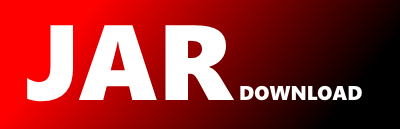
com.aliyuncs.utils.MapUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aliyun-java-sdk-core Show documentation
Show all versions of aliyun-java-sdk-core Show documentation
Aliyun Open API SDK for Java
Copyright (C) Alibaba Cloud Computing
All rights reserved.
版权所有 (C)阿里云计算有限公司
http://www.aliyun.com
The newest version!
package com.aliyuncs.utils;
import java.util.*;
public class MapUtils {
public static String getMapString(Map map) {
if (map == null) {
return "";
}
StringBuilder sb = new StringBuilder("{");
for (Map.Entry entry : map.entrySet()) {
sb.append("\"").append(entry.getKey()).append("\":\"").append(entry.getValue()).append("\"");
}
sb.append("}");
return sb.toString();
}
public Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy