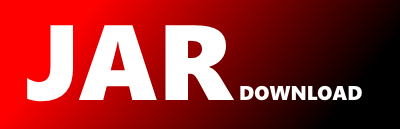
com.aliyuncs.sae.model.v20190506.ListWebApplicationsResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aliyun-java-sdk-sae Show documentation
Show all versions of aliyun-java-sdk-sae Show documentation
Aliyun Open API SDK for Java
Copyright (C) Alibaba Cloud Computing
All rights reserved.
版权所有 (C)阿里云计算有限公司
http://www.aliyun.com
The newest version!
/*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.aliyuncs.sae.model.v20190506;
import java.util.List;
import java.util.Map;
import com.aliyuncs.AcsResponse;
import com.aliyuncs.sae.transform.v20190506.ListWebApplicationsResponseUnmarshaller;
import com.aliyuncs.transform.UnmarshallerContext;
/**
* @author auto create
* @version
*/
public class ListWebApplicationsResponse extends AcsResponse {
private Integer code;
private String message;
private String requestId;
private Boolean success;
private Data data;
public Integer getCode() {
return this.code;
}
public void setCode(Integer code) {
this.code = code;
}
public String getMessage() {
return this.message;
}
public void setMessage(String message) {
this.message = message;
}
public String getRequestId() {
return this.requestId;
}
public void setRequestId(String requestId) {
this.requestId = requestId;
}
public Boolean getSuccess() {
return this.success;
}
public void setSuccess(Boolean success) {
this.success = success;
}
public Data getData() {
return this.data;
}
public void setData(Data data) {
this.data = data;
}
public static class Data {
private String nextToken;
private List webApplicationWithInstanceCount;
public String getNextToken() {
return this.nextToken;
}
public void setNextToken(String nextToken) {
this.nextToken = nextToken;
}
public List getWebApplicationWithInstanceCount() {
return this.webApplicationWithInstanceCount;
}
public void setWebApplicationWithInstanceCount(List webApplicationWithInstanceCount) {
this.webApplicationWithInstanceCount = webApplicationWithInstanceCount;
}
public static class WebApplicationWithInstanceCountItem {
private Long instanceCount;
private WebApplication webApplication;
public Long getInstanceCount() {
return this.instanceCount;
}
public void setInstanceCount(Long instanceCount) {
this.instanceCount = instanceCount;
}
public WebApplication getWebApplication() {
return this.webApplication;
}
public void setWebApplication(WebApplication webApplication) {
this.webApplication = webApplication;
}
public static class WebApplication {
private String applicationId;
private String applicationName;
private String createdTime;
private String description;
private String lastModifiedTime;
private String namespaceId;
private String internetURL;
private String intranetURL;
private String vpcId;
private RevisionConfig revisionConfig;
private WebScalingConfig webScalingConfig;
private WebTrafficConfig webTrafficConfig;
public String getApplicationId() {
return this.applicationId;
}
public void setApplicationId(String applicationId) {
this.applicationId = applicationId;
}
public String getApplicationName() {
return this.applicationName;
}
public void setApplicationName(String applicationName) {
this.applicationName = applicationName;
}
public String getCreatedTime() {
return this.createdTime;
}
public void setCreatedTime(String createdTime) {
this.createdTime = createdTime;
}
public String getDescription() {
return this.description;
}
public void setDescription(String description) {
this.description = description;
}
public String getLastModifiedTime() {
return this.lastModifiedTime;
}
public void setLastModifiedTime(String lastModifiedTime) {
this.lastModifiedTime = lastModifiedTime;
}
public String getNamespaceId() {
return this.namespaceId;
}
public void setNamespaceId(String namespaceId) {
this.namespaceId = namespaceId;
}
public String getInternetURL() {
return this.internetURL;
}
public void setInternetURL(String internetURL) {
this.internetURL = internetURL;
}
public String getIntranetURL() {
return this.intranetURL;
}
public void setIntranetURL(String intranetURL) {
this.intranetURL = intranetURL;
}
public String getVpcId() {
return this.vpcId;
}
public void setVpcId(String vpcId) {
this.vpcId = vpcId;
}
public RevisionConfig getRevisionConfig() {
return this.revisionConfig;
}
public void setRevisionConfig(RevisionConfig revisionConfig) {
this.revisionConfig = revisionConfig;
}
public WebScalingConfig getWebScalingConfig() {
return this.webScalingConfig;
}
public void setWebScalingConfig(WebScalingConfig webScalingConfig) {
this.webScalingConfig = webScalingConfig;
}
public WebTrafficConfig getWebTrafficConfig() {
return this.webTrafficConfig;
}
public void setWebTrafficConfig(WebTrafficConfig webTrafficConfig) {
this.webTrafficConfig = webTrafficConfig;
}
public static class RevisionConfig {
private Boolean enableArmsMetrics;
private List containers;
private WebNetworkConfig webNetworkConfig;
public Boolean getEnableArmsMetrics() {
return this.enableArmsMetrics;
}
public void setEnableArmsMetrics(Boolean enableArmsMetrics) {
this.enableArmsMetrics = enableArmsMetrics;
}
public List getContainers() {
return this.containers;
}
public void setContainers(List containers) {
this.containers = containers;
}
public WebNetworkConfig getWebNetworkConfig() {
return this.webNetworkConfig;
}
public void setWebNetworkConfig(WebNetworkConfig webNetworkConfig) {
this.webNetworkConfig = webNetworkConfig;
}
public static class ContainersItem {
private String args;
private String command;
private Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy