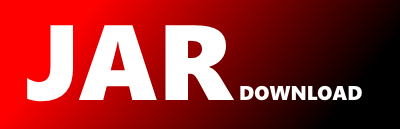
com.aliyuncs.sas.model.v20181203.DescribeAffectedMaliciousFileImagesRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aliyun-java-sdk-sas Show documentation
Show all versions of aliyun-java-sdk-sas Show documentation
Aliyun Open API SDK for Java
Copyright (C) Alibaba Cloud Computing
All rights reserved.
版权所有 (C)阿里云计算有限公司
http://www.aliyun.com
/*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.aliyuncs.sas.model.v20181203;
import com.aliyuncs.RpcAcsRequest;
import java.util.List;
import com.aliyuncs.http.MethodType;
import com.aliyuncs.sas.Endpoint;
/**
* @author auto create
* @version
*/
public class DescribeAffectedMaliciousFileImagesRequest extends RpcAcsRequest {
private String repoId;
private String pod;
private String clusterName;
private String repoNamespace;
private String imageDigest;
private List scanRanges;
private String pageSize;
private String lang;
private String imageTag;
private String image;
private String maliciousMd5;
private Integer currentPage;
private String clusterId;
private String repoName;
private String namespace;
private String repoInstanceId;
private String imageLayer;
private String containerId;
private String levels;
private String repoRegionId;
public DescribeAffectedMaliciousFileImagesRequest() {
super("Sas", "2018-12-03", "DescribeAffectedMaliciousFileImages");
setMethod(MethodType.POST);
try {
com.aliyuncs.AcsRequest.class.getDeclaredField("productEndpointMap").set(this, Endpoint.endpointMap);
com.aliyuncs.AcsRequest.class.getDeclaredField("productEndpointRegional").set(this, Endpoint.endpointRegionalType);
} catch (Exception e) {}
}
public String getRepoId() {
return this.repoId;
}
public void setRepoId(String repoId) {
this.repoId = repoId;
if(repoId != null){
putQueryParameter("RepoId", repoId);
}
}
public String getPod() {
return this.pod;
}
public void setPod(String pod) {
this.pod = pod;
if(pod != null){
putQueryParameter("Pod", pod);
}
}
public String getClusterName() {
return this.clusterName;
}
public void setClusterName(String clusterName) {
this.clusterName = clusterName;
if(clusterName != null){
putQueryParameter("ClusterName", clusterName);
}
}
public String getRepoNamespace() {
return this.repoNamespace;
}
public void setRepoNamespace(String repoNamespace) {
this.repoNamespace = repoNamespace;
if(repoNamespace != null){
putQueryParameter("RepoNamespace", repoNamespace);
}
}
public String getImageDigest() {
return this.imageDigest;
}
public void setImageDigest(String imageDigest) {
this.imageDigest = imageDigest;
if(imageDigest != null){
putQueryParameter("ImageDigest", imageDigest);
}
}
public List getScanRanges() {
return this.scanRanges;
}
public void setScanRanges(List scanRanges) {
this.scanRanges = scanRanges;
if (scanRanges != null) {
for (int i = 0; i < scanRanges.size(); i++) {
putQueryParameter("ScanRange." + (i + 1) , scanRanges.get(i));
}
}
}
public String getPageSize() {
return this.pageSize;
}
public void setPageSize(String pageSize) {
this.pageSize = pageSize;
if(pageSize != null){
putQueryParameter("PageSize", pageSize);
}
}
public String getLang() {
return this.lang;
}
public void setLang(String lang) {
this.lang = lang;
if(lang != null){
putQueryParameter("Lang", lang);
}
}
public String getImageTag() {
return this.imageTag;
}
public void setImageTag(String imageTag) {
this.imageTag = imageTag;
if(imageTag != null){
putQueryParameter("ImageTag", imageTag);
}
}
public String getImage() {
return this.image;
}
public void setImage(String image) {
this.image = image;
if(image != null){
putQueryParameter("Image", image);
}
}
public String getMaliciousMd5() {
return this.maliciousMd5;
}
public void setMaliciousMd5(String maliciousMd5) {
this.maliciousMd5 = maliciousMd5;
if(maliciousMd5 != null){
putQueryParameter("MaliciousMd5", maliciousMd5);
}
}
public Integer getCurrentPage() {
return this.currentPage;
}
public void setCurrentPage(Integer currentPage) {
this.currentPage = currentPage;
if(currentPage != null){
putQueryParameter("CurrentPage", currentPage.toString());
}
}
public String getClusterId() {
return this.clusterId;
}
public void setClusterId(String clusterId) {
this.clusterId = clusterId;
if(clusterId != null){
putQueryParameter("ClusterId", clusterId);
}
}
public String getRepoName() {
return this.repoName;
}
public void setRepoName(String repoName) {
this.repoName = repoName;
if(repoName != null){
putQueryParameter("RepoName", repoName);
}
}
public String getNamespace() {
return this.namespace;
}
public void setNamespace(String namespace) {
this.namespace = namespace;
if(namespace != null){
putQueryParameter("Namespace", namespace);
}
}
public String getRepoInstanceId() {
return this.repoInstanceId;
}
public void setRepoInstanceId(String repoInstanceId) {
this.repoInstanceId = repoInstanceId;
if(repoInstanceId != null){
putQueryParameter("RepoInstanceId", repoInstanceId);
}
}
public String getImageLayer() {
return this.imageLayer;
}
public void setImageLayer(String imageLayer) {
this.imageLayer = imageLayer;
if(imageLayer != null){
putQueryParameter("ImageLayer", imageLayer);
}
}
public String getContainerId() {
return this.containerId;
}
public void setContainerId(String containerId) {
this.containerId = containerId;
if(containerId != null){
putQueryParameter("ContainerId", containerId);
}
}
public String getLevels() {
return this.levels;
}
public void setLevels(String levels) {
this.levels = levels;
if(levels != null){
putQueryParameter("Levels", levels);
}
}
public String getRepoRegionId() {
return this.repoRegionId;
}
public void setRepoRegionId(String repoRegionId) {
this.repoRegionId = repoRegionId;
if(repoRegionId != null){
putQueryParameter("RepoRegionId", repoRegionId);
}
}
@Override
public Class getResponseClass() {
return DescribeAffectedMaliciousFileImagesResponse.class;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy