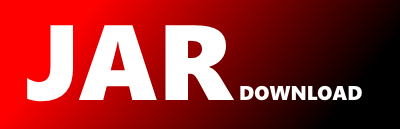
com.aliyun.auth.credentials.provider.RamRoleArnCredentialProvider Maven / Gradle / Ivy
The newest version!
package com.aliyun.auth.credentials.provider;
import com.aliyun.auth.credentials.Credential;
import com.aliyun.auth.credentials.ICredential;
import com.aliyun.auth.credentials.exception.*;
import com.aliyun.auth.credentials.http.*;
import com.aliyun.auth.credentials.utils.*;
import com.aliyun.core.utils.StringUtils;
import com.aliyun.core.utils.Validate;
import com.google.gson.Gson;
import java.time.Instant;
import java.util.Map;
public final class RamRoleArnCredentialProvider extends HttpCredentialProvider {
/**
* Default duration for started sessions. Unit of Second
*/
private int durationSeconds;
/**
* The arn of the role to be assumed.
*/
private String roleArn;
/**
* An identifier for the assumed role session.
*/
private final String roleSessionName;
private String policy;
private String externalId;
/**
* Unit of millisecond
*/
private int connectionTimeout;
private int readTimeout;
private final ICredentialProvider credentialsProvider;
/**
* Endpoint of RAM OpenAPI
*/
private final String stsEndpoint;
private final CompatibleUrlConnClient client;
private String protocol = "https";
private RamRoleArnCredentialProvider(BuilderImpl builder) {
super(builder);
this.roleSessionName = builder.roleSessionName == null ? !StringUtils.isEmpty(AuthUtils.getEnvironmentRoleSessionName()) ?
AuthUtils.getEnvironmentRoleSessionName() : "aliyun-java-auth-" + System.currentTimeMillis() : builder.roleSessionName;
this.durationSeconds = builder.durationSeconds == null ? 3600 : builder.durationSeconds;
if (this.durationSeconds < 900) {
throw new IllegalArgumentException("Session duration should be in the range of 900s - max session duration.");
}
this.roleArn = builder.roleArn == null ? AuthUtils.getEnvironmentRoleArn() : builder.roleArn;
if (StringUtils.isEmpty(this.roleArn)) {
throw new IllegalArgumentException("RoleArn or environment variable ALIBABA_CLOUD_ROLE_ARN cannot be empty.");
}
this.policy = builder.policy;
this.externalId = builder.externalId;
this.connectionTimeout = builder.connectionTimeout == null ? 5000 : builder.connectionTimeout;
this.readTimeout = builder.readTimeout == null ? 10000 : builder.readTimeout;
if (!StringUtils.isEmpty(builder.stsEndpoint)) {
this.stsEndpoint = builder.stsEndpoint;
} else {
String prefix = builder.enableVpc != null ? (builder.enableVpc ? "sts-vpc" : "sts") : AuthUtils.isEnableVpcEndpoint() ? "sts-vpc" : "sts";
if (!StringUtils.isEmpty(builder.stsRegionId)) {
this.stsEndpoint = String.format("%s.%s.aliyuncs.com", prefix, builder.stsRegionId);
} else if (!StringUtils.isEmpty(AuthUtils.getEnvironmentSTSRegion())) {
this.stsEndpoint = String.format("%s.%s.aliyuncs.com", prefix, AuthUtils.getEnvironmentSTSRegion());
} else {
this.stsEndpoint = "sts.aliyuncs.com";
}
}
if (null != builder.credentialsProvider) {
this.credentialsProvider = builder.credentialsProvider;
} else {
Validate.notNull(builder.credential, "Credentials must not be null.");
this.credentialsProvider = StaticCredentialProvider.create(builder.credential);
}
this.client = new CompatibleUrlConnClient();
this.buildRefreshCache();
}
public static RamRoleArnCredentialProvider create(Credential credential) {
return builder().credential(credential).build();
}
public static Builder builder() {
return new BuilderImpl();
}
public String getStsEndpoint() {
return this.stsEndpoint;
}
@Override
public RefreshResult refreshCredentials() {
ParameterHelper parameterHelper = new ParameterHelper();
HttpRequest httpRequest = new HttpRequest();
httpRequest.setUrlParameter("Action", "AssumeRole");
httpRequest.setUrlParameter("Format", "JSON");
httpRequest.setUrlParameter("Version", "2015-04-01");
httpRequest.setUrlParameter("DurationSeconds", String.valueOf(durationSeconds));
httpRequest.setUrlParameter("RoleArn", roleArn);
httpRequest.setUrlParameter("RoleSessionName", roleSessionName);
if (policy != null) {
httpRequest.setUrlParameter("Policy", policy);
}
if (externalId != null) {
httpRequest.setUrlParameter("ExternalId", this.externalId);
}
httpRequest.setSysMethod(MethodType.GET);
httpRequest.setSysConnectTimeout(this.connectionTimeout);
httpRequest.setSysReadTimeout(this.readTimeout);
ICredential credentials = this.credentialsProvider.getCredentials();
Validate.notNull(credentials, "Unable to load original credentials from the providers in RAM role arn.");
httpRequest.setUrlParameter("AccessKeyId", credentials.accessKeyId());
if (!StringUtils.isEmpty(credentials.securityToken())) {
httpRequest.setUrlParameter("SecurityToken", credentials.securityToken());
}
String strToSign = parameterHelper.composeStringToSign(MethodType.GET, httpRequest.getUrlParameters());
String signature = parameterHelper.signString(strToSign, credentials.accessKeySecret() + "&");
httpRequest.setUrlParameter("Signature", signature);
httpRequest.setSysUrl(parameterHelper.composeUrl(this.stsEndpoint, httpRequest.getUrlParameters(),
protocol));
HttpResponse httpResponse;
try {
httpResponse = client.syncInvoke(httpRequest);
} catch (Exception e) {
throw new CredentialException("Failed to connect RamRoleArn Service: " + e);
}
if (httpResponse.getResponseCode() != 200) {
throw new CredentialException(String.format("Error refreshing credentials from RamRoleArn, HttpCode: %s, result: %s.", httpResponse.getResponseCode(), httpResponse.getHttpContentString()));
}
Gson gson = new Gson();
Map map = gson.fromJson(httpResponse.getHttpContentString(), Map.class);
if (null == map || !map.containsKey("Credentials")) {
throw new CredentialException(String.format("Error retrieving credentials from RamRoleArn result: %s.", httpResponse.getHttpContentString()));
}
Map result = (Map) map.get("Credentials");
if (!result.containsKey("AccessKeyId") || !result.containsKey("AccessKeySecret") || !result.containsKey("SecurityToken")) {
throw new CredentialException(String.format("Error retrieving credentials from RamRoleArn result: %s.", httpResponse.getHttpContentString()));
}
Instant expiration = ParameterHelper.getUTCDate(result.get("Expiration")).toInstant();
ICredential credential = Credential.builder()
.accessKeyId(result.get("AccessKeyId"))
.accessKeySecret(result.get("AccessKeySecret"))
.securityToken(result.get("SecurityToken"))
.build();
return RefreshResult.builder(credential)
.staleTime(getStaleTime(expiration))
.prefetchTime(getPrefetchTime(expiration))
.build();
}
@Override
public void close() {
super.close();
this.client.close();
}
public interface Builder extends HttpCredentialProvider.Builder {
Builder roleSessionName(String roleSessionName);
Builder durationSeconds(Integer durationSeconds);
Builder roleArn(String roleArn);
Builder regionId(String regionId);
Builder policy(String policy);
Builder connectionTimeout(Integer connectionTimeout);
Builder readTimeout(Integer readTimeout);
Builder credential(Credential credential);
Builder credentialsProvider(ICredentialProvider credentialsProvider);
Builder stsEndpoint(String stsEndpoint);
Builder stsRegionId(String stsRegionId);
Builder enableVpc(Boolean enableVpc);
Builder externalId(String externalId);
@Override
RamRoleArnCredentialProvider build();
}
private static final class BuilderImpl
extends HttpCredentialProvider.BuilderImpl
implements Builder {
private String roleSessionName;
private Integer durationSeconds;
private String roleArn;
private String regionId;
private String policy;
private Integer connectionTimeout;
private Integer readTimeout;
private Credential credential;
private String stsEndpoint;
private String stsRegionId;
private Boolean enableVpc;
private ICredentialProvider credentialsProvider;
private String externalId;
public Builder roleSessionName(String roleSessionName) {
this.roleSessionName = roleSessionName;
return this;
}
public Builder durationSeconds(Integer durationSeconds) {
this.durationSeconds = durationSeconds;
return this;
}
public Builder roleArn(String roleArn) {
this.roleArn = roleArn;
return this;
}
public Builder regionId(String regionId) {
this.regionId = regionId;
return this;
}
public Builder policy(String policy) {
this.policy = policy;
return this;
}
public Builder connectionTimeout(Integer connectionTimeout) {
this.connectionTimeout = connectionTimeout;
return this;
}
public Builder readTimeout(Integer readTimeout) {
this.readTimeout = readTimeout;
return this;
}
public Builder credential(Credential credential) {
this.credential = credential;
return this;
}
public Builder credentialsProvider(ICredentialProvider credentialsProvider) {
this.credentialsProvider = credentialsProvider;
return this;
}
public Builder stsEndpoint(String stsEndpoint) {
this.stsEndpoint = stsEndpoint;
return this;
}
public Builder stsRegionId(String stsRegionId) {
this.stsRegionId = stsRegionId;
return this;
}
public Builder enableVpc(Boolean enableVpc) {
this.enableVpc = enableVpc;
return this;
}
public Builder externalId(String externalId) {
this.externalId = externalId;
return this;
}
@Override
public RamRoleArnCredentialProvider build() {
return new RamRoleArnCredentialProvider(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy