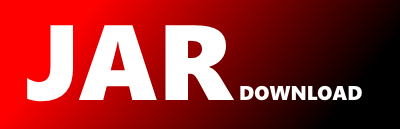
com.aliyun.arms20190808.models.CreateOrUpdateIMRobotRequest Maven / Gradle / Ivy
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.arms20190808.models;
import com.aliyun.tea.*;
public class CreateOrUpdateIMRobotRequest extends TeaModel {
/**
* The configurations of the alert card template. For more information about the parameters in the template, see the following section.
*
* example:
* { "button": [ "claim", "close", "follow", "send_itsm", "block", "unResolvedIncident" ], "field": [ { "fieldName": "alarmName", "visible": true }, { "fieldName": "notificationPolicy", "visible": true }, { "fieldName": "alarmContent", "visible": true }, { "fieldName": "alarmTime", "visible": true }, { "fieldName": "seriesChart", "visible": true }, { "fieldName": "includeEvent", "visible": true }, { "fieldName": "assigned", "visible": true }, { "fieldName": "similarAlarm", "visible": true }, { "fieldName": "operator", "visible": true } ] }
*/
@NameInMap("CardTemplate")
public String cardTemplate;
/**
* Specifies whether to send daily statistics. Valid values:
*
* false
(default): Daily statistics are not sent.
* true
: Daily statistics are sent. If you set the value to true
, the DailyNocTime parameter is required.
*
*
* example:
* true
*/
@NameInMap("DailyNoc")
public Boolean dailyNoc;
/**
* The points in time at which the daily statistics are sent. Separate multiple points in time with commas (,). The points in time are in the HH:SS format. The information that ARMS sends at the specified points in time includes the total number of alerts generated on the current day, the number of cleared alerts, and the number of alerts to be cleared.
*
* example:
* 09:30,17:00
*/
@NameInMap("DailyNocTime")
public String dailyNocTime;
/**
* The signature key of DingTalk. If you specify a signature key, DingTalk authentication is performed by using the signature key. If you do not specify a signature key, a whitelist is used for authentication by default. The keyword of the whitelist is Alert.
*
* example:
*
*/
@NameInMap("DingSignKey")
public String dingSignKey;
/**
* Specifies whether to enable the Outgoing feature.
*
* example:
* true
*/
@NameInMap("EnableOutgoing")
public Boolean enableOutgoing;
/**
* The webhook URL of the IM chatbot.
* This parameter is required.
*
* example:
* https://oapi.dingtalk.com/robot/send?access_token=e1a049121******
*/
@NameInMap("RobotAddress")
public String robotAddress;
/**
* The ID of the IM chatbot.
*
* If you do not specify the parameter, a new IM chatbot is created.
*
*
* example:
* 123
*/
@NameInMap("RobotId")
public Long robotId;
/**
* The name of the IM chatbot.
* This parameter is required.
*
* example:
* Chatbot name
*/
@NameInMap("RobotName")
public String robotName;
/**
* The token required to enable the Outgoing feature.
*
* example:
* 1656558719183be1245ab44********
*/
@NameInMap("Token")
public String token;
/**
* The type of the IM chatbot. Valid values:
*
* dingding
: DingTalk chatbot
* wechat
: WeCom chatbot
* feishu
: Lark chatbot
*
* This parameter is required.
*
* example:
* dingding
*/
@NameInMap("Type")
public String type;
public static CreateOrUpdateIMRobotRequest build(java.util.Map map) throws Exception {
CreateOrUpdateIMRobotRequest self = new CreateOrUpdateIMRobotRequest();
return TeaModel.build(map, self);
}
public CreateOrUpdateIMRobotRequest setCardTemplate(String cardTemplate) {
this.cardTemplate = cardTemplate;
return this;
}
public String getCardTemplate() {
return this.cardTemplate;
}
public CreateOrUpdateIMRobotRequest setDailyNoc(Boolean dailyNoc) {
this.dailyNoc = dailyNoc;
return this;
}
public Boolean getDailyNoc() {
return this.dailyNoc;
}
public CreateOrUpdateIMRobotRequest setDailyNocTime(String dailyNocTime) {
this.dailyNocTime = dailyNocTime;
return this;
}
public String getDailyNocTime() {
return this.dailyNocTime;
}
public CreateOrUpdateIMRobotRequest setDingSignKey(String dingSignKey) {
this.dingSignKey = dingSignKey;
return this;
}
public String getDingSignKey() {
return this.dingSignKey;
}
public CreateOrUpdateIMRobotRequest setEnableOutgoing(Boolean enableOutgoing) {
this.enableOutgoing = enableOutgoing;
return this;
}
public Boolean getEnableOutgoing() {
return this.enableOutgoing;
}
public CreateOrUpdateIMRobotRequest setRobotAddress(String robotAddress) {
this.robotAddress = robotAddress;
return this;
}
public String getRobotAddress() {
return this.robotAddress;
}
public CreateOrUpdateIMRobotRequest setRobotId(Long robotId) {
this.robotId = robotId;
return this;
}
public Long getRobotId() {
return this.robotId;
}
public CreateOrUpdateIMRobotRequest setRobotName(String robotName) {
this.robotName = robotName;
return this;
}
public String getRobotName() {
return this.robotName;
}
public CreateOrUpdateIMRobotRequest setToken(String token) {
this.token = token;
return this;
}
public String getToken() {
return this.token;
}
public CreateOrUpdateIMRobotRequest setType(String type) {
this.type = type;
return this;
}
public String getType() {
return this.type;
}
}