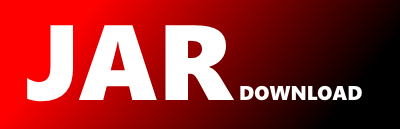
com.aliyun.arms20190808.models.CreateOrUpdateSilencePolicyRequest Maven / Gradle / Ivy
Show all versions of arms20190808 Show documentation
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.arms20190808.models;
import com.aliyun.tea.*;
public class CreateOrUpdateSilencePolicyRequest extends TeaModel {
/**
* The effective duration of the silence policy. Valid values: PERMANENT, CUSTOM_TIME, and CYCLE_EFFECT.
*
* example:
* PERMANENT
*/
@NameInMap("EffectiveTimeType")
public String effectiveTimeType;
/**
* The ID of the silence policy.
*
* - If you do not configure this parameter, a new silence policy is created.
* - If you configure this parameter, the specified silence policy is modified.
*
*
* example:
* 1234
*/
@NameInMap("Id")
public Long id;
/**
* The matching rules. The following code shows the format of matching rules:
* [
* {
* "matchingConditions": [
* {
* "value": "test", // The value of the matching condition.
* "key": "altertname", // The key of the matching condition.
* "operator": "eq" // The logical operator of the matching condition, including eq (equal to), neq (not equal to), in (contains), nin (does not contain), re (regular expression match), and nre (regular expression mismatch).
* }
* ]
* }
* ]
*
*
* example:
* [ { "matchingConditions": [ { "value": "test", "key": "altertname", "operator": "eq" } ] } ]
*/
@NameInMap("MatchingRules")
public String matchingRules;
/**
* The name of the silence policy.
* This parameter is required.
*
* example:
* silencepolicy_test
*/
@NameInMap("Name")
public String name;
/**
* The ID of the region.
*
* example:
* cn-hangzhou
*/
@NameInMap("RegionId")
public String regionId;
/**
* Specifies whether to enable the silence policy. Valid values: enable and disable.
*
* example:
* enable
*/
@NameInMap("State")
public String state;
/**
* The recurring period. This parameter is required when EffectiveTimeType is set to CYCLE_EFFECT. DAY: The silence policy is effective by day. WEEK: The silence policy is effective by week.
*
* example:
* DAY
*/
@NameInMap("TimePeriod")
public String timePeriod;
/**
* The time period during which the silence policy is effective. If you set EffectiveTimeType to CUSTOM_TIME, specify a custom time period in the following format: [{"startTime":"2024-08-04 22:13","endTime":"2024-08-04 22:21"}] If you set EffectiveTimeType to CYCLE_EFFECT and TimePeriod to DAY, specify a custom time period in the following format: [{"startTime":"22:13","endTime":"22:21"}]. The start time cannot be later than the end time. If you set EffectiveTimeType to CYCLE_EFFECT and TimePeriod to WEEK, specify a custom time period in the following format: [{"startWeek":"1", "endWeek":"2" "startTime":"22:13","endTime":"22:21"}]. Valid values of startWeek and endWeek: 1 to 7. The start time cannot be later than the end time.
*
* example:
* [{"startTime":"2024-08-04 22:13","endTime":"2024-08-04 22:21"}]
*/
@NameInMap("TimeSlots")
public String timeSlots;
public static CreateOrUpdateSilencePolicyRequest build(java.util.Map map) throws Exception {
CreateOrUpdateSilencePolicyRequest self = new CreateOrUpdateSilencePolicyRequest();
return TeaModel.build(map, self);
}
public CreateOrUpdateSilencePolicyRequest setEffectiveTimeType(String effectiveTimeType) {
this.effectiveTimeType = effectiveTimeType;
return this;
}
public String getEffectiveTimeType() {
return this.effectiveTimeType;
}
public CreateOrUpdateSilencePolicyRequest setId(Long id) {
this.id = id;
return this;
}
public Long getId() {
return this.id;
}
public CreateOrUpdateSilencePolicyRequest setMatchingRules(String matchingRules) {
this.matchingRules = matchingRules;
return this;
}
public String getMatchingRules() {
return this.matchingRules;
}
public CreateOrUpdateSilencePolicyRequest setName(String name) {
this.name = name;
return this;
}
public String getName() {
return this.name;
}
public CreateOrUpdateSilencePolicyRequest setRegionId(String regionId) {
this.regionId = regionId;
return this;
}
public String getRegionId() {
return this.regionId;
}
public CreateOrUpdateSilencePolicyRequest setState(String state) {
this.state = state;
return this;
}
public String getState() {
return this.state;
}
public CreateOrUpdateSilencePolicyRequest setTimePeriod(String timePeriod) {
this.timePeriod = timePeriod;
return this;
}
public String getTimePeriod() {
return this.timePeriod;
}
public CreateOrUpdateSilencePolicyRequest setTimeSlots(String timeSlots) {
this.timeSlots = timeSlots;
return this;
}
public String getTimeSlots() {
return this.timeSlots;
}
}