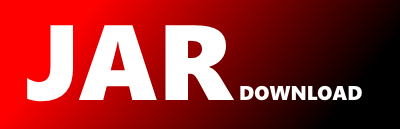
com.aliyun.sdk.service.waf_openapi20211001.models.DescribeDefenseTemplatesResponseBody Maven / Gradle / Ivy
Show all versions of alibabacloud-waf_openapi20211001 Show documentation
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.sdk.service.waf_openapi20211001.models;
import darabonba.core.RequestModel;
import darabonba.core.TeaModel;
import com.aliyun.sdk.gateway.pop.models.*;
/**
*
* {@link DescribeDefenseTemplatesResponseBody} extends {@link TeaModel}
*
* DescribeDefenseTemplatesResponseBody
*/
public class DescribeDefenseTemplatesResponseBody extends TeaModel {
@com.aliyun.core.annotation.NameInMap("RequestId")
private String requestId;
@com.aliyun.core.annotation.NameInMap("Templates")
private java.util.List < Templates> templates;
@com.aliyun.core.annotation.NameInMap("TotalCount")
private Long totalCount;
private DescribeDefenseTemplatesResponseBody(Builder builder) {
this.requestId = builder.requestId;
this.templates = builder.templates;
this.totalCount = builder.totalCount;
}
public static Builder builder() {
return new Builder();
}
public static DescribeDefenseTemplatesResponseBody create() {
return builder().build();
}
/**
* @return requestId
*/
public String getRequestId() {
return this.requestId;
}
/**
* @return templates
*/
public java.util.List < Templates> getTemplates() {
return this.templates;
}
/**
* @return totalCount
*/
public Long getTotalCount() {
return this.totalCount;
}
public static final class Builder {
private String requestId;
private java.util.List < Templates> templates;
private Long totalCount;
/**
* The request ID.
*
* example:
* 4F26D2F1-E288-5104-8518-05E240E3****
*/
public Builder requestId(String requestId) {
this.requestId = requestId;
return this;
}
/**
* The protection templates.
*/
public Builder templates(java.util.List < Templates> templates) {
this.templates = templates;
return this;
}
/**
* The total number of entries returned.
*
* example:
* 100
*/
public Builder totalCount(Long totalCount) {
this.totalCount = totalCount;
return this;
}
public DescribeDefenseTemplatesResponseBody build() {
return new DescribeDefenseTemplatesResponseBody(this);
}
}
/**
*
* {@link DescribeDefenseTemplatesResponseBody} extends {@link TeaModel}
*
* DescribeDefenseTemplatesResponseBody
*/
public static class Templates extends TeaModel {
@com.aliyun.core.annotation.NameInMap("DefenseScene")
private String defenseScene;
@com.aliyun.core.annotation.NameInMap("DefenseSubScene")
private String defenseSubScene;
@com.aliyun.core.annotation.NameInMap("Description")
private String description;
@com.aliyun.core.annotation.NameInMap("GmtModified")
private Long gmtModified;
@com.aliyun.core.annotation.NameInMap("TemplateId")
private Long templateId;
@com.aliyun.core.annotation.NameInMap("TemplateName")
private String templateName;
@com.aliyun.core.annotation.NameInMap("TemplateOrigin")
private String templateOrigin;
@com.aliyun.core.annotation.NameInMap("TemplateStatus")
private Integer templateStatus;
@com.aliyun.core.annotation.NameInMap("TemplateType")
private String templateType;
private Templates(Builder builder) {
this.defenseScene = builder.defenseScene;
this.defenseSubScene = builder.defenseSubScene;
this.description = builder.description;
this.gmtModified = builder.gmtModified;
this.templateId = builder.templateId;
this.templateName = builder.templateName;
this.templateOrigin = builder.templateOrigin;
this.templateStatus = builder.templateStatus;
this.templateType = builder.templateType;
}
public static Builder builder() {
return new Builder();
}
public static Templates create() {
return builder().build();
}
/**
* @return defenseScene
*/
public String getDefenseScene() {
return this.defenseScene;
}
/**
* @return defenseSubScene
*/
public String getDefenseSubScene() {
return this.defenseSubScene;
}
/**
* @return description
*/
public String getDescription() {
return this.description;
}
/**
* @return gmtModified
*/
public Long getGmtModified() {
return this.gmtModified;
}
/**
* @return templateId
*/
public Long getTemplateId() {
return this.templateId;
}
/**
* @return templateName
*/
public String getTemplateName() {
return this.templateName;
}
/**
* @return templateOrigin
*/
public String getTemplateOrigin() {
return this.templateOrigin;
}
/**
* @return templateStatus
*/
public Integer getTemplateStatus() {
return this.templateStatus;
}
/**
* @return templateType
*/
public String getTemplateType() {
return this.templateType;
}
public static final class Builder {
private String defenseScene;
private String defenseSubScene;
private String description;
private Long gmtModified;
private Long templateId;
private String templateName;
private String templateOrigin;
private Integer templateStatus;
private String templateType;
/**
* The scenario in which the protection template is used.
*
* - waf_group: basic protection.
* - antiscan: scan protection.
* - ip_blacklist: IP address blacklist.
* - custom_acl: custom rule.
* - whitelist: whitelist.
* - region_block: region blacklist.
* - custom_response: custom response.
* - cc: HTTP flood protection.
* - tamperproof: website tamper-proofing.
* - dlp: data leakage prevention.
*
*
* example:
* whitelist
*/
public Builder defenseScene(String defenseScene) {
this.defenseScene = defenseScene;
return this;
}
/**
* The sub-scenario in which the protection template is used. Valid values:
*
* - web: bot management for website protection.
* - app: bot management for app protection.
* - basic: bot management for basic protection.
*
*
* example:
* basic
*/
public Builder defenseSubScene(String defenseSubScene) {
this.defenseSubScene = defenseSubScene;
return this;
}
/**
* The description of the protection template.
*
* example:
* test
*/
public Builder description(String description) {
this.description = description;
return this;
}
/**
* The time when the protection template was created. This value is a UNIX timestamp representing the number of milliseconds that have elapsed since January 1, 1970, 00:00:00 UTC.
*
* example:
* 1683776070000
*/
public Builder gmtModified(Long gmtModified) {
this.gmtModified = gmtModified;
return this;
}
/**
* The ID of the protection template.
*
* example:
* 12345
*/
public Builder templateId(Long templateId) {
this.templateId = templateId;
return this;
}
/**
* The name of the protection template.
*
* example:
* testTemplateName
*/
public Builder templateName(String templateName) {
this.templateName = templateName;
return this;
}
/**
* The origin of the protection template. The value custom indicates that the protection template is a custom template created by the user.
*
* example:
* custom
*/
public Builder templateOrigin(String templateOrigin) {
this.templateOrigin = templateOrigin;
return this;
}
/**
* The status of the protection template. Valid values:
*
* - 0: disabled.
* - 1: enabled.
*
*
* example:
* 1
*/
public Builder templateStatus(Integer templateStatus) {
this.templateStatus = templateStatus;
return this;
}
/**
* The type of the protection template. Valid values:
*
* - user_default: default template.
* - user_custom: custom template.
*
*
* example:
* user_custom
*/
public Builder templateType(String templateType) {
this.templateType = templateType;
return this;
}
public Templates build() {
return new Templates(this);
}
}
}
}