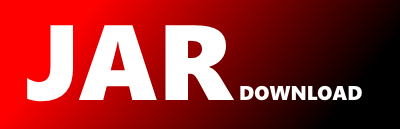
com.aliyun.httpcomponent.httpclient.implementation.reactive.ReactiveResponseConsumer Maven / Gradle / Ivy
The newest version!
package com.aliyun.httpcomponent.httpclient.implementation.reactive;
import com.aliyun.core.http.HttpHeaders;
import com.aliyun.core.http.HttpResponseHandler;
import com.aliyun.apache.hc.core5.concurrent.BasicFuture;
import com.aliyun.apache.hc.core5.concurrent.FutureCallback;
import com.aliyun.apache.hc.core5.http.EntityDetails;
import com.aliyun.apache.hc.core5.http.Header;
import com.aliyun.apache.hc.core5.http.HttpResponse;
import com.aliyun.apache.hc.core5.http.nio.AsyncResponseConsumer;
import com.aliyun.apache.hc.core5.http.nio.CapacityChannel;
import com.aliyun.apache.hc.core5.http.protocol.HttpContext;
import java.io.IOException;
import java.nio.ByteBuffer;
import java.util.*;
public final class ReactiveResponseConsumer implements AsyncResponseConsumer {
private final ReactiveDataConsumer reactiveDataConsumer = new ReactiveDataConsumer();
private final List trailers = Collections.synchronizedList(new ArrayList());
private volatile BasicFuture responseCompletion;
private volatile HttpResponse informationResponse;
private volatile EntityDetails entityDetails;
private HttpResponseHandler handler;
private HttpResponse response;
private com.aliyun.core.http.HttpHeaders sdkHttpHeaders;
/**
* Creates a {@code ReactiveResponseConsumer}.
*/
public ReactiveResponseConsumer(HttpResponseHandler handler) {
this.handler = handler;
}
/**
* Returns the intermediate (1xx) HTTP response if one was received.
*
* @return the information response, or {@code null} if none.
*/
public HttpResponse getInformationResponse() {
return informationResponse;
}
/**
* Returns the response entity details.
*
* @return the entity details, or {@code null} if none.
*/
public EntityDetails getEntityDetails() {
return entityDetails;
}
/**
* Returns the trailers received at the end of the response.
*
* @return a non-null list of zero or more trailers.
*/
public List getTrailers() {
return trailers;
}
@Override
public void consumeResponse(
final HttpResponse response,
final EntityDetails entityDetails,
final HttpContext httpContext,
final FutureCallback resultCallback
) {
this.response = response;
this.entityDetails = entityDetails;
this.responseCompletion = new BasicFuture<>(resultCallback);
this.sdkHttpHeaders = toHeaders(response);
this.handler.onStream(reactiveDataConsumer, response.getCode(), sdkHttpHeaders);
if (entityDetails == null) {
streamEnd(null);
}
}
@Override
public void informationResponse(final HttpResponse response, final HttpContext httpContext) {
this.informationResponse = response;
}
@Override
public void failed(final Exception cause) {
reactiveDataConsumer.failed(cause);
if (responseCompletion != null) {
responseCompletion.failed(cause);
}
}
@Override
public void updateCapacity(final CapacityChannel capacityChannel) throws IOException {
reactiveDataConsumer.updateCapacity(capacityChannel);
}
@Override
public void consume(final ByteBuffer src) throws IOException {
reactiveDataConsumer.consume(src);
}
@Override
public void streamEnd(final List extends Header> trailers) {
if (trailers != null) {
this.trailers.addAll(trailers);
}
reactiveDataConsumer.streamEnd(trailers);
ReactiveApacheHttpResponse result = ReactiveApacheHttpResponse.copyLite(this.response, this.sdkHttpHeaders);
if (responseCompletion != null) {
responseCompletion.completed(result);
}
}
@Override
public void releaseResources() {
reactiveDataConsumer.releaseResources();
if (responseCompletion != null) {
responseCompletion.cancel();
}
}
private static com.aliyun.core.http.HttpHeaders toHeaders(HttpResponse response) {
final HttpHeaders httpHeaders = new HttpHeaders();
Set nameSet = new HashSet<>();
Header[] apacheHeaders = response.getHeaders();
for (Header header : apacheHeaders) {
nameSet.add(header.getName());
}
nameSet.forEach((name) -> {
Header[] entityHeaders = response.getHeaders(name);
List values = new ArrayList<>();
for (Header entityHeader : entityHeaders) {
values.add(entityHeader.getValue());
}
httpHeaders.set(name, values);
});
return httpHeaders;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy