
}
/**
* @return immediateStart
*/
public Integer getImmediateStart() {
return this.immediateStart;
}
/**
* @return ownerAccount
*/
public String getOwnerAccount() {
return this.ownerAccount;
}
/**
* @return ownerId
*/
public Long getOwnerId() {
return this.ownerId;
}
/**
* @return regionId
*/
public String getRegionId() {
return this.regionId;
}
/**
* @return resourceOwnerAccount
*/
public String getResourceOwnerAccount() {
return this.resourceOwnerAccount;
}
/**
* @return resourceOwnerId
*/
public Long getResourceOwnerId() {
return this.resourceOwnerId;
}
/**
* @return securityToken
*/
public String getSecurityToken() {
return this.securityToken;
}
/**
* @return switchTime
*/
public String getSwitchTime() {
return this.switchTime;
}
/**
* @return taskIds
*/
public String getTaskIds() {
return this.taskIds;
}
public static final class Builder extends Request.Builder {
private Integer immediateStart;
private String ownerAccount;
private Long ownerId;
private String regionId;
private String resourceOwnerAccount;
private Long resourceOwnerId;
private String securityToken;
private String switchTime;
private String taskIds;
private Builder() {
super();
}
private Builder(ModifyActiveOperationTasksRequest request) {
super(request);
this.immediateStart = request.immediateStart;
this.ownerAccount = request.ownerAccount;
this.ownerId = request.ownerId;
this.regionId = request.regionId;
this.resourceOwnerAccount = request.resourceOwnerAccount;
this.resourceOwnerId = request.resourceOwnerId;
this.securityToken = request.securityToken;
this.switchTime = request.switchTime;
this.taskIds = request.taskIds;
}
/**
* Specifies whether to immediately start scheduling. Valid values:
*
* - 0: No. This is the default value.
* - 1: Yes.
*
*
*
*
* If you set this parameter to 0, you must specify the SwitchTime parameter.
*
* If you set this parameter to 1, the SwitchTime parameter does not take effect. In this case, the start time of the event is set to the current time, and the system determines the switching time based on the start time. Scheduling is started immediately, which is a prerequisite for the switchover. Then, the switchover is performed. You can call the DescribeActiveOperationTasks operation and check the return value of the PrepareInterval parameter for the preparation time.
*
*
*
* example:
* 0
*/
public Builder immediateStart(Integer immediateStart) {
this.putQueryParameter("ImmediateStart", immediateStart);
this.immediateStart = immediateStart;
return this;
}
/**
* OwnerAccount.
*/
public Builder ownerAccount(String ownerAccount) {
this.putQueryParameter("OwnerAccount", ownerAccount);
this.ownerAccount = ownerAccount;
return this;
}
/**
* OwnerId.
*/
public Builder ownerId(Long ownerId) {
this.putQueryParameter("OwnerId", ownerId);
this.ownerId = ownerId;
return this;
}
/**
* The region ID.
*
* You can call the DescribeRegions operation to query the region information about all clusters within a specified account.
*
* This parameter is required.
*
* example:
* cn-beijing
*/
public Builder regionId(String regionId) {
this.putQueryParameter("RegionId", regionId);
this.regionId = regionId;
return this;
}
/**
* ResourceOwnerAccount.
*/
public Builder resourceOwnerAccount(String resourceOwnerAccount) {
this.putQueryParameter("ResourceOwnerAccount", resourceOwnerAccount);
this.resourceOwnerAccount = resourceOwnerAccount;
return this;
}
/**
* ResourceOwnerId.
*/
public Builder resourceOwnerId(Long resourceOwnerId) {
this.putQueryParameter("ResourceOwnerId", resourceOwnerId);
this.resourceOwnerId = resourceOwnerId;
return this;
}
/**
* SecurityToken.
*/
public Builder securityToken(String securityToken) {
this.putQueryParameter("SecurityToken", securityToken);
this.securityToken = securityToken;
return this;
}
/**
* The scheduled switching time that you want to specify. Specify the time in the ISO 8601 standard in the yyyy-MM-ddTHH:mm:ssZ format. The time must be in UTC.
*
*
*
* The time that is specified by this parameter cannot be later than the latest execution time.
*
* You can call the DescribeActiveOperationTasks operation and check the return value of the Deadline parameter for the latest execution time.
*
*
*
* example:
* 2023-04-25T06:00:00Z
*/
public Builder switchTime(String switchTime) {
this.putQueryParameter("SwitchTime", switchTime);
this.switchTime = switchTime;
return this;
}
/**
* The task IDs.
* This parameter is required.
*
* example:
* 11111,22222
*/
public Builder taskIds(String taskIds) {
this.putQueryParameter("TaskIds", taskIds);
this.taskIds = taskIds;
return this;
}
@Override
public ModifyActiveOperationTasksRequest build() {
return new ModifyActiveOperationTasksRequest(this);
}
}
}