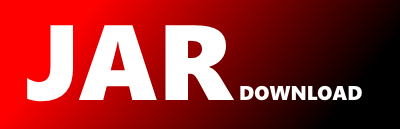
com.aliyuncs.cbn.model.v20170912.DescribeCenRouteMapsResponse Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.aliyuncs.cbn.model.v20170912;
import java.util.List;
import com.aliyuncs.AcsResponse;
import com.aliyuncs.cbn.transform.v20170912.DescribeCenRouteMapsResponseUnmarshaller;
import com.aliyuncs.transform.UnmarshallerContext;
/**
* @author auto create
* @version
*/
public class DescribeCenRouteMapsResponse extends AcsResponse {
private Integer pageSize;
private String requestId;
private Integer pageNumber;
private Integer totalCount;
private List routeMaps;
public Integer getPageSize() {
return this.pageSize;
}
public void setPageSize(Integer pageSize) {
this.pageSize = pageSize;
}
public String getRequestId() {
return this.requestId;
}
public void setRequestId(String requestId) {
this.requestId = requestId;
}
public Integer getPageNumber() {
return this.pageNumber;
}
public void setPageNumber(Integer pageNumber) {
this.pageNumber = pageNumber;
}
public Integer getTotalCount() {
return this.totalCount;
}
public void setTotalCount(Integer totalCount) {
this.totalCount = totalCount;
}
public List getRouteMaps() {
return this.routeMaps;
}
public void setRouteMaps(List routeMaps) {
this.routeMaps = routeMaps;
}
public static class RouteMap {
private String routeMapId;
private String status;
private String transmitDirection;
private Boolean sourceInstanceIdsReverseMatch;
private String cenRegionId;
private String cenId;
private Integer priority;
private String transitRouterRouteTableId;
private String communityOperateMode;
private String mapResult;
private String communityMatchMode;
private String description;
private String asPathMatchMode;
private Integer preference;
private Boolean destinationInstanceIdsReverseMatch;
private String cidrMatchMode;
private Integer nextPriority;
private String matchAddressType;
private String gatewayZoneId;
private String gatewayRegionId;
private List sourceRegionIds;
private List sourceChildInstanceTypes;
private List destinationRouteTableIds;
private List sourceInstanceIds;
private List destinationCidrBlocks;
private List destinationRegionIds;
private List sourceRouteTableIds;
private List matchCommunitySet;
private List prependAsPath;
private List routeTypes;
private List originalRouteTableIds;
private List destinationChildInstanceTypes;
private List destinationInstanceIds;
private List matchAsns;
private List operateCommunitySet;
private List srcZoneIds;
public String getRouteMapId() {
return this.routeMapId;
}
public void setRouteMapId(String routeMapId) {
this.routeMapId = routeMapId;
}
public String getStatus() {
return this.status;
}
public void setStatus(String status) {
this.status = status;
}
public String getTransmitDirection() {
return this.transmitDirection;
}
public void setTransmitDirection(String transmitDirection) {
this.transmitDirection = transmitDirection;
}
public Boolean getSourceInstanceIdsReverseMatch() {
return this.sourceInstanceIdsReverseMatch;
}
public void setSourceInstanceIdsReverseMatch(Boolean sourceInstanceIdsReverseMatch) {
this.sourceInstanceIdsReverseMatch = sourceInstanceIdsReverseMatch;
}
public String getCenRegionId() {
return this.cenRegionId;
}
public void setCenRegionId(String cenRegionId) {
this.cenRegionId = cenRegionId;
}
public String getCenId() {
return this.cenId;
}
public void setCenId(String cenId) {
this.cenId = cenId;
}
public Integer getPriority() {
return this.priority;
}
public void setPriority(Integer priority) {
this.priority = priority;
}
public String getTransitRouterRouteTableId() {
return this.transitRouterRouteTableId;
}
public void setTransitRouterRouteTableId(String transitRouterRouteTableId) {
this.transitRouterRouteTableId = transitRouterRouteTableId;
}
public String getCommunityOperateMode() {
return this.communityOperateMode;
}
public void setCommunityOperateMode(String communityOperateMode) {
this.communityOperateMode = communityOperateMode;
}
public String getMapResult() {
return this.mapResult;
}
public void setMapResult(String mapResult) {
this.mapResult = mapResult;
}
public String getCommunityMatchMode() {
return this.communityMatchMode;
}
public void setCommunityMatchMode(String communityMatchMode) {
this.communityMatchMode = communityMatchMode;
}
public String getDescription() {
return this.description;
}
public void setDescription(String description) {
this.description = description;
}
public String getAsPathMatchMode() {
return this.asPathMatchMode;
}
public void setAsPathMatchMode(String asPathMatchMode) {
this.asPathMatchMode = asPathMatchMode;
}
public Integer getPreference() {
return this.preference;
}
public void setPreference(Integer preference) {
this.preference = preference;
}
public Boolean getDestinationInstanceIdsReverseMatch() {
return this.destinationInstanceIdsReverseMatch;
}
public void setDestinationInstanceIdsReverseMatch(Boolean destinationInstanceIdsReverseMatch) {
this.destinationInstanceIdsReverseMatch = destinationInstanceIdsReverseMatch;
}
public String getCidrMatchMode() {
return this.cidrMatchMode;
}
public void setCidrMatchMode(String cidrMatchMode) {
this.cidrMatchMode = cidrMatchMode;
}
public Integer getNextPriority() {
return this.nextPriority;
}
public void setNextPriority(Integer nextPriority) {
this.nextPriority = nextPriority;
}
public String getMatchAddressType() {
return this.matchAddressType;
}
public void setMatchAddressType(String matchAddressType) {
this.matchAddressType = matchAddressType;
}
public String getGatewayZoneId() {
return this.gatewayZoneId;
}
public void setGatewayZoneId(String gatewayZoneId) {
this.gatewayZoneId = gatewayZoneId;
}
public String getGatewayRegionId() {
return this.gatewayRegionId;
}
public void setGatewayRegionId(String gatewayRegionId) {
this.gatewayRegionId = gatewayRegionId;
}
public List getSourceRegionIds() {
return this.sourceRegionIds;
}
public void setSourceRegionIds(List sourceRegionIds) {
this.sourceRegionIds = sourceRegionIds;
}
public List getSourceChildInstanceTypes() {
return this.sourceChildInstanceTypes;
}
public void setSourceChildInstanceTypes(List sourceChildInstanceTypes) {
this.sourceChildInstanceTypes = sourceChildInstanceTypes;
}
public List getDestinationRouteTableIds() {
return this.destinationRouteTableIds;
}
public void setDestinationRouteTableIds(List destinationRouteTableIds) {
this.destinationRouteTableIds = destinationRouteTableIds;
}
public List getSourceInstanceIds() {
return this.sourceInstanceIds;
}
public void setSourceInstanceIds(List sourceInstanceIds) {
this.sourceInstanceIds = sourceInstanceIds;
}
public List getDestinationCidrBlocks() {
return this.destinationCidrBlocks;
}
public void setDestinationCidrBlocks(List destinationCidrBlocks) {
this.destinationCidrBlocks = destinationCidrBlocks;
}
public List getDestinationRegionIds() {
return this.destinationRegionIds;
}
public void setDestinationRegionIds(List destinationRegionIds) {
this.destinationRegionIds = destinationRegionIds;
}
public List getSourceRouteTableIds() {
return this.sourceRouteTableIds;
}
public void setSourceRouteTableIds(List sourceRouteTableIds) {
this.sourceRouteTableIds = sourceRouteTableIds;
}
public List getMatchCommunitySet() {
return this.matchCommunitySet;
}
public void setMatchCommunitySet(List matchCommunitySet) {
this.matchCommunitySet = matchCommunitySet;
}
public List getPrependAsPath() {
return this.prependAsPath;
}
public void setPrependAsPath(List prependAsPath) {
this.prependAsPath = prependAsPath;
}
public List getRouteTypes() {
return this.routeTypes;
}
public void setRouteTypes(List routeTypes) {
this.routeTypes = routeTypes;
}
public List getOriginalRouteTableIds() {
return this.originalRouteTableIds;
}
public void setOriginalRouteTableIds(List originalRouteTableIds) {
this.originalRouteTableIds = originalRouteTableIds;
}
public List getDestinationChildInstanceTypes() {
return this.destinationChildInstanceTypes;
}
public void setDestinationChildInstanceTypes(List destinationChildInstanceTypes) {
this.destinationChildInstanceTypes = destinationChildInstanceTypes;
}
public List getDestinationInstanceIds() {
return this.destinationInstanceIds;
}
public void setDestinationInstanceIds(List destinationInstanceIds) {
this.destinationInstanceIds = destinationInstanceIds;
}
public List getMatchAsns() {
return this.matchAsns;
}
public void setMatchAsns(List matchAsns) {
this.matchAsns = matchAsns;
}
public List getOperateCommunitySet() {
return this.operateCommunitySet;
}
public void setOperateCommunitySet(List operateCommunitySet) {
this.operateCommunitySet = operateCommunitySet;
}
public List getSrcZoneIds() {
return this.srcZoneIds;
}
public void setSrcZoneIds(List srcZoneIds) {
this.srcZoneIds = srcZoneIds;
}
}
@Override
public DescribeCenRouteMapsResponse getInstance(UnmarshallerContext context) {
return DescribeCenRouteMapsResponseUnmarshaller.unmarshall(this, context);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy