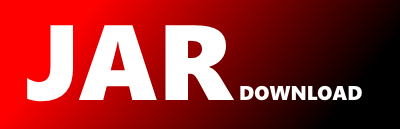
com.aliyun.auth.credentials.provider.ProfileCredentialProvider Maven / Gradle / Ivy
The newest version!
package com.aliyun.auth.credentials.provider;
import com.aliyun.auth.credentials.Credential;
import com.aliyun.auth.credentials.ICredential;
import com.aliyun.auth.credentials.exception.*;
import com.aliyun.auth.credentials.utils.*;
import com.aliyun.core.utils.StringUtils;
import java.io.IOException;
import java.util.HashMap;
import java.util.Map;
public class ProfileCredentialProvider implements ICredentialProvider {
private static volatile Map> ini;
private final String profileFile;
private final String clientType;
private final ICredentialProvider credentialProvider;
private final boolean asyncCredentialUpdateEnabled;
private ProfileCredentialProvider(Builder builder) {
this.profileFile = builder.profileFile == null ? AuthConstant.DEFAULT_CREDENTIALS_FILE_PATH : builder.profileFile;
this.clientType = builder.clientType == null ? AuthConstant.DEFAULT_CLIENT_TYPE : builder.clientType;
this.asyncCredentialUpdateEnabled = builder.asyncCredentialUpdateEnabled;
if (this.profileFile.length() == 0) {
throw new CredentialException("The specified credentials file is empty.");
}
Map> ini;
try {
ini = getIni(this.profileFile);
} catch (IOException e) {
throw new CredentialException("The specified credentials file is not exist.");
}
Map> client = loadIni(ini);
Map clientConfig = client.get(this.clientType);
if (clientConfig == null) {
throw new CredentialException("Client is not open in the specified credentials file.");
}
this.credentialProvider = createCredentialProvider(clientConfig);
}
public static Builder builder() {
return new Builder();
}
public static ProfileCredentialProvider create() {
return builder().build();
}
public static ProfileCredentialProvider create(String clientType) {
return builder().clientType(clientType).build();
}
@Override
public ICredential getCredentials() throws CredentialException {
return credentialProvider.getCredentials();
}
@Override
public void close() {
}
private static Map> getIni(String profileFile) throws IOException {
if (null == ini) {
ini = ProfileUtils.parseFile(profileFile);
}
return ini;
}
private Map> loadIni(Map> ini) {
Map> client = new HashMap>(16);
String enable;
for (Map.Entry> clientType : ini.entrySet()) {
enable = clientType.getValue().get(AuthConstant.INI_ENABLE);
if (Boolean.parseBoolean(enable)) {
Map clientConfig = new HashMap(16);
for (Map.Entry enabledClient : clientType.getValue().entrySet()) {
clientConfig.put(enabledClient.getKey(), enabledClient.getValue());
}
client.put(clientType.getKey(), clientConfig);
}
}
return client;
}
private ICredentialProvider createCredentialProvider(Map clientConfig) {
String configType = clientConfig.get(AuthConstant.INI_TYPE);
if (StringUtils.isEmpty(configType)) {
throw new CredentialException("The configured client type is empty.");
}
if (AuthConstant.INI_TYPE_ARN.equals(configType)) {
return getSTSAssumeRoleSessionCredentialProvider(clientConfig);
}
if (AuthConstant.INI_TYPE_KEY_PAIR.equals(configType)) {
return getSTSGetSessionAccessKeyCredentialProvider(clientConfig);
}
if (AuthConstant.INI_TYPE_RAM.equals(configType)) {
return getInstanceProfileCredentialProvider(clientConfig);
}
if (AuthConstant.INI_TYPE_OIDC.equals(configType)) {
return getSTSOIDCRoleSessionCredentials(clientConfig);
}
String accessKeyId = clientConfig.get(AuthConstant.INI_ACCESS_KEY_ID);
String accessKeySecret = clientConfig.get(AuthConstant.INI_ACCESS_KEY_IDSECRET);
if (StringUtils.isEmpty(accessKeyId) || StringUtils.isEmpty(accessKeySecret)) {
throw new CredentialException("The configured access_key_id or access_key_secret is empty");
}
return StaticCredentialProvider.create(Credential.builder()
.accessKeyId(accessKeyId)
.accessKeySecret(accessKeySecret)
.build());
}
private ICredentialProvider getSTSAssumeRoleSessionCredentialProvider(Map clientConfig) {
String accessKeyId = clientConfig.get(AuthConstant.INI_ACCESS_KEY_ID);
String accessKeySecret = clientConfig.get(AuthConstant.INI_ACCESS_KEY_IDSECRET);
String roleSessionName = clientConfig.get(AuthConstant.INI_ROLE_SESSION_NAME);
String roleArn = clientConfig.get(AuthConstant.INI_ROLE_ARN);
String regionId = clientConfig.get(AuthConstant.DEFAULT_REGION);
String policy = clientConfig.get(AuthConstant.INI_POLICY);
if (StringUtils.isEmpty(accessKeyId) || StringUtils.isEmpty(accessKeySecret)) {
throw new CredentialException("The configured access_key_id or access_key_secret is empty.");
}
if (StringUtils.isEmpty(roleSessionName) || StringUtils.isEmpty(roleArn)) {
throw new CredentialException("The configured role_session_name or role_arn is empty.");
}
return RamRoleArnCredentialProvider.builder()
.roleSessionName(roleSessionName)
.roleArn(roleArn)
.regionId(regionId)
.policy(policy)
.asyncCredentialUpdateEnabled(this.asyncCredentialUpdateEnabled)
.credential(Credential.builder()
.accessKeyId(accessKeyId)
.accessKeySecret(accessKeySecret)
.build())
.build();
}
private ICredentialProvider getSTSOIDCRoleSessionCredentials(Map clientConfig) {
String roleSessionName = clientConfig.get(AuthConstant.INI_ROLE_SESSION_NAME);
String roleArn = clientConfig.get(AuthConstant.INI_ROLE_ARN);
String OIDCProviderArn = clientConfig.get(AuthConstant.INI_OIDC_PROVIDER_ARN);
String OIDCTokenFilePath = clientConfig.get(AuthConstant.INI_OIDC_TOKEN_FILE_PATH);
String policy = clientConfig.get(AuthConstant.INI_POLICY);
if (StringUtils.isEmpty(roleArn)) {
throw new CredentialException("The configured role_arn is empty.");
}
if (StringUtils.isEmpty(OIDCProviderArn)) {
throw new CredentialException("The configured oidc_provider_arn is empty.");
}
if (StringUtils.isEmpty(OIDCTokenFilePath)) {
throw new CredentialException("The configured oidc_token_file_path is empty.");
}
return OIDCRoleArnCredentialProvider.builder()
.roleArn(roleArn)
.roleSessionName(roleSessionName)
.oidcProviderArn(OIDCProviderArn)
.oidcTokenFilePath(OIDCTokenFilePath)
.policy(policy)
.build();
}
public ICredentialProvider getSTSGetSessionAccessKeyCredentialProvider(Map clientConfig) {
String publicKeyId = clientConfig.get(AuthConstant.INI_PUBLIC_KEY_ID);
String privateKeyFile = clientConfig.get(AuthConstant.INI_PRIVATE_KEY_FILE);
if (StringUtils.isEmpty(privateKeyFile)) {
throw new CredentialException("The configured private_key_file is empty.");
}
String privateKey = AuthUtils.getPrivateKey(privateKeyFile);
if (StringUtils.isEmpty(publicKeyId) || StringUtils.isEmpty(privateKey)) {
throw new CredentialException("The configured public_key_id or private_key_file content is empty.");
}
return RsaKeyPairCredentialProvider.builder()
.asyncCredentialUpdateEnabled(this.asyncCredentialUpdateEnabled)
.credential(Credential.builder()
.accessKeyId(publicKeyId)
.accessKeySecret(privateKey)
.build())
.build();
}
private ICredentialProvider getInstanceProfileCredentialProvider(Map clientConfig) {
String roleName = clientConfig.get(AuthConstant.INI_ROLE_NAME);
if (StringUtils.isEmpty(roleName)) {
throw new CredentialException("The configured role_name is empty.");
}
return EcsRamRoleCredentialProvider.builder()
.asyncCredentialUpdateEnabled(this.asyncCredentialUpdateEnabled)
.roleName(roleName)
.build();
}
static final class Builder {
private String profileFile = AuthConstant.CREDENTIALS_FILE_PATH;
private String clientType = AuthConstant.CLIENT_TYPE;
private boolean asyncCredentialUpdateEnabled = false;
public Builder profileFile(String profileFile) {
this.profileFile = profileFile;
return this;
}
public Builder clientType(String clientType) {
this.clientType = clientType;
return this;
}
public Builder asyncCredentialUpdateEnabled(Boolean asyncCredentialUpdateEnabled) {
this.asyncCredentialUpdateEnabled = asyncCredentialUpdateEnabled;
return this;
}
public ProfileCredentialProvider build() {
return new ProfileCredentialProvider(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy