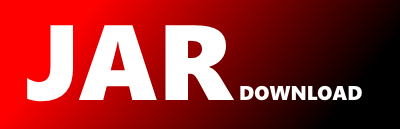
com.aliyuncs.polardb.model.v20170801.DescribeDBClusterMigrationResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aliyun-java-sdk-polardb Show documentation
Show all versions of aliyun-java-sdk-polardb Show documentation
Aliyun Open API SDK for Java
Copyright (C) Alibaba Cloud Computing
All rights reserved.
版权所有 (C)阿里云计算有限公司
http://www.aliyun.com
The newest version!
/*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.aliyuncs.polardb.model.v20170801;
import java.util.List;
import com.aliyuncs.AcsResponse;
import com.aliyuncs.polardb.transform.v20170801.DescribeDBClusterMigrationResponseUnmarshaller;
import com.aliyuncs.transform.UnmarshallerContext;
/**
* @author auto create
* @version
*/
public class DescribeDBClusterMigrationResponse extends AcsResponse {
private String comment;
private String requestId;
private String expiredTime;
private String dBClusterId;
private String topologies;
private String rdsReadWriteMode;
private String sourceRDSDBInstanceId;
private String dBClusterReadWriteMode;
private Integer delayedSeconds;
private String migrationStatus;
private String dtsInstanceId;
private String srcDbType;
private String migrationSwitch;
private String migrationDtsJobEndpoint;
private String dstBinlogPosition;
private String srcBinlogPosition;
private String migrationProgress;
private List dBClusterEndpointList;
private List rdsEndpointList;
private List srcDtsJobList;
private List dstDtsJobList;
public String getComment() {
return this.comment;
}
public void setComment(String comment) {
this.comment = comment;
}
public String getRequestId() {
return this.requestId;
}
public void setRequestId(String requestId) {
this.requestId = requestId;
}
public String getExpiredTime() {
return this.expiredTime;
}
public void setExpiredTime(String expiredTime) {
this.expiredTime = expiredTime;
}
public String getDBClusterId() {
return this.dBClusterId;
}
public void setDBClusterId(String dBClusterId) {
this.dBClusterId = dBClusterId;
}
public String getTopologies() {
return this.topologies;
}
public void setTopologies(String topologies) {
this.topologies = topologies;
}
public String getRdsReadWriteMode() {
return this.rdsReadWriteMode;
}
public void setRdsReadWriteMode(String rdsReadWriteMode) {
this.rdsReadWriteMode = rdsReadWriteMode;
}
public String getSourceRDSDBInstanceId() {
return this.sourceRDSDBInstanceId;
}
public void setSourceRDSDBInstanceId(String sourceRDSDBInstanceId) {
this.sourceRDSDBInstanceId = sourceRDSDBInstanceId;
}
public String getDBClusterReadWriteMode() {
return this.dBClusterReadWriteMode;
}
public void setDBClusterReadWriteMode(String dBClusterReadWriteMode) {
this.dBClusterReadWriteMode = dBClusterReadWriteMode;
}
public Integer getDelayedSeconds() {
return this.delayedSeconds;
}
public void setDelayedSeconds(Integer delayedSeconds) {
this.delayedSeconds = delayedSeconds;
}
public String getMigrationStatus() {
return this.migrationStatus;
}
public void setMigrationStatus(String migrationStatus) {
this.migrationStatus = migrationStatus;
}
public String getDtsInstanceId() {
return this.dtsInstanceId;
}
public void setDtsInstanceId(String dtsInstanceId) {
this.dtsInstanceId = dtsInstanceId;
}
public String getSrcDbType() {
return this.srcDbType;
}
public void setSrcDbType(String srcDbType) {
this.srcDbType = srcDbType;
}
public String getMigrationSwitch() {
return this.migrationSwitch;
}
public void setMigrationSwitch(String migrationSwitch) {
this.migrationSwitch = migrationSwitch;
}
public String getMigrationDtsJobEndpoint() {
return this.migrationDtsJobEndpoint;
}
public void setMigrationDtsJobEndpoint(String migrationDtsJobEndpoint) {
this.migrationDtsJobEndpoint = migrationDtsJobEndpoint;
}
public String getDstBinlogPosition() {
return this.dstBinlogPosition;
}
public void setDstBinlogPosition(String dstBinlogPosition) {
this.dstBinlogPosition = dstBinlogPosition;
}
public String getSrcBinlogPosition() {
return this.srcBinlogPosition;
}
public void setSrcBinlogPosition(String srcBinlogPosition) {
this.srcBinlogPosition = srcBinlogPosition;
}
public String getMigrationProgress() {
return this.migrationProgress;
}
public void setMigrationProgress(String migrationProgress) {
this.migrationProgress = migrationProgress;
}
public List getDBClusterEndpointList() {
return this.dBClusterEndpointList;
}
public void setDBClusterEndpointList(List dBClusterEndpointList) {
this.dBClusterEndpointList = dBClusterEndpointList;
}
public List getRdsEndpointList() {
return this.rdsEndpointList;
}
public void setRdsEndpointList(List rdsEndpointList) {
this.rdsEndpointList = rdsEndpointList;
}
public List getSrcDtsJobList() {
return this.srcDtsJobList;
}
public void setSrcDtsJobList(List srcDtsJobList) {
this.srcDtsJobList = srcDtsJobList;
}
public List getDstDtsJobList() {
return this.dstDtsJobList;
}
public void setDstDtsJobList(List dstDtsJobList) {
this.dstDtsJobList = dstDtsJobList;
}
public static class DBClusterEndpoint {
private String dBEndpointId;
private String endpointType;
private String readWriteMode;
private List addressItems;
public String getDBEndpointId() {
return this.dBEndpointId;
}
public void setDBEndpointId(String dBEndpointId) {
this.dBEndpointId = dBEndpointId;
}
public String getEndpointType() {
return this.endpointType;
}
public void setEndpointType(String endpointType) {
this.endpointType = endpointType;
}
public String getReadWriteMode() {
return this.readWriteMode;
}
public void setReadWriteMode(String readWriteMode) {
this.readWriteMode = readWriteMode;
}
public List getAddressItems() {
return this.addressItems;
}
public void setAddressItems(List addressItems) {
this.addressItems = addressItems;
}
public static class Address {
private String vSwitchId;
private String connectionString;
private String netType;
private String port;
private String vPCId;
private String iPAddress;
private String sSLEnabled;
public String getVSwitchId() {
return this.vSwitchId;
}
public void setVSwitchId(String vSwitchId) {
this.vSwitchId = vSwitchId;
}
public String getConnectionString() {
return this.connectionString;
}
public void setConnectionString(String connectionString) {
this.connectionString = connectionString;
}
public String getNetType() {
return this.netType;
}
public void setNetType(String netType) {
this.netType = netType;
}
public String getPort() {
return this.port;
}
public void setPort(String port) {
this.port = port;
}
public String getVPCId() {
return this.vPCId;
}
public void setVPCId(String vPCId) {
this.vPCId = vPCId;
}
public String getIPAddress() {
return this.iPAddress;
}
public void setIPAddress(String iPAddress) {
this.iPAddress = iPAddress;
}
public String getSSLEnabled() {
return this.sSLEnabled;
}
public void setSSLEnabled(String sSLEnabled) {
this.sSLEnabled = sSLEnabled;
}
}
}
public static class RdsEndpoint {
private String dBEndpointId;
private String endpointType;
private String custinsType;
private List addressItems1;
public String getDBEndpointId() {
return this.dBEndpointId;
}
public void setDBEndpointId(String dBEndpointId) {
this.dBEndpointId = dBEndpointId;
}
public String getEndpointType() {
return this.endpointType;
}
public void setEndpointType(String endpointType) {
this.endpointType = endpointType;
}
public String getCustinsType() {
return this.custinsType;
}
public void setCustinsType(String custinsType) {
this.custinsType = custinsType;
}
public List getAddressItems1() {
return this.addressItems1;
}
public void setAddressItems1(List addressItems1) {
this.addressItems1 = addressItems1;
}
public static class Address2 {
private String vSwitchId;
private String connectionString;
private String netType;
private String port;
private String vPCId;
private String iPAddress;
private String sSLEnabled;
public String getVSwitchId() {
return this.vSwitchId;
}
public void setVSwitchId(String vSwitchId) {
this.vSwitchId = vSwitchId;
}
public String getConnectionString() {
return this.connectionString;
}
public void setConnectionString(String connectionString) {
this.connectionString = connectionString;
}
public String getNetType() {
return this.netType;
}
public void setNetType(String netType) {
this.netType = netType;
}
public String getPort() {
return this.port;
}
public void setPort(String port) {
this.port = port;
}
public String getVPCId() {
return this.vPCId;
}
public void setVPCId(String vPCId) {
this.vPCId = vPCId;
}
public String getIPAddress() {
return this.iPAddress;
}
public void setIPAddress(String iPAddress) {
this.iPAddress = iPAddress;
}
public String getSSLEnabled() {
return this.sSLEnabled;
}
public void setSSLEnabled(String sSLEnabled) {
this.sSLEnabled = sSLEnabled;
}
}
}
public static class SrcDtsJob {
private String dtsJobId;
private String dtsJobName;
private String dtsInstanceID;
private String dtsJobDirection;
private String status;
private String sourceEndpoint;
private String destinationEndpoint;
public String getDtsJobId() {
return this.dtsJobId;
}
public void setDtsJobId(String dtsJobId) {
this.dtsJobId = dtsJobId;
}
public String getDtsJobName() {
return this.dtsJobName;
}
public void setDtsJobName(String dtsJobName) {
this.dtsJobName = dtsJobName;
}
public String getDtsInstanceID() {
return this.dtsInstanceID;
}
public void setDtsInstanceID(String dtsInstanceID) {
this.dtsInstanceID = dtsInstanceID;
}
public String getDtsJobDirection() {
return this.dtsJobDirection;
}
public void setDtsJobDirection(String dtsJobDirection) {
this.dtsJobDirection = dtsJobDirection;
}
public String getStatus() {
return this.status;
}
public void setStatus(String status) {
this.status = status;
}
public String getSourceEndpoint() {
return this.sourceEndpoint;
}
public void setSourceEndpoint(String sourceEndpoint) {
this.sourceEndpoint = sourceEndpoint;
}
public String getDestinationEndpoint() {
return this.destinationEndpoint;
}
public void setDestinationEndpoint(String destinationEndpoint) {
this.destinationEndpoint = destinationEndpoint;
}
}
public static class DstDtsJob {
private String dtsJobId;
private String dtsJobName;
private String dtsInstanceId;
private String dtsJobDirection;
private String status;
private String sourceEndpoint;
private String destinationEndpoint;
public String getDtsJobId() {
return this.dtsJobId;
}
public void setDtsJobId(String dtsJobId) {
this.dtsJobId = dtsJobId;
}
public String getDtsJobName() {
return this.dtsJobName;
}
public void setDtsJobName(String dtsJobName) {
this.dtsJobName = dtsJobName;
}
public String getDtsInstanceId() {
return this.dtsInstanceId;
}
public void setDtsInstanceId(String dtsInstanceId) {
this.dtsInstanceId = dtsInstanceId;
}
public String getDtsJobDirection() {
return this.dtsJobDirection;
}
public void setDtsJobDirection(String dtsJobDirection) {
this.dtsJobDirection = dtsJobDirection;
}
public String getStatus() {
return this.status;
}
public void setStatus(String status) {
this.status = status;
}
public String getSourceEndpoint() {
return this.sourceEndpoint;
}
public void setSourceEndpoint(String sourceEndpoint) {
this.sourceEndpoint = sourceEndpoint;
}
public String getDestinationEndpoint() {
return this.destinationEndpoint;
}
public void setDestinationEndpoint(String destinationEndpoint) {
this.destinationEndpoint = destinationEndpoint;
}
}
@Override
public DescribeDBClusterMigrationResponse getInstance(UnmarshallerContext context) {
return DescribeDBClusterMigrationResponseUnmarshaller.unmarshall(this, context);
}
@Override
public boolean checkShowJsonItemName() {
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy