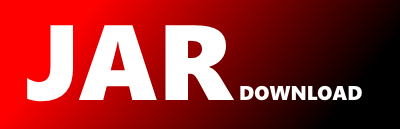
com.aliyun.sdk.service.polardb20170801.models.ModifyDBClusterRequest Maven / Gradle / Ivy
Show all versions of alibabacloud-polardb20170801 Show documentation
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.sdk.service.polardb20170801.models;
import com.aliyun.sdk.gateway.pop.*;
import darabonba.core.*;
import darabonba.core.async.*;
import darabonba.core.sync.*;
import darabonba.core.client.*;
import darabonba.core.RequestModel;
import darabonba.core.TeaModel;
import com.aliyun.sdk.gateway.pop.models.*;
/**
*
* {@link ModifyDBClusterRequest} extends {@link RequestModel}
*
* ModifyDBClusterRequest
*/
public class ModifyDBClusterRequest extends Request {
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("CompressStorage")
private String compressStorage;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("DBClusterId")
@com.aliyun.core.annotation.Validation(required = true)
private String DBClusterId;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("DBNodeCrashList")
private String DBNodeCrashList;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("DataSyncMode")
private String dataSyncMode;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("FaultInjectionType")
private String faultInjectionType;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("FaultSimulateMode")
private String faultSimulateMode;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("ImciAutoIndex")
private String imciAutoIndex;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("OwnerAccount")
private String ownerAccount;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("OwnerId")
private Long ownerId;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("ResourceOwnerAccount")
private String resourceOwnerAccount;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("ResourceOwnerId")
private Long resourceOwnerId;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("StandbyHAMode")
private String standbyHAMode;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("StorageAutoScale")
private String storageAutoScale;
@com.aliyun.core.annotation.Query
@com.aliyun.core.annotation.NameInMap("StorageUpperBound")
@com.aliyun.core.annotation.Validation(maximum = 64000, minimum = 20)
private Long storageUpperBound;
private ModifyDBClusterRequest(Builder builder) {
super(builder);
this.compressStorage = builder.compressStorage;
this.DBClusterId = builder.DBClusterId;
this.DBNodeCrashList = builder.DBNodeCrashList;
this.dataSyncMode = builder.dataSyncMode;
this.faultInjectionType = builder.faultInjectionType;
this.faultSimulateMode = builder.faultSimulateMode;
this.imciAutoIndex = builder.imciAutoIndex;
this.ownerAccount = builder.ownerAccount;
this.ownerId = builder.ownerId;
this.resourceOwnerAccount = builder.resourceOwnerAccount;
this.resourceOwnerId = builder.resourceOwnerId;
this.standbyHAMode = builder.standbyHAMode;
this.storageAutoScale = builder.storageAutoScale;
this.storageUpperBound = builder.storageUpperBound;
}
public static Builder builder() {
return new Builder();
}
public static ModifyDBClusterRequest create() {
return builder().build();
}
@Override
public Builder toBuilder() {
return new Builder(this);
}
/**
* @return compressStorage
*/
public String getCompressStorage() {
return this.compressStorage;
}
/**
* @return DBClusterId
*/
public String getDBClusterId() {
return this.DBClusterId;
}
/**
* @return DBNodeCrashList
*/
public String getDBNodeCrashList() {
return this.DBNodeCrashList;
}
/**
* @return dataSyncMode
*/
public String getDataSyncMode() {
return this.dataSyncMode;
}
/**
* @return faultInjectionType
*/
public String getFaultInjectionType() {
return this.faultInjectionType;
}
/**
* @return faultSimulateMode
*/
public String getFaultSimulateMode() {
return this.faultSimulateMode;
}
/**
* @return imciAutoIndex
*/
public String getImciAutoIndex() {
return this.imciAutoIndex;
}
/**
* @return ownerAccount
*/
public String getOwnerAccount() {
return this.ownerAccount;
}
/**
* @return ownerId
*/
public Long getOwnerId() {
return this.ownerId;
}
/**
* @return resourceOwnerAccount
*/
public String getResourceOwnerAccount() {
return this.resourceOwnerAccount;
}
/**
* @return resourceOwnerId
*/
public Long getResourceOwnerId() {
return this.resourceOwnerId;
}
/**
* @return standbyHAMode
*/
public String getStandbyHAMode() {
return this.standbyHAMode;
}
/**
* @return storageAutoScale
*/
public String getStorageAutoScale() {
return this.storageAutoScale;
}
/**
* @return storageUpperBound
*/
public Long getStorageUpperBound() {
return this.storageUpperBound;
}
public static final class Builder extends Request.Builder {
private String compressStorage;
private String DBClusterId;
private String DBNodeCrashList;
private String dataSyncMode;
private String faultInjectionType;
private String faultSimulateMode;
private String imciAutoIndex;
private String ownerAccount;
private Long ownerId;
private String resourceOwnerAccount;
private Long resourceOwnerId;
private String standbyHAMode;
private String storageAutoScale;
private Long storageUpperBound;
private Builder() {
super();
}
private Builder(ModifyDBClusterRequest request) {
super(request);
this.compressStorage = request.compressStorage;
this.DBClusterId = request.DBClusterId;
this.DBNodeCrashList = request.DBNodeCrashList;
this.dataSyncMode = request.dataSyncMode;
this.faultInjectionType = request.faultInjectionType;
this.faultSimulateMode = request.faultSimulateMode;
this.imciAutoIndex = request.imciAutoIndex;
this.ownerAccount = request.ownerAccount;
this.ownerId = request.ownerId;
this.resourceOwnerAccount = request.resourceOwnerAccount;
this.resourceOwnerId = request.resourceOwnerId;
this.standbyHAMode = request.standbyHAMode;
this.storageAutoScale = request.storageAutoScale;
this.storageUpperBound = request.storageUpperBound;
}
/**
* Specifies whether to enable storage compression. Set the value to ON.
*
* example:
* ON
*/
public Builder compressStorage(String compressStorage) {
this.putQueryParameter("CompressStorage", compressStorage);
this.compressStorage = compressStorage;
return this;
}
/**
* The cluster ID.
*
* You can call the DescribeDBClusters operation to query information about all PolarDB clusters that are deployed in a specified region, such as cluster IDs.
*
* This parameter is required.
*
* example:
* pc-*************
*/
public Builder DBClusterId(String DBClusterId) {
this.putQueryParameter("DBClusterId", DBClusterId);
this.DBClusterId = DBClusterId;
return this;
}
/**
* The list of nodes for the drill.
*
* You can specify only one node for a node-level disaster recovery drill. For a primary zone-level disaster recovery drill, you can either choose not to specify this parameter or specify all nodes.
*
*
* example:
* pi-rwxxx
*/
public Builder DBNodeCrashList(String DBNodeCrashList) {
this.putQueryParameter("DBNodeCrashList", DBNodeCrashList);
this.DBNodeCrashList = DBNodeCrashList;
return this;
}
/**
* The method used to replicate data across zones. Valid values:
*
* - AsyncSync: the asynchronous mode.
* - SemiSync: the semi-synchronous mode.
*
*
* example:
* AsynSync
*/
public Builder dataSyncMode(String dataSyncMode) {
this.putQueryParameter("DataSyncMode", dataSyncMode);
this.dataSyncMode = dataSyncMode;
return this;
}
/**
* The fault injection method. Valid values:
*
* - CrashSQLInjection:
Crash SQL
-based fault injection.
*
*
* example:
* 0
*/
public Builder faultInjectionType(String faultInjectionType) {
this.putQueryParameter("FaultInjectionType", faultInjectionType);
this.faultInjectionType = faultInjectionType;
return this;
}
/**
* The level of the disaster recovery drill. Valid values:
*
* 0
or FaultInjection
: The primary zone level.
* 1
: The node level.
*
*
*
*
* In primary zone-level disaster recovery drill scenarios, all compute nodes in the primary zone are unavailable. Data loss occurs during failovers in the scenarios.
*
* In node-level disaster recovery drill scenarios, you can specify only one compute node for the disaster recovery drill. You can use the DBNodeCrashList
parameter to specify the name of the compute node that you want to use for the drill.
*
*
*
* example:
* 0
*/
public Builder faultSimulateMode(String faultSimulateMode) {
this.putQueryParameter("FaultSimulateMode", faultSimulateMode);
this.faultSimulateMode = faultSimulateMode;
return this;
}
/**
* Specifies whether to enable automatic IMCI-based query acceleration. IMCI is short for In-Memory Column Index. Valid values:
*
* ON
: enables automatic IMCI-based query acceleration.
* OFF
: disables automatic IMCI-based query acceleration.
*
*
*
*
* This parameter is supported only for PolarDB for MySQL clusters.
*
* For information about the cluster version limits, see Automatic IMCI-based query acceleration.
*
*
*
* example:
* OFF
*/
public Builder imciAutoIndex(String imciAutoIndex) {
this.putQueryParameter("ImciAutoIndex", imciAutoIndex);
this.imciAutoIndex = imciAutoIndex;
return this;
}
/**
* OwnerAccount.
*/
public Builder ownerAccount(String ownerAccount) {
this.putQueryParameter("OwnerAccount", ownerAccount);
this.ownerAccount = ownerAccount;
return this;
}
/**
* OwnerId.
*/
public Builder ownerId(Long ownerId) {
this.putQueryParameter("OwnerId", ownerId);
this.ownerId = ownerId;
return this;
}
/**
* ResourceOwnerAccount.
*/
public Builder resourceOwnerAccount(String resourceOwnerAccount) {
this.putQueryParameter("ResourceOwnerAccount", resourceOwnerAccount);
this.resourceOwnerAccount = resourceOwnerAccount;
return this;
}
/**
* ResourceOwnerId.
*/
public Builder resourceOwnerId(Long resourceOwnerId) {
this.putQueryParameter("ResourceOwnerId", resourceOwnerId);
this.resourceOwnerId = resourceOwnerId;
return this;
}
/**
* Specifies whether to enable cross-zone automatic switchover. Valid values:
*
* - ON: enables cross-zone automatic switchover.
* - OFF: disables cross-zone automatic switchover.
*
*
* example:
* ON
*/
public Builder standbyHAMode(String standbyHAMode) {
this.putQueryParameter("StandbyHAMode", standbyHAMode);
this.standbyHAMode = standbyHAMode;
return this;
}
/**
* Specifies whether to enable automatic storage scaling. This parameter is available only for Standard Edition clusters. Valid values:
*
* - Enable
* - Disable
*
*
* example:
* Enable
*/
public Builder storageAutoScale(String storageAutoScale) {
this.putQueryParameter("StorageAutoScale", storageAutoScale);
this.storageAutoScale = storageAutoScale;
return this;
}
/**
* The maximum storage capacity of the cluster of Standard Edition in automatic scaling. Unit: GB.
*
* The maximum value of this parameter is 32000.
*
*
* example:
* 800
*/
public Builder storageUpperBound(Long storageUpperBound) {
this.putQueryParameter("StorageUpperBound", storageUpperBound);
this.storageUpperBound = storageUpperBound;
return this;
}
@Override
public ModifyDBClusterRequest build() {
return new ModifyDBClusterRequest(this);
}
}
}