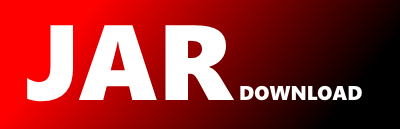
com.aliyuncs.vod.transform.v20170321.GetTranscodeSummaryResponseUnmarshaller Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aliyun-java-sdk-vod Show documentation
Show all versions of aliyun-java-sdk-vod Show documentation
Aliyun Open API SDK for Java
Copyright (C) Alibaba Cloud Computing
All rights reserved.
版权所有 (C)阿里云计算有限公司
http://www.aliyun.com
The newest version!
/*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.aliyuncs.vod.transform.v20170321;
import java.util.ArrayList;
import java.util.List;
import com.aliyuncs.vod.model.v20170321.GetTranscodeSummaryResponse;
import com.aliyuncs.vod.model.v20170321.GetTranscodeSummaryResponse.TranscodeSummary;
import com.aliyuncs.vod.model.v20170321.GetTranscodeSummaryResponse.TranscodeSummary.TranscodeJobInfoSummary;
import com.aliyuncs.transform.UnmarshallerContext;
public class GetTranscodeSummaryResponseUnmarshaller {
public static GetTranscodeSummaryResponse unmarshall(GetTranscodeSummaryResponse getTranscodeSummaryResponse, UnmarshallerContext _ctx) {
getTranscodeSummaryResponse.setRequestId(_ctx.stringValue("GetTranscodeSummaryResponse.RequestId"));
List nonExistVideoIds = new ArrayList();
for (int i = 0; i < _ctx.lengthValue("GetTranscodeSummaryResponse.NonExistVideoIds.Length"); i++) {
nonExistVideoIds.add(_ctx.stringValue("GetTranscodeSummaryResponse.NonExistVideoIds["+ i +"]"));
}
getTranscodeSummaryResponse.setNonExistVideoIds(nonExistVideoIds);
List transcodeSummaryList = new ArrayList();
for (int i = 0; i < _ctx.lengthValue("GetTranscodeSummaryResponse.TranscodeSummaryList.Length"); i++) {
TranscodeSummary transcodeSummary = new TranscodeSummary();
transcodeSummary.setCreationTime(_ctx.stringValue("GetTranscodeSummaryResponse.TranscodeSummaryList["+ i +"].CreationTime"));
transcodeSummary.setTrigger(_ctx.stringValue("GetTranscodeSummaryResponse.TranscodeSummaryList["+ i +"].Trigger"));
transcodeSummary.setVideoId(_ctx.stringValue("GetTranscodeSummaryResponse.TranscodeSummaryList["+ i +"].VideoId"));
transcodeSummary.setCompleteTime(_ctx.stringValue("GetTranscodeSummaryResponse.TranscodeSummaryList["+ i +"].CompleteTime"));
transcodeSummary.setTranscodeStatus(_ctx.stringValue("GetTranscodeSummaryResponse.TranscodeSummaryList["+ i +"].TranscodeStatus"));
transcodeSummary.setTranscodeTemplateGroupId(_ctx.stringValue("GetTranscodeSummaryResponse.TranscodeSummaryList["+ i +"].TranscodeTemplateGroupId"));
List transcodeJobInfoSummaryList = new ArrayList();
for (int j = 0; j < _ctx.lengthValue("GetTranscodeSummaryResponse.TranscodeSummaryList["+ i +"].TranscodeJobInfoSummaryList.Length"); j++) {
TranscodeJobInfoSummary transcodeJobInfoSummary = new TranscodeJobInfoSummary();
transcodeJobInfoSummary.setCreationTime(_ctx.stringValue("GetTranscodeSummaryResponse.TranscodeSummaryList["+ i +"].TranscodeJobInfoSummaryList["+ j +"].CreationTime"));
transcodeJobInfoSummary.setErrorMessage(_ctx.stringValue("GetTranscodeSummaryResponse.TranscodeSummaryList["+ i +"].TranscodeJobInfoSummaryList["+ j +"].ErrorMessage"));
transcodeJobInfoSummary.setEncryption(_ctx.stringValue("GetTranscodeSummaryResponse.TranscodeSummaryList["+ i +"].TranscodeJobInfoSummaryList["+ j +"].Encryption"));
transcodeJobInfoSummary.setHeight(_ctx.stringValue("GetTranscodeSummaryResponse.TranscodeSummaryList["+ i +"].TranscodeJobInfoSummaryList["+ j +"].Height"));
transcodeJobInfoSummary.setTranscodeProgress(_ctx.longValue("GetTranscodeSummaryResponse.TranscodeSummaryList["+ i +"].TranscodeJobInfoSummaryList["+ j +"].TranscodeProgress"));
transcodeJobInfoSummary.setTranscodeTemplateId(_ctx.stringValue("GetTranscodeSummaryResponse.TranscodeSummaryList["+ i +"].TranscodeJobInfoSummaryList["+ j +"].TranscodeTemplateId"));
transcodeJobInfoSummary.setBitrate(_ctx.stringValue("GetTranscodeSummaryResponse.TranscodeSummaryList["+ i +"].TranscodeJobInfoSummaryList["+ j +"].Bitrate"));
transcodeJobInfoSummary.setDefinition(_ctx.stringValue("GetTranscodeSummaryResponse.TranscodeSummaryList["+ i +"].TranscodeJobInfoSummaryList["+ j +"].Definition"));
transcodeJobInfoSummary.setErrorCode(_ctx.stringValue("GetTranscodeSummaryResponse.TranscodeSummaryList["+ i +"].TranscodeJobInfoSummaryList["+ j +"].ErrorCode"));
transcodeJobInfoSummary.setCompleteTime(_ctx.stringValue("GetTranscodeSummaryResponse.TranscodeSummaryList["+ i +"].TranscodeJobInfoSummaryList["+ j +"].CompleteTime"));
transcodeJobInfoSummary.setWidth(_ctx.stringValue("GetTranscodeSummaryResponse.TranscodeSummaryList["+ i +"].TranscodeJobInfoSummaryList["+ j +"].Width"));
transcodeJobInfoSummary.setDuration(_ctx.stringValue("GetTranscodeSummaryResponse.TranscodeSummaryList["+ i +"].TranscodeJobInfoSummaryList["+ j +"].Duration"));
transcodeJobInfoSummary.setFps(_ctx.stringValue("GetTranscodeSummaryResponse.TranscodeSummaryList["+ i +"].TranscodeJobInfoSummaryList["+ j +"].Fps"));
transcodeJobInfoSummary.setTranscodeJobStatus(_ctx.stringValue("GetTranscodeSummaryResponse.TranscodeSummaryList["+ i +"].TranscodeJobInfoSummaryList["+ j +"].TranscodeJobStatus"));
transcodeJobInfoSummary.setFilesize(_ctx.longValue("GetTranscodeSummaryResponse.TranscodeSummaryList["+ i +"].TranscodeJobInfoSummaryList["+ j +"].Filesize"));
transcodeJobInfoSummary.setFormat(_ctx.stringValue("GetTranscodeSummaryResponse.TranscodeSummaryList["+ i +"].TranscodeJobInfoSummaryList["+ j +"].Format"));
List watermarkIdList = new ArrayList();
for (int k = 0; k < _ctx.lengthValue("GetTranscodeSummaryResponse.TranscodeSummaryList["+ i +"].TranscodeJobInfoSummaryList["+ j +"].WatermarkIdList.Length"); k++) {
watermarkIdList.add(_ctx.stringValue("GetTranscodeSummaryResponse.TranscodeSummaryList["+ i +"].TranscodeJobInfoSummaryList["+ j +"].WatermarkIdList["+ k +"]"));
}
transcodeJobInfoSummary.setWatermarkIdList(watermarkIdList);
transcodeJobInfoSummaryList.add(transcodeJobInfoSummary);
}
transcodeSummary.setTranscodeJobInfoSummaryList(transcodeJobInfoSummaryList);
transcodeSummaryList.add(transcodeSummary);
}
getTranscodeSummaryResponse.setTranscodeSummaryList(transcodeSummaryList);
return getTranscodeSummaryResponse;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy