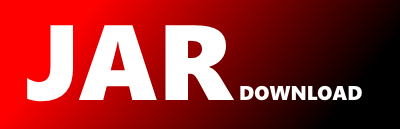
com.aliyuncs.aegis.model.v20161111.DescribeScreenSecurityStatInfoResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aliyun-java-sdk-aegis Show documentation
Show all versions of aliyun-java-sdk-aegis Show documentation
Aliyun Open API SDK for Java
Copyright (C) Alibaba Cloud Computing
All rights reserved.
版权所有 (C)阿里云计算有限公司
http://www.aliyun.com
The newest version!
/*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.aliyuncs.aegis.model.v20161111;
import java.util.List;
import com.aliyuncs.AcsResponse;
import com.aliyuncs.aegis.transform.v20161111.DescribeScreenSecurityStatInfoResponseUnmarshaller;
import com.aliyuncs.transform.UnmarshallerContext;
/**
* @author auto create
* @version
*/
public class DescribeScreenSecurityStatInfoResponse extends AcsResponse {
private String requestId;
private Boolean success;
private SecurityEvent securityEvent;
private AttackEvent attackEvent;
private HealthCheck healthCheck;
private Vulnerability vulnerability;
public String getRequestId() {
return this.requestId;
}
public void setRequestId(String requestId) {
this.requestId = requestId;
}
public Boolean getSuccess() {
return this.success;
}
public void setSuccess(Boolean success) {
this.success = success;
}
public SecurityEvent getSecurityEvent() {
return this.securityEvent;
}
public void setSecurityEvent(SecurityEvent securityEvent) {
this.securityEvent = securityEvent;
}
public AttackEvent getAttackEvent() {
return this.attackEvent;
}
public void setAttackEvent(AttackEvent attackEvent) {
this.attackEvent = attackEvent;
}
public HealthCheck getHealthCheck() {
return this.healthCheck;
}
public void setHealthCheck(HealthCheck healthCheck) {
this.healthCheck = healthCheck;
}
public Vulnerability getVulnerability() {
return this.vulnerability;
}
public void setVulnerability(Vulnerability vulnerability) {
this.vulnerability = vulnerability;
}
public static class SecurityEvent {
private Integer seriousCount;
private Integer suspiciousCount;
private Integer remindCount;
private Integer totalCount;
private List dateArray;
private List valueArray;
private List levelsOn;
private List seriousList;
private List suspiciousList;
private List remindList;
public Integer getSeriousCount() {
return this.seriousCount;
}
public void setSeriousCount(Integer seriousCount) {
this.seriousCount = seriousCount;
}
public Integer getSuspiciousCount() {
return this.suspiciousCount;
}
public void setSuspiciousCount(Integer suspiciousCount) {
this.suspiciousCount = suspiciousCount;
}
public Integer getRemindCount() {
return this.remindCount;
}
public void setRemindCount(Integer remindCount) {
this.remindCount = remindCount;
}
public Integer getTotalCount() {
return this.totalCount;
}
public void setTotalCount(Integer totalCount) {
this.totalCount = totalCount;
}
public List getDateArray() {
return this.dateArray;
}
public void setDateArray(List dateArray) {
this.dateArray = dateArray;
}
public List getValueArray() {
return this.valueArray;
}
public void setValueArray(List valueArray) {
this.valueArray = valueArray;
}
public List getLevelsOn() {
return this.levelsOn;
}
public void setLevelsOn(List levelsOn) {
this.levelsOn = levelsOn;
}
public List getSeriousList() {
return this.seriousList;
}
public void setSeriousList(List seriousList) {
this.seriousList = seriousList;
}
public List getSuspiciousList() {
return this.suspiciousList;
}
public void setSuspiciousList(List suspiciousList) {
this.suspiciousList = suspiciousList;
}
public List getRemindList() {
return this.remindList;
}
public void setRemindList(List remindList) {
this.remindList = remindList;
}
}
public static class AttackEvent {
private Integer totalCount;
private List dateArray1;
private List valueArray2;
public Integer getTotalCount() {
return this.totalCount;
}
public void setTotalCount(Integer totalCount) {
this.totalCount = totalCount;
}
public List getDateArray1() {
return this.dateArray1;
}
public void setDateArray1(List dateArray1) {
this.dateArray1 = dateArray1;
}
public List getValueArray2() {
return this.valueArray2;
}
public void setValueArray2(List valueArray2) {
this.valueArray2 = valueArray2;
}
}
public static class HealthCheck {
private Integer mediumCount;
private Integer highCount;
private Integer lowCount;
private Integer totalCount;
private List dateArray3;
private List valueArray4;
private List levelsOn5;
private List highList;
private List mediumList;
private List lowList;
public Integer getMediumCount() {
return this.mediumCount;
}
public void setMediumCount(Integer mediumCount) {
this.mediumCount = mediumCount;
}
public Integer getHighCount() {
return this.highCount;
}
public void setHighCount(Integer highCount) {
this.highCount = highCount;
}
public Integer getLowCount() {
return this.lowCount;
}
public void setLowCount(Integer lowCount) {
this.lowCount = lowCount;
}
public Integer getTotalCount() {
return this.totalCount;
}
public void setTotalCount(Integer totalCount) {
this.totalCount = totalCount;
}
public List getDateArray3() {
return this.dateArray3;
}
public void setDateArray3(List dateArray3) {
this.dateArray3 = dateArray3;
}
public List getValueArray4() {
return this.valueArray4;
}
public void setValueArray4(List valueArray4) {
this.valueArray4 = valueArray4;
}
public List getLevelsOn5() {
return this.levelsOn5;
}
public void setLevelsOn5(List levelsOn5) {
this.levelsOn5 = levelsOn5;
}
public List getHighList() {
return this.highList;
}
public void setHighList(List highList) {
this.highList = highList;
}
public List getMediumList() {
return this.mediumList;
}
public void setMediumList(List mediumList) {
this.mediumList = mediumList;
}
public List getLowList() {
return this.lowList;
}
public void setLowList(List lowList) {
this.lowList = lowList;
}
}
public static class Vulnerability {
private Integer nntfCount;
private Integer laterCount;
private Integer asapCount;
private Integer totalCount;
private List dateArray6;
private List valueArray7;
private List levelsOn8;
private List nntfList;
private List asapList;
private List laterList;
public Integer getNntfCount() {
return this.nntfCount;
}
public void setNntfCount(Integer nntfCount) {
this.nntfCount = nntfCount;
}
public Integer getLaterCount() {
return this.laterCount;
}
public void setLaterCount(Integer laterCount) {
this.laterCount = laterCount;
}
public Integer getAsapCount() {
return this.asapCount;
}
public void setAsapCount(Integer asapCount) {
this.asapCount = asapCount;
}
public Integer getTotalCount() {
return this.totalCount;
}
public void setTotalCount(Integer totalCount) {
this.totalCount = totalCount;
}
public List getDateArray6() {
return this.dateArray6;
}
public void setDateArray6(List dateArray6) {
this.dateArray6 = dateArray6;
}
public List getValueArray7() {
return this.valueArray7;
}
public void setValueArray7(List valueArray7) {
this.valueArray7 = valueArray7;
}
public List getLevelsOn8() {
return this.levelsOn8;
}
public void setLevelsOn8(List levelsOn8) {
this.levelsOn8 = levelsOn8;
}
public List getNntfList() {
return this.nntfList;
}
public void setNntfList(List nntfList) {
this.nntfList = nntfList;
}
public List getAsapList() {
return this.asapList;
}
public void setAsapList(List asapList) {
this.asapList = asapList;
}
public List getLaterList() {
return this.laterList;
}
public void setLaterList(List laterList) {
this.laterList = laterList;
}
}
@Override
public DescribeScreenSecurityStatInfoResponse getInstance(UnmarshallerContext context) {
return DescribeScreenSecurityStatInfoResponseUnmarshaller.unmarshall(this, context);
}
@Override
public boolean checkShowJsonItemName() {
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy