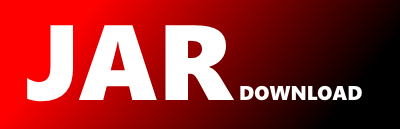
com.aliyuncs.clouddesktop.model.v20170301.DescribeDesktopsResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aliyun-java-sdk-clouddesktop Show documentation
Show all versions of aliyun-java-sdk-clouddesktop Show documentation
Aliyun Open API SDK for Java
Copyright (C) Alibaba Cloud Computing
All rights reserved.
版权所有 (C)阿里云计算有限公司
http://www.aliyun.com
The newest version!
/*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.aliyuncs.clouddesktop.model.v20170301;
import java.util.List;
import com.aliyuncs.AcsResponse;
import com.aliyuncs.clouddesktop.transform.v20170301.DescribeDesktopsResponseUnmarshaller;
import com.aliyuncs.transform.UnmarshallerContext;
/**
* @author auto create
* @version
*/
public class DescribeDesktopsResponse extends AcsResponse {
private String requestId;
private Boolean success;
private String message;
private String errorCode;
private Integer total;
private Integer pageSize;
private Integer pageCount;
private Integer page;
private List desktops;
public String getRequestId() {
return this.requestId;
}
public void setRequestId(String requestId) {
this.requestId = requestId;
}
public Boolean getSuccess() {
return this.success;
}
public void setSuccess(Boolean success) {
this.success = success;
}
public String getMessage() {
return this.message;
}
public void setMessage(String message) {
this.message = message;
}
public String getErrorCode() {
return this.errorCode;
}
public void setErrorCode(String errorCode) {
this.errorCode = errorCode;
}
public Integer getTotal() {
return this.total;
}
public void setTotal(Integer total) {
this.total = total;
}
public Integer getPageSize() {
return this.pageSize;
}
public void setPageSize(Integer pageSize) {
this.pageSize = pageSize;
}
public Integer getPageCount() {
return this.pageCount;
}
public void setPageCount(Integer pageCount) {
this.pageCount = pageCount;
}
public Integer getPage() {
return this.page;
}
public void setPage(Integer page) {
this.page = page;
}
public List getDesktops() {
return this.desktops;
}
public void setDesktops(List desktops) {
this.desktops = desktops;
}
public static class DesktopAttributes {
private String desktopId;
private String desktopName;
private String userName;
private String regionId;
private String zoneId;
private String status;
private String imageId;
private String imageName;
private String imagePlatform;
private String imageArch;
private String imageSize;
private String desktopType;
private String cpu;
private String memory;
private String eipAddress;
private String eipInstanceId;
private String internetChargeType;
private String internetBandwidth;
private String vpcId;
private String switchId;
private String desktopIpAddress;
private String desktopChargeType;
private String createDate;
private String expiredDate;
private Boolean enable;
private String errorCode;
private String errorMessage;
private String policy;
private String userGroup;
private List diskInfos;
private List securityGroups;
public String getDesktopId() {
return this.desktopId;
}
public void setDesktopId(String desktopId) {
this.desktopId = desktopId;
}
public String getDesktopName() {
return this.desktopName;
}
public void setDesktopName(String desktopName) {
this.desktopName = desktopName;
}
public String getUserName() {
return this.userName;
}
public void setUserName(String userName) {
this.userName = userName;
}
public String getRegionId() {
return this.regionId;
}
public void setRegionId(String regionId) {
this.regionId = regionId;
}
public String getZoneId() {
return this.zoneId;
}
public void setZoneId(String zoneId) {
this.zoneId = zoneId;
}
public String getStatus() {
return this.status;
}
public void setStatus(String status) {
this.status = status;
}
public String getImageId() {
return this.imageId;
}
public void setImageId(String imageId) {
this.imageId = imageId;
}
public String getImageName() {
return this.imageName;
}
public void setImageName(String imageName) {
this.imageName = imageName;
}
public String getImagePlatform() {
return this.imagePlatform;
}
public void setImagePlatform(String imagePlatform) {
this.imagePlatform = imagePlatform;
}
public String getImageArch() {
return this.imageArch;
}
public void setImageArch(String imageArch) {
this.imageArch = imageArch;
}
public String getImageSize() {
return this.imageSize;
}
public void setImageSize(String imageSize) {
this.imageSize = imageSize;
}
public String getDesktopType() {
return this.desktopType;
}
public void setDesktopType(String desktopType) {
this.desktopType = desktopType;
}
public String getCpu() {
return this.cpu;
}
public void setCpu(String cpu) {
this.cpu = cpu;
}
public String getMemory() {
return this.memory;
}
public void setMemory(String memory) {
this.memory = memory;
}
public String getEipAddress() {
return this.eipAddress;
}
public void setEipAddress(String eipAddress) {
this.eipAddress = eipAddress;
}
public String getEipInstanceId() {
return this.eipInstanceId;
}
public void setEipInstanceId(String eipInstanceId) {
this.eipInstanceId = eipInstanceId;
}
public String getInternetChargeType() {
return this.internetChargeType;
}
public void setInternetChargeType(String internetChargeType) {
this.internetChargeType = internetChargeType;
}
public String getInternetBandwidth() {
return this.internetBandwidth;
}
public void setInternetBandwidth(String internetBandwidth) {
this.internetBandwidth = internetBandwidth;
}
public String getVpcId() {
return this.vpcId;
}
public void setVpcId(String vpcId) {
this.vpcId = vpcId;
}
public String getSwitchId() {
return this.switchId;
}
public void setSwitchId(String switchId) {
this.switchId = switchId;
}
public String getDesktopIpAddress() {
return this.desktopIpAddress;
}
public void setDesktopIpAddress(String desktopIpAddress) {
this.desktopIpAddress = desktopIpAddress;
}
public String getDesktopChargeType() {
return this.desktopChargeType;
}
public void setDesktopChargeType(String desktopChargeType) {
this.desktopChargeType = desktopChargeType;
}
public String getCreateDate() {
return this.createDate;
}
public void setCreateDate(String createDate) {
this.createDate = createDate;
}
public String getExpiredDate() {
return this.expiredDate;
}
public void setExpiredDate(String expiredDate) {
this.expiredDate = expiredDate;
}
public Boolean getEnable() {
return this.enable;
}
public void setEnable(Boolean enable) {
this.enable = enable;
}
public String getErrorCode() {
return this.errorCode;
}
public void setErrorCode(String errorCode) {
this.errorCode = errorCode;
}
public String getErrorMessage() {
return this.errorMessage;
}
public void setErrorMessage(String errorMessage) {
this.errorMessage = errorMessage;
}
public String getPolicy() {
return this.policy;
}
public void setPolicy(String policy) {
this.policy = policy;
}
public String getUserGroup() {
return this.userGroup;
}
public void setUserGroup(String userGroup) {
this.userGroup = userGroup;
}
public List getDiskInfos() {
return this.diskInfos;
}
public void setDiskInfos(List diskInfos) {
this.diskInfos = diskInfos;
}
public List getSecurityGroups() {
return this.securityGroups;
}
public void setSecurityGroups(List securityGroups) {
this.securityGroups = securityGroups;
}
public static class DiskInfo {
private String id;
private String category;
private String type;
private Integer size;
public String getId() {
return this.id;
}
public void setId(String id) {
this.id = id;
}
public String getCategory() {
return this.category;
}
public void setCategory(String category) {
this.category = category;
}
public String getType() {
return this.type;
}
public void setType(String type) {
this.type = type;
}
public Integer getSize() {
return this.size;
}
public void setSize(Integer size) {
this.size = size;
}
}
}
@Override
public DescribeDesktopsResponse getInstance(UnmarshallerContext context) {
return DescribeDesktopsResponseUnmarshaller.unmarshall(this, context);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy