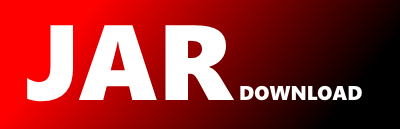
com.aliyun.arms20190808.models.GetTraceAppResponseBody Maven / Gradle / Ivy
Show all versions of arms20190808 Show documentation
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.arms20190808.models;
import com.aliyun.tea.*;
public class GetTraceAppResponseBody extends TeaModel {
/**
* The request ID.
*
* example:
* D80ADAAC-8C32-5479-BD14-C28CF832****
*/
@NameInMap("RequestId")
public String requestId;
/**
* The information about the array object.
*/
@NameInMap("TraceApp")
public GetTraceAppResponseBodyTraceApp traceApp;
public static GetTraceAppResponseBody build(java.util.Map map) throws Exception {
GetTraceAppResponseBody self = new GetTraceAppResponseBody();
return TeaModel.build(map, self);
}
public GetTraceAppResponseBody setRequestId(String requestId) {
this.requestId = requestId;
return this;
}
public String getRequestId() {
return this.requestId;
}
public GetTraceAppResponseBody setTraceApp(GetTraceAppResponseBodyTraceApp traceApp) {
this.traceApp = traceApp;
return this;
}
public GetTraceAppResponseBodyTraceApp getTraceApp() {
return this.traceApp;
}
public static class GetTraceAppResponseBodyTraceAppTags extends TeaModel {
/**
* The tag key.
*
* example:
* TestKey
*/
@NameInMap("Key")
public String key;
/**
* The tag value.
*
* example:
* TestValue
*/
@NameInMap("Value")
public String value;
public static GetTraceAppResponseBodyTraceAppTags build(java.util.Map map) throws Exception {
GetTraceAppResponseBodyTraceAppTags self = new GetTraceAppResponseBodyTraceAppTags();
return TeaModel.build(map, self);
}
public GetTraceAppResponseBodyTraceAppTags setKey(String key) {
this.key = key;
return this;
}
public String getKey() {
return this.key;
}
public GetTraceAppResponseBodyTraceAppTags setValue(String value) {
this.value = value;
return this;
}
public String getValue() {
return this.value;
}
}
public static class GetTraceAppResponseBodyTraceApp extends TeaModel {
/**
* The application ID.
*
* example:
* 123
*/
@NameInMap("AppId")
public Long appId;
/**
* The name of the application.
*
* example:
* arms-k8s-demo
*/
@NameInMap("AppName")
public String appName;
/**
* Cluster ID, used only in K8s scenarios.
*
* example:
* c905d1364c2dd4b6284a3f41790c4****
*/
@NameInMap("ClusterId")
public String clusterId;
/**
* The timestamp generated when the task was created.
*
* example:
* 1576599253000
*/
@NameInMap("CreateTime")
public Long createTime;
/**
* The aliases of the application.
*/
@NameInMap("Labels")
public java.util.List labels;
/**
* Coding Language.
*
* example:
* java
*/
@NameInMap("Language")
public String language;
/**
* The process identifier (PID) of the application.
*
* example:
* b590lhguqs@d8deedfa9bf****
*/
@NameInMap("Pid")
public String pid;
/**
* The region ID.
*
* example:
* cn-hangzhou
*/
@NameInMap("RegionId")
public String regionId;
/**
* The ID of the resource group.
*
* example:
* rg-acfmxyexli2****
*/
@NameInMap("ResourceGroupId")
public String resourceGroupId;
/**
* Indicates whether the application is displayed in the Application Real-Time Monitoring Service (ARMS) console. Valid values:
*
* true
: yes
* false
: no
*
*
* example:
* true
*/
@NameInMap("Show")
public Boolean show;
/**
* The source of the application.
*
* example:
* ACSK8S
*/
@NameInMap("Source")
public String source;
/**
* A list of key-value pairs.
*/
@NameInMap("Tags")
public java.util.List tags;
/**
* The type of the monitoring task. Valid values:
*
* TRACE
: Application Monitoring
* RETCODE
: Browser Monitoring
*
*
* example:
* TRACE
*/
@NameInMap("Type")
public String type;
/**
* The timestamp generated when the task information was updated.
*
* example:
* 1635700348000
*/
@NameInMap("UpdateTime")
public Long updateTime;
/**
* The user ID.
*
* example:
* 113197164949****
*/
@NameInMap("UserId")
public String userId;
public static GetTraceAppResponseBodyTraceApp build(java.util.Map map) throws Exception {
GetTraceAppResponseBodyTraceApp self = new GetTraceAppResponseBodyTraceApp();
return TeaModel.build(map, self);
}
public GetTraceAppResponseBodyTraceApp setAppId(Long appId) {
this.appId = appId;
return this;
}
public Long getAppId() {
return this.appId;
}
public GetTraceAppResponseBodyTraceApp setAppName(String appName) {
this.appName = appName;
return this;
}
public String getAppName() {
return this.appName;
}
public GetTraceAppResponseBodyTraceApp setClusterId(String clusterId) {
this.clusterId = clusterId;
return this;
}
public String getClusterId() {
return this.clusterId;
}
public GetTraceAppResponseBodyTraceApp setCreateTime(Long createTime) {
this.createTime = createTime;
return this;
}
public Long getCreateTime() {
return this.createTime;
}
public GetTraceAppResponseBodyTraceApp setLabels(java.util.List labels) {
this.labels = labels;
return this;
}
public java.util.List getLabels() {
return this.labels;
}
public GetTraceAppResponseBodyTraceApp setLanguage(String language) {
this.language = language;
return this;
}
public String getLanguage() {
return this.language;
}
public GetTraceAppResponseBodyTraceApp setPid(String pid) {
this.pid = pid;
return this;
}
public String getPid() {
return this.pid;
}
public GetTraceAppResponseBodyTraceApp setRegionId(String regionId) {
this.regionId = regionId;
return this;
}
public String getRegionId() {
return this.regionId;
}
public GetTraceAppResponseBodyTraceApp setResourceGroupId(String resourceGroupId) {
this.resourceGroupId = resourceGroupId;
return this;
}
public String getResourceGroupId() {
return this.resourceGroupId;
}
public GetTraceAppResponseBodyTraceApp setShow(Boolean show) {
this.show = show;
return this;
}
public Boolean getShow() {
return this.show;
}
public GetTraceAppResponseBodyTraceApp setSource(String source) {
this.source = source;
return this;
}
public String getSource() {
return this.source;
}
public GetTraceAppResponseBodyTraceApp setTags(java.util.List tags) {
this.tags = tags;
return this;
}
public java.util.List getTags() {
return this.tags;
}
public GetTraceAppResponseBodyTraceApp setType(String type) {
this.type = type;
return this;
}
public String getType() {
return this.type;
}
public GetTraceAppResponseBodyTraceApp setUpdateTime(Long updateTime) {
this.updateTime = updateTime;
return this;
}
public Long getUpdateTime() {
return this.updateTime;
}
public GetTraceAppResponseBodyTraceApp setUserId(String userId) {
this.userId = userId;
return this;
}
public String getUserId() {
return this.userId;
}
}
}