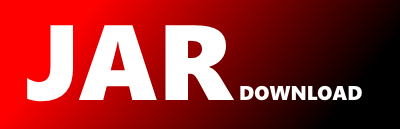
com.aliyuncs.cbn.model.v20170912.CreateCenRouteMapRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aliyun-java-sdk-cbn Show documentation
Show all versions of aliyun-java-sdk-cbn Show documentation
Aliyun Open API SDK for Java
Copyright (C) Alibaba Cloud Computing
All rights reserved.
版权所有 (C)阿里云计算有限公司
http://www.aliyun.com
/*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.aliyuncs.cbn.model.v20170912;
import com.aliyuncs.RpcAcsRequest;
import java.util.List;
import com.aliyuncs.http.MethodType;
import com.aliyuncs.cbn.Endpoint;
/**
* @author auto create
* @version
*/
public class CreateCenRouteMapRequest extends RpcAcsRequest {
private Long resourceOwnerId;
private String communityMatchMode;
private String mapResult;
private Integer nextPriority;
private List destinationCidrBlockss;
private String transitRouterRouteTableId;
private List sourceInstanceIdss;
private List sourceRegionIdss;
private List matchAsnss;
private Integer preference;
private Long ownerId;
private Integer priority;
private List destinationChildInstanceTypess;
private List sourceRouteTableIdss;
private List sourceChildInstanceTypess;
private String communityOperateMode;
private List operateCommunitySets;
private List routeTypess;
private String matchAddressType;
private String cidrMatchMode;
private String cenId;
private String description;
private Boolean sourceInstanceIdsReverseMatch;
private List destinationRouteTableIdss;
private String transmitDirection;
private List destinationInstanceIdss;
private String resourceOwnerAccount;
private String ownerAccount;
private Boolean destinationInstanceIdsReverseMatch;
private List prependAsPaths;
private String asPathMatchMode;
private List matchCommunitySets;
private String cenRegionId;
public CreateCenRouteMapRequest() {
super("Cbn", "2017-09-12", "CreateCenRouteMap");
setMethod(MethodType.POST);
try {
com.aliyuncs.AcsRequest.class.getDeclaredField("productEndpointMap").set(this, Endpoint.endpointMap);
com.aliyuncs.AcsRequest.class.getDeclaredField("productEndpointRegional").set(this, Endpoint.endpointRegionalType);
} catch (Exception e) {}
}
public Long getResourceOwnerId() {
return this.resourceOwnerId;
}
public void setResourceOwnerId(Long resourceOwnerId) {
this.resourceOwnerId = resourceOwnerId;
if(resourceOwnerId != null){
putQueryParameter("ResourceOwnerId", resourceOwnerId.toString());
}
}
public String getCommunityMatchMode() {
return this.communityMatchMode;
}
public void setCommunityMatchMode(String communityMatchMode) {
this.communityMatchMode = communityMatchMode;
if(communityMatchMode != null){
putQueryParameter("CommunityMatchMode", communityMatchMode);
}
}
public String getMapResult() {
return this.mapResult;
}
public void setMapResult(String mapResult) {
this.mapResult = mapResult;
if(mapResult != null){
putQueryParameter("MapResult", mapResult);
}
}
public Integer getNextPriority() {
return this.nextPriority;
}
public void setNextPriority(Integer nextPriority) {
this.nextPriority = nextPriority;
if(nextPriority != null){
putQueryParameter("NextPriority", nextPriority.toString());
}
}
public List getDestinationCidrBlockss() {
return this.destinationCidrBlockss;
}
public void setDestinationCidrBlockss(List destinationCidrBlockss) {
this.destinationCidrBlockss = destinationCidrBlockss;
if (destinationCidrBlockss != null) {
for (int i = 0; i < destinationCidrBlockss.size(); i++) {
putQueryParameter("DestinationCidrBlocks." + (i + 1) , destinationCidrBlockss.get(i));
}
}
}
public String getTransitRouterRouteTableId() {
return this.transitRouterRouteTableId;
}
public void setTransitRouterRouteTableId(String transitRouterRouteTableId) {
this.transitRouterRouteTableId = transitRouterRouteTableId;
if(transitRouterRouteTableId != null){
putQueryParameter("TransitRouterRouteTableId", transitRouterRouteTableId);
}
}
public List getSourceInstanceIdss() {
return this.sourceInstanceIdss;
}
public void setSourceInstanceIdss(List sourceInstanceIdss) {
this.sourceInstanceIdss = sourceInstanceIdss;
if (sourceInstanceIdss != null) {
for (int i = 0; i < sourceInstanceIdss.size(); i++) {
putQueryParameter("SourceInstanceIds." + (i + 1) , sourceInstanceIdss.get(i));
}
}
}
public List getSourceRegionIdss() {
return this.sourceRegionIdss;
}
public void setSourceRegionIdss(List sourceRegionIdss) {
this.sourceRegionIdss = sourceRegionIdss;
if (sourceRegionIdss != null) {
for (int i = 0; i < sourceRegionIdss.size(); i++) {
putQueryParameter("SourceRegionIds." + (i + 1) , sourceRegionIdss.get(i));
}
}
}
public List getMatchAsnss() {
return this.matchAsnss;
}
public void setMatchAsnss(List matchAsnss) {
this.matchAsnss = matchAsnss;
if (matchAsnss != null) {
for (int i = 0; i < matchAsnss.size(); i++) {
putQueryParameter("MatchAsns." + (i + 1) , matchAsnss.get(i));
}
}
}
public Integer getPreference() {
return this.preference;
}
public void setPreference(Integer preference) {
this.preference = preference;
if(preference != null){
putQueryParameter("Preference", preference.toString());
}
}
public Long getOwnerId() {
return this.ownerId;
}
public void setOwnerId(Long ownerId) {
this.ownerId = ownerId;
if(ownerId != null){
putQueryParameter("OwnerId", ownerId.toString());
}
}
public Integer getPriority() {
return this.priority;
}
public void setPriority(Integer priority) {
this.priority = priority;
if(priority != null){
putQueryParameter("Priority", priority.toString());
}
}
public List getDestinationChildInstanceTypess() {
return this.destinationChildInstanceTypess;
}
public void setDestinationChildInstanceTypess(List destinationChildInstanceTypess) {
this.destinationChildInstanceTypess = destinationChildInstanceTypess;
if (destinationChildInstanceTypess != null) {
for (int i = 0; i < destinationChildInstanceTypess.size(); i++) {
putQueryParameter("DestinationChildInstanceTypes." + (i + 1) , destinationChildInstanceTypess.get(i));
}
}
}
public List getSourceRouteTableIdss() {
return this.sourceRouteTableIdss;
}
public void setSourceRouteTableIdss(List sourceRouteTableIdss) {
this.sourceRouteTableIdss = sourceRouteTableIdss;
if (sourceRouteTableIdss != null) {
for (int i = 0; i < sourceRouteTableIdss.size(); i++) {
putQueryParameter("SourceRouteTableIds." + (i + 1) , sourceRouteTableIdss.get(i));
}
}
}
public List getSourceChildInstanceTypess() {
return this.sourceChildInstanceTypess;
}
public void setSourceChildInstanceTypess(List sourceChildInstanceTypess) {
this.sourceChildInstanceTypess = sourceChildInstanceTypess;
if (sourceChildInstanceTypess != null) {
for (int i = 0; i < sourceChildInstanceTypess.size(); i++) {
putQueryParameter("SourceChildInstanceTypes." + (i + 1) , sourceChildInstanceTypess.get(i));
}
}
}
public String getCommunityOperateMode() {
return this.communityOperateMode;
}
public void setCommunityOperateMode(String communityOperateMode) {
this.communityOperateMode = communityOperateMode;
if(communityOperateMode != null){
putQueryParameter("CommunityOperateMode", communityOperateMode);
}
}
public List getOperateCommunitySets() {
return this.operateCommunitySets;
}
public void setOperateCommunitySets(List operateCommunitySets) {
this.operateCommunitySets = operateCommunitySets;
if (operateCommunitySets != null) {
for (int i = 0; i < operateCommunitySets.size(); i++) {
putQueryParameter("OperateCommunitySet." + (i + 1) , operateCommunitySets.get(i));
}
}
}
public List getRouteTypess() {
return this.routeTypess;
}
public void setRouteTypess(List routeTypess) {
this.routeTypess = routeTypess;
if (routeTypess != null) {
for (int i = 0; i < routeTypess.size(); i++) {
putQueryParameter("RouteTypes." + (i + 1) , routeTypess.get(i));
}
}
}
public String getMatchAddressType() {
return this.matchAddressType;
}
public void setMatchAddressType(String matchAddressType) {
this.matchAddressType = matchAddressType;
if(matchAddressType != null){
putQueryParameter("MatchAddressType", matchAddressType);
}
}
public String getCidrMatchMode() {
return this.cidrMatchMode;
}
public void setCidrMatchMode(String cidrMatchMode) {
this.cidrMatchMode = cidrMatchMode;
if(cidrMatchMode != null){
putQueryParameter("CidrMatchMode", cidrMatchMode);
}
}
public String getCenId() {
return this.cenId;
}
public void setCenId(String cenId) {
this.cenId = cenId;
if(cenId != null){
putQueryParameter("CenId", cenId);
}
}
public String getDescription() {
return this.description;
}
public void setDescription(String description) {
this.description = description;
if(description != null){
putQueryParameter("Description", description);
}
}
public Boolean getSourceInstanceIdsReverseMatch() {
return this.sourceInstanceIdsReverseMatch;
}
public void setSourceInstanceIdsReverseMatch(Boolean sourceInstanceIdsReverseMatch) {
this.sourceInstanceIdsReverseMatch = sourceInstanceIdsReverseMatch;
if(sourceInstanceIdsReverseMatch != null){
putQueryParameter("SourceInstanceIdsReverseMatch", sourceInstanceIdsReverseMatch.toString());
}
}
public List getDestinationRouteTableIdss() {
return this.destinationRouteTableIdss;
}
public void setDestinationRouteTableIdss(List destinationRouteTableIdss) {
this.destinationRouteTableIdss = destinationRouteTableIdss;
if (destinationRouteTableIdss != null) {
for (int i = 0; i < destinationRouteTableIdss.size(); i++) {
putQueryParameter("DestinationRouteTableIds." + (i + 1) , destinationRouteTableIdss.get(i));
}
}
}
public String getTransmitDirection() {
return this.transmitDirection;
}
public void setTransmitDirection(String transmitDirection) {
this.transmitDirection = transmitDirection;
if(transmitDirection != null){
putQueryParameter("TransmitDirection", transmitDirection);
}
}
public List getDestinationInstanceIdss() {
return this.destinationInstanceIdss;
}
public void setDestinationInstanceIdss(List destinationInstanceIdss) {
this.destinationInstanceIdss = destinationInstanceIdss;
if (destinationInstanceIdss != null) {
for (int i = 0; i < destinationInstanceIdss.size(); i++) {
putQueryParameter("DestinationInstanceIds." + (i + 1) , destinationInstanceIdss.get(i));
}
}
}
public String getResourceOwnerAccount() {
return this.resourceOwnerAccount;
}
public void setResourceOwnerAccount(String resourceOwnerAccount) {
this.resourceOwnerAccount = resourceOwnerAccount;
if(resourceOwnerAccount != null){
putQueryParameter("ResourceOwnerAccount", resourceOwnerAccount);
}
}
public String getOwnerAccount() {
return this.ownerAccount;
}
public void setOwnerAccount(String ownerAccount) {
this.ownerAccount = ownerAccount;
if(ownerAccount != null){
putQueryParameter("OwnerAccount", ownerAccount);
}
}
public Boolean getDestinationInstanceIdsReverseMatch() {
return this.destinationInstanceIdsReverseMatch;
}
public void setDestinationInstanceIdsReverseMatch(Boolean destinationInstanceIdsReverseMatch) {
this.destinationInstanceIdsReverseMatch = destinationInstanceIdsReverseMatch;
if(destinationInstanceIdsReverseMatch != null){
putQueryParameter("DestinationInstanceIdsReverseMatch", destinationInstanceIdsReverseMatch.toString());
}
}
public List getPrependAsPaths() {
return this.prependAsPaths;
}
public void setPrependAsPaths(List prependAsPaths) {
this.prependAsPaths = prependAsPaths;
if (prependAsPaths != null) {
for (int i = 0; i < prependAsPaths.size(); i++) {
putQueryParameter("PrependAsPath." + (i + 1) , prependAsPaths.get(i));
}
}
}
public String getAsPathMatchMode() {
return this.asPathMatchMode;
}
public void setAsPathMatchMode(String asPathMatchMode) {
this.asPathMatchMode = asPathMatchMode;
if(asPathMatchMode != null){
putQueryParameter("AsPathMatchMode", asPathMatchMode);
}
}
public List getMatchCommunitySets() {
return this.matchCommunitySets;
}
public void setMatchCommunitySets(List matchCommunitySets) {
this.matchCommunitySets = matchCommunitySets;
if (matchCommunitySets != null) {
for (int i = 0; i < matchCommunitySets.size(); i++) {
putQueryParameter("MatchCommunitySet." + (i + 1) , matchCommunitySets.get(i));
}
}
}
public String getCenRegionId() {
return this.cenRegionId;
}
public void setCenRegionId(String cenRegionId) {
this.cenRegionId = cenRegionId;
if(cenRegionId != null){
putQueryParameter("CenRegionId", cenRegionId);
}
}
@Override
public Class getResponseClass() {
return CreateCenRouteMapResponse.class;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy