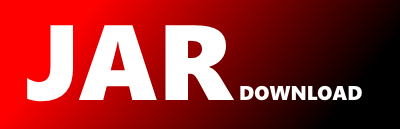
com.aliyuncs.ddoscoo.model.v20200101.DescribeDomainResourceResponse Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.aliyuncs.ddoscoo.model.v20200101;
import java.util.List;
import com.aliyuncs.AcsResponse;
import com.aliyuncs.ddoscoo.transform.v20200101.DescribeDomainResourceResponseUnmarshaller;
import com.aliyuncs.transform.UnmarshallerContext;
/**
* @author auto create
* @version
*/
public class DescribeDomainResourceResponse extends AcsResponse {
private Long totalCount;
private String requestId;
private List webRules;
public Long getTotalCount() {
return this.totalCount;
}
public void setTotalCount(Long totalCount) {
this.totalCount = totalCount;
}
public String getRequestId() {
return this.requestId;
}
public void setRequestId(String requestId) {
this.requestId = requestId;
}
public List getWebRules() {
return this.webRules;
}
public void setWebRules(List webRules) {
this.webRules = webRules;
}
public static class WebRule {
private String domain;
private Boolean http2HttpsEnable;
private String sslProtocols;
private Integer punishReason;
private String ccTemplate;
private String httpsExt;
private Boolean ccEnabled;
private String sslCiphers;
private Boolean ccRuleEnabled;
private Boolean ssl13Enabled;
private Integer rsType;
private Boolean punishStatus;
private Boolean proxyEnabled;
private String certName;
private String policyMode;
private String cname;
private Boolean ocspEnabled;
private Boolean http2Enable;
private Boolean https2HttpEnable;
private List proxyTypes;
private List instanceIds;
private List customCiphers;
private List whiteList;
private List blackList;
private List realServers;
public String getDomain() {
return this.domain;
}
public void setDomain(String domain) {
this.domain = domain;
}
public Boolean getHttp2HttpsEnable() {
return this.http2HttpsEnable;
}
public void setHttp2HttpsEnable(Boolean http2HttpsEnable) {
this.http2HttpsEnable = http2HttpsEnable;
}
public String getSslProtocols() {
return this.sslProtocols;
}
public void setSslProtocols(String sslProtocols) {
this.sslProtocols = sslProtocols;
}
public Integer getPunishReason() {
return this.punishReason;
}
public void setPunishReason(Integer punishReason) {
this.punishReason = punishReason;
}
public String getCcTemplate() {
return this.ccTemplate;
}
public void setCcTemplate(String ccTemplate) {
this.ccTemplate = ccTemplate;
}
public String getHttpsExt() {
return this.httpsExt;
}
public void setHttpsExt(String httpsExt) {
this.httpsExt = httpsExt;
}
public Boolean getCcEnabled() {
return this.ccEnabled;
}
public void setCcEnabled(Boolean ccEnabled) {
this.ccEnabled = ccEnabled;
}
public String getSslCiphers() {
return this.sslCiphers;
}
public void setSslCiphers(String sslCiphers) {
this.sslCiphers = sslCiphers;
}
public Boolean getCcRuleEnabled() {
return this.ccRuleEnabled;
}
public void setCcRuleEnabled(Boolean ccRuleEnabled) {
this.ccRuleEnabled = ccRuleEnabled;
}
public Boolean getSsl13Enabled() {
return this.ssl13Enabled;
}
public void setSsl13Enabled(Boolean ssl13Enabled) {
this.ssl13Enabled = ssl13Enabled;
}
public Integer getRsType() {
return this.rsType;
}
public void setRsType(Integer rsType) {
this.rsType = rsType;
}
public Boolean getPunishStatus() {
return this.punishStatus;
}
public void setPunishStatus(Boolean punishStatus) {
this.punishStatus = punishStatus;
}
public Boolean getProxyEnabled() {
return this.proxyEnabled;
}
public void setProxyEnabled(Boolean proxyEnabled) {
this.proxyEnabled = proxyEnabled;
}
public String getCertName() {
return this.certName;
}
public void setCertName(String certName) {
this.certName = certName;
}
public String getPolicyMode() {
return this.policyMode;
}
public void setPolicyMode(String policyMode) {
this.policyMode = policyMode;
}
public String getCname() {
return this.cname;
}
public void setCname(String cname) {
this.cname = cname;
}
public Boolean getOcspEnabled() {
return this.ocspEnabled;
}
public void setOcspEnabled(Boolean ocspEnabled) {
this.ocspEnabled = ocspEnabled;
}
public Boolean getHttp2Enable() {
return this.http2Enable;
}
public void setHttp2Enable(Boolean http2Enable) {
this.http2Enable = http2Enable;
}
public Boolean getHttps2HttpEnable() {
return this.https2HttpEnable;
}
public void setHttps2HttpEnable(Boolean https2HttpEnable) {
this.https2HttpEnable = https2HttpEnable;
}
public List getProxyTypes() {
return this.proxyTypes;
}
public void setProxyTypes(List proxyTypes) {
this.proxyTypes = proxyTypes;
}
public List getInstanceIds() {
return this.instanceIds;
}
public void setInstanceIds(List instanceIds) {
this.instanceIds = instanceIds;
}
public List getCustomCiphers() {
return this.customCiphers;
}
public void setCustomCiphers(List customCiphers) {
this.customCiphers = customCiphers;
}
public List getWhiteList() {
return this.whiteList;
}
public void setWhiteList(List whiteList) {
this.whiteList = whiteList;
}
public List getBlackList() {
return this.blackList;
}
public void setBlackList(List blackList) {
this.blackList = blackList;
}
public List getRealServers() {
return this.realServers;
}
public void setRealServers(List realServers) {
this.realServers = realServers;
}
public static class ProxyConfig {
private String proxyType;
private List proxyPorts;
public String getProxyType() {
return this.proxyType;
}
public void setProxyType(String proxyType) {
this.proxyType = proxyType;
}
public List getProxyPorts() {
return this.proxyPorts;
}
public void setProxyPorts(List proxyPorts) {
this.proxyPorts = proxyPorts;
}
}
}
@Override
public DescribeDomainResourceResponse getInstance(UnmarshallerContext context) {
return DescribeDomainResourceResponseUnmarshaller.unmarshall(this, context);
}
@Override
public boolean checkShowJsonItemName() {
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy