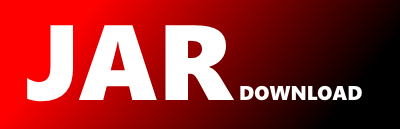
com.aliyun.auth.credentials.provider.RsaKeyPairCredentialProvider Maven / Gradle / Ivy
The newest version!
package com.aliyun.auth.credentials.provider;
import com.aliyun.auth.credentials.Credential;
import com.aliyun.auth.credentials.ICredential;
import com.aliyun.auth.credentials.exception.*;
import com.aliyun.auth.credentials.http.*;
import com.aliyun.auth.credentials.utils.*;
import com.aliyun.core.utils.StringUtils;
import com.aliyun.core.utils.Validate;
import com.google.gson.Gson;
import java.time.Instant;
import java.util.Map;
public class RsaKeyPairCredentialProvider extends HttpCredentialProvider {
/**
* Default duration for started sessions. Unit of Second
*/
private int durationSeconds;
private String regionId;
/**
* Unit of millisecond
*/
private int connectionTimeout;
private int readTimeout;
private Credential credential;
private final String stsEndpoint;
private final CompatibleUrlConnClient client;
private RsaKeyPairCredentialProvider(BuilderImpl builder) {
super(builder);
this.durationSeconds = builder.durationSeconds == null ? 3600 : builder.durationSeconds;
if (this.durationSeconds < 900) {
throw new IllegalArgumentException("Session duration should be in the range of 900s - max session duration.");
}
this.regionId = builder.regionId;
this.connectionTimeout = builder.connectionTimeout == null ? 5000 : builder.connectionTimeout;
this.readTimeout = builder.readTimeout == null ? 10000 : builder.readTimeout;
this.credential = Validate.notNull(builder.credential, "Credentials must not be null.");
if (!StringUtils.isEmpty(builder.stsEndpoint)) {
this.stsEndpoint = builder.stsEndpoint;
} else {
String prefix = builder.enableVpc != null ? (builder.enableVpc ? "sts-vpc" : "sts") : AuthUtils.isEnableVpcEndpoint() ? "sts-vpc" : "sts";
if (!StringUtils.isEmpty(builder.stsRegionId)) {
this.stsEndpoint = String.format("%s.%s.aliyuncs.com", prefix, builder.stsRegionId);
} else if (!StringUtils.isEmpty(AuthUtils.getEnvironmentSTSRegion())) {
this.stsEndpoint = String.format("%s.%s.aliyuncs.com", prefix, AuthUtils.getEnvironmentSTSRegion());
} else {
this.stsEndpoint = "sts.ap-northeast-1.aliyuncs.com";
}
}
this.client = new CompatibleUrlConnClient();
this.buildRefreshCache();
}
public static RsaKeyPairCredentialProvider create(Credential credential) {
return builder().credential(credential).build();
}
public static Builder builder() {
return new BuilderImpl();
}
@Override
public RefreshResult refreshCredentials() {
ParameterHelper parameterHelper = new ParameterHelper();
HttpRequest httpRequest = new HttpRequest();
httpRequest.setUrlParameter("Action", "GenerateSessionAccessKey");
httpRequest.setUrlParameter("Format", "JSON");
httpRequest.setUrlParameter("Version", "2015-04-01");
httpRequest.setUrlParameter("DurationSeconds", String.valueOf(durationSeconds));
httpRequest.setUrlParameter("AccessKeyId", credential.accessKeyId());
httpRequest.setUrlParameter("RegionId", this.regionId);
String strToSign = parameterHelper.composeStringToSign(MethodType.GET, httpRequest.getUrlParameters());
String signature = parameterHelper.signString(strToSign, credential.accessKeySecret() + "&");
httpRequest.setUrlParameter("Signature", signature);
httpRequest.setSysMethod(MethodType.GET);
httpRequest.setSysConnectTimeout(this.connectionTimeout);
httpRequest.setSysReadTimeout(this.readTimeout);
httpRequest.setSysUrl(parameterHelper.composeUrl(this.stsEndpoint, httpRequest.getUrlParameters(),
"https"));
HttpResponse httpResponse;
try {
httpResponse = client.syncInvoke(httpRequest);
} catch (Exception e) {
throw new CredentialException("Failed to connect RsaKeyPair Service: " + e);
}
if (httpResponse.getResponseCode() != 200) {
throw new CredentialException(String.format("Error refreshing credentials from RsaKeyPair, HttpCode: %s, result: %s.", httpResponse.getResponseCode(), httpResponse.getHttpContentString()));
}
Gson gson = new Gson();
Map map = gson.fromJson(httpResponse.getHttpContentString(), Map.class);
if (null == map || !map.containsKey("SessionAccessKey")) {
throw new CredentialException(String.format("Error retrieving credentials from RsaKeyPair result: %s.", httpResponse.getHttpContentString()));
}
Map credentials = (Map) map.get("SessionAccessKey");
Instant expiration = ParameterHelper.getUTCDate(credentials.get("Expiration")).toInstant();
ICredential credential = Credential.builder()
.accessKeyId(credentials.get("SessionAccessKeyId"))
.accessKeySecret(credentials.get("SessionAccessKeySecret"))
.build();
return RefreshResult.builder(credential)
.staleTime(getStaleTime(expiration))
.prefetchTime(getPrefetchTime(expiration))
.build();
}
@Override
public void close() {
super.close();
this.client.close();
}
public interface Builder extends HttpCredentialProvider.Builder {
Builder durationSeconds(Integer durationSeconds);
Builder regionId(String regionId);
Builder connectionTimeout(Integer connectionTimeout);
Builder readTimeout(Integer readTimeout);
Builder credential(Credential credential);
Builder stsEndpoint(String stsEndpoint);
Builder stsRegionId(String stsRegionId);
Builder enableVpc(Boolean enableVpc);
@Override
RsaKeyPairCredentialProvider build();
}
private static final class BuilderImpl
extends HttpCredentialProvider.BuilderImpl
implements Builder {
private Integer durationSeconds;
private String regionId = "cn-hangzhou";
/**
* Unit of millisecond
*/
private Integer connectionTimeout;
private Integer readTimeout;
private Credential credential;
private String stsEndpoint;
private String stsRegionId;
private Boolean enableVpc;
public Builder durationSeconds(Integer durationSeconds) {
if (!StringUtils.isEmpty(durationSeconds)) {
this.durationSeconds = durationSeconds;
}
return this;
}
public Builder regionId(String regionId) {
if (!StringUtils.isEmpty(regionId)) {
this.regionId = regionId;
}
return this;
}
public Builder connectionTimeout(Integer connectionTimeout) {
if (!StringUtils.isEmpty(connectionTimeout)) {
this.connectionTimeout = connectionTimeout;
}
return this;
}
public Builder readTimeout(Integer readTimeout) {
if (!StringUtils.isEmpty(readTimeout)) {
this.readTimeout = readTimeout;
}
return this;
}
public Builder credential(Credential credential) {
this.credential = credential;
return this;
}
public Builder stsEndpoint(String stsEndpoint) {
this.stsEndpoint = stsEndpoint;
return this;
}
public Builder stsRegionId(String stsRegionId) {
this.stsRegionId = stsRegionId;
return this;
}
public Builder enableVpc(Boolean enableVpc) {
this.enableVpc = enableVpc;
return this;
}
@Override
public RsaKeyPairCredentialProvider build() {
return new RsaKeyPairCredentialProvider(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy