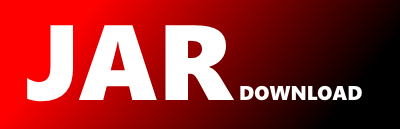
com.aliyun.httpcomponent.httpclient.implementation.StreamEntityProducer Maven / Gradle / Ivy
The newest version!
package com.aliyun.httpcomponent.httpclient.implementation;
import com.aliyun.apache.hc.core5.http.nio.AsyncEntityProducer;
import com.aliyun.apache.hc.core5.http.nio.DataStreamChannel;
import java.io.IOException;
import java.io.InputStream;
import java.nio.ByteBuffer;
import java.util.Set;
public final class StreamEntityProducer implements AsyncEntityProducer {
private InputStream data;
private long contentLength;
private ByteBuffer buffer;
private boolean gotEOF;
public StreamEntityProducer(InputStream inputstream) {
this.data = inputstream;
this.gotEOF = false;
this.buffer = null;
this.contentLength = -1;
}
@Override
public boolean isRepeatable() {
return false;
}
@Override
public void failed(Exception e) {
releaseResources();
}
@Override
public long getContentLength() {
return contentLength;
}
@Override
public String getContentType() {
return null;
}
@Override
public String getContentEncoding() {
return null;
}
@Override
public boolean isChunked() {
return contentLength == -1;
}
@Override
public Set getTrailerNames() {
return null;
}
@Override
public int available() {
return Integer.MAX_VALUE;
}
@Override
public void produce(DataStreamChannel dataStreamChannel) throws IOException {
fillBuffer();
while (buffer != null && buffer.remaining() > 0) {
dataStreamChannel.write(buffer);
if (buffer.remaining() > 0) {
break;
} else {
fillBuffer();
}
}
if (gotEOF && (buffer == null || !buffer.hasRemaining())) {
dataStreamChannel.endStream();
}
}
@Override
public void releaseResources() {
}
private void fillBuffer() throws IOException {
if (buffer == null || !buffer.hasRemaining()) {
byte[] src = new byte[4096];
int ret = data.read(src);
if (ret < 0) {
gotEOF = true;
return;
}
buffer = ByteBuffer.wrap(src, 0, ret);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy