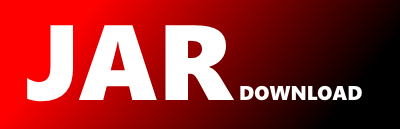
com.aliyuncs.v5.utils.MapUtils Maven / Gradle / Ivy
package com.aliyuncs.v5.utils;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class MapUtils {
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy