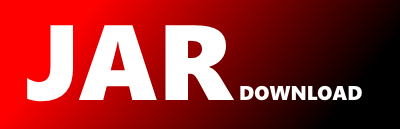
com.aliyun.auth.credentials.provider.URLCredentialProvider Maven / Gradle / Ivy
The newest version!
package com.aliyun.auth.credentials.provider;
import com.aliyun.auth.credentials.Credential;
import com.aliyun.auth.credentials.ICredential;
import com.aliyun.auth.credentials.exception.CredentialException;
import com.aliyun.auth.credentials.http.CompatibleUrlConnClient;
import com.aliyun.auth.credentials.http.HttpRequest;
import com.aliyun.auth.credentials.http.HttpResponse;
import com.aliyun.auth.credentials.http.MethodType;
import com.aliyun.auth.credentials.utils.AuthUtils;
import com.aliyun.auth.credentials.utils.ParameterHelper;
import com.aliyun.auth.credentials.utils.RefreshResult;
import com.aliyun.core.utils.StringUtils;
import com.google.gson.Gson;
import java.net.MalformedURLException;
import java.net.URL;
import java.time.Instant;
import java.util.Map;
public class URLCredentialProvider extends HttpCredentialProvider {
private final URL credentialsURI;
/**
* Unit of millisecond
*/
private int connectTimeout;
private int readTimeout;
private final CompatibleUrlConnClient client;
private URLCredentialProvider(BuilderImpl builder) {
super(builder);
String credentialsURI = builder.credentialsURI == null ? AuthUtils.getEnvironmentCredentialsURI() : builder.credentialsURI;
if (StringUtils.isEmpty(credentialsURI)) {
throw new IllegalArgumentException("Credential URI or environment variable ALIBABA_CLOUD_CREDENTIALS_URI cannot be empty.");
}
try {
this.credentialsURI = new URL(credentialsURI);
} catch (MalformedURLException e) {
throw new IllegalArgumentException("Credential URI is not valid.");
}
this.connectTimeout = builder.connectionTimeout == null ? 5000 : builder.connectionTimeout;
this.readTimeout = builder.readTimeout == null ? 10000 : builder.readTimeout;
this.client = new CompatibleUrlConnClient();
this.buildRefreshCache();
}
public static Builder builder() {
return new BuilderImpl();
}
@Override
public RefreshResult refreshCredentials() {
HttpRequest request = new HttpRequest(this.credentialsURI.toString());
request.setSysMethod(MethodType.GET);
request.setSysConnectTimeout(connectTimeout);
request.setSysReadTimeout(readTimeout);
HttpResponse response;
try {
response = client.syncInvoke(request);
} catch (Exception e) {
throw new CredentialException("Failed to connect Server: " + e.toString(), e);
} finally {
client.close();
}
if (response.getResponseCode() >= 300 || response.getResponseCode() < 200) {
throw new CredentialException("Failed to get credentials from server: " + this.credentialsURI.toString()
+ "\nHttpCode=" + response.getResponseCode()
+ "\nHttpRAWContent=" + response.getHttpContentString());
}
Gson gson = new Gson();
Map map;
try {
map = gson.fromJson(response.getHttpContentString(), Map.class);
} catch (Exception e) {
throw new CredentialException("Failed to get credentials from server: " + this.credentialsURI.toString()
+ "\nHttpCode=" + response.getResponseCode()
+ "\nHttpRAWContent=" + response.getHttpContentString(), e);
}
if (map.containsKey("Code") && map.get("Code").equals("Success")) {
Instant expiration = ParameterHelper.getUTCDate(map.get("Expiration")).toInstant();
ICredential credential = Credential.builder()
.accessKeyId(map.get("AccessKeyId"))
.accessKeySecret(map.get("AccessKeySecret"))
.securityToken(map.get("SecurityToken"))
.build();
return RefreshResult.builder(credential)
.staleTime(getStaleTime(expiration))
.prefetchTime(getPrefetchTime(expiration))
.build();
} else {
throw new CredentialException(String.format("Error retrieving credentials from credentialsURI result: %s.", response.getHttpContentString()));
}
}
@Override
public void close() {
super.close();
this.client.close();
}
public interface Builder extends HttpCredentialProvider.Builder {
Builder credentialsURI(URL credentialsURI);
Builder credentialsURI(String credentialsURI);
Builder connectionTimeout(Integer connectionTimeout);
Builder readTimeout(Integer readTimeout);
@Override
URLCredentialProvider build();
}
private static final class BuilderImpl
extends HttpCredentialProvider.BuilderImpl
implements Builder {
private String credentialsURI;
private Integer connectionTimeout;
private Integer readTimeout;
public Builder credentialsURI(URL credentialsURI) {
this.credentialsURI = credentialsURI.toString();
return this;
}
public Builder credentialsURI(String credentialsURI) {
this.credentialsURI = credentialsURI;
return this;
}
public Builder connectionTimeout(Integer connectionTimeout) {
this.connectionTimeout = connectionTimeout;
return this;
}
public Builder readTimeout(Integer readTimeout) {
this.readTimeout = readTimeout;
return this;
}
@Override
public URLCredentialProvider build() {
return new URLCredentialProvider(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy