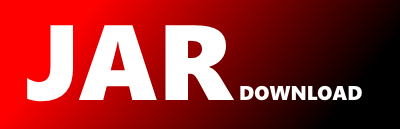
com.aliyun.arms20190808.models.DescribeEnvironmentResponseBody Maven / Gradle / Ivy
Show all versions of arms20190808 Show documentation
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.arms20190808.models;
import com.aliyun.tea.*;
public class DescribeEnvironmentResponseBody extends TeaModel {
/**
* The HTTP status code. The status code 200 indicates that the request was successful. Other status codes indicate that the request failed.
*
* example:
* 200
*/
@NameInMap("Code")
public Integer code;
/**
* The returned struct.
*/
@NameInMap("Data")
public DescribeEnvironmentResponseBodyData data;
/**
* The returned message.
*
* example:
* message
*/
@NameInMap("Message")
public String message;
/**
* The request ID.
*
* example:
* C21AB7CF-B7AF-410F-BD61-82D1567F****
*/
@NameInMap("RequestId")
public String requestId;
public static DescribeEnvironmentResponseBody build(java.util.Map map) throws Exception {
DescribeEnvironmentResponseBody self = new DescribeEnvironmentResponseBody();
return TeaModel.build(map, self);
}
public DescribeEnvironmentResponseBody setCode(Integer code) {
this.code = code;
return this;
}
public Integer getCode() {
return this.code;
}
public DescribeEnvironmentResponseBody setData(DescribeEnvironmentResponseBodyData data) {
this.data = data;
return this;
}
public DescribeEnvironmentResponseBodyData getData() {
return this.data;
}
public DescribeEnvironmentResponseBody setMessage(String message) {
this.message = message;
return this;
}
public String getMessage() {
return this.message;
}
public DescribeEnvironmentResponseBody setRequestId(String requestId) {
this.requestId = requestId;
return this;
}
public String getRequestId() {
return this.requestId;
}
public static class DescribeEnvironmentResponseBodyDataTags extends TeaModel {
/**
* The tag key.
*
* example:
* user1
*/
@NameInMap("Key")
public String key;
/**
* The tag value.
*
* example:
* p_dev
*/
@NameInMap("Value")
public String value;
public static DescribeEnvironmentResponseBodyDataTags build(java.util.Map map) throws Exception {
DescribeEnvironmentResponseBodyDataTags self = new DescribeEnvironmentResponseBodyDataTags();
return TeaModel.build(map, self);
}
public DescribeEnvironmentResponseBodyDataTags setKey(String key) {
this.key = key;
return this;
}
public String getKey() {
return this.key;
}
public DescribeEnvironmentResponseBodyDataTags setValue(String value) {
this.value = value;
return this;
}
public String getValue() {
return this.value;
}
}
public static class DescribeEnvironmentResponseBodyData extends TeaModel {
/**
* The ID of the resource associated with the environment, such as the ACK cluster ID or VPC ID.
*
* example:
* vpc-xxxxx
*/
@NameInMap("BindResourceId")
public String bindResourceId;
/**
* The profile of the resource.
*
* example:
* Default
*/
@NameInMap("BindResourceProfile")
public String bindResourceProfile;
/**
* The status of the resource.
*
* example:
* running
*/
@NameInMap("BindResourceStatus")
public String bindResourceStatus;
/**
* The retention period of the resource. Unit: days.
*
* example:
* 15
*/
@NameInMap("BindResourceStoreDuration")
public String bindResourceStoreDuration;
/**
* The resource type.
*
* example:
* ECS
*/
@NameInMap("BindResourceType")
public String bindResourceType;
/**
* The VPC CIDR block.
*
* example:
* 192.168.0.0/16
*/
@NameInMap("BindVpcCidr")
public String bindVpcCidr;
/**
* The status of the database that is bound to the Prometheus instance.
* Valid values:
*
* UNINSTALLING
*
*
*
*
*
*
* INSTALLING
*
*
*
*
*
*
* UNINSTALLED
*
*
*
*
*
*
* RUNNING
*
*
*
*
*
*
* MODIFYING
*
*
*
*
*
*
*
* example:
* RUNNING
*/
@NameInMap("DbInstanceStatus")
public String dbInstanceStatus;
/**
* The ID of the environment instance.
*
* example:
* env-xxxxx
*/
@NameInMap("EnvironmentId")
public String environmentId;
/**
* The environment name.
*
* example:
* env1
*/
@NameInMap("EnvironmentName")
public String environmentName;
/**
* Environment subtypes:
*
* - CS: Currently supports ACK.
* - ECS: ECS is currently supported.
* - Cloud: Currently supports Cloud.
*
*
* example:
* ACK
*/
@NameInMap("EnvironmentSubType")
public String environmentSubType;
/**
* The type of the environment. Valid values:
*
* - CS: Container Service for Kubernetes (ACK)
* - ECS: Elastic Compute Service
* - Cloud: cloud service
*
*
* example:
* CS
*/
@NameInMap("EnvironmentType")
public String environmentType;
/**
* The payable resource plan. Valid values:
*
* - If the EnvironmentType parameter is set to CS, set the value to CS_Basic or CS_Pro.
* - Otherwise, leave the parameter empty.
*
*
* example:
* CS_Basic
*/
@NameInMap("FeePackage")
public String feePackage;
/**
* The name of the Grafana data source.
*
* example:
* datasource1
*/
@NameInMap("GrafaDataSourceName")
public String grafaDataSourceName;
/**
* The unique ID of the Grafana data source.
*
* example:
* zuvw
*/
@NameInMap("GrafanaDatasourceUid")
public String grafanaDatasourceUid;
/**
* The name of the Grafana directory.
*
* example:
* folder1
*/
@NameInMap("GrafanaFolderTitle")
public String grafanaFolderTitle;
/**
* The unique ID of the Grafana directory.
*
* example:
* xyz
*/
@NameInMap("GrafanaFolderUid")
public String grafanaFolderUid;
/**
* The URL of the Grafana directory.
*
* example:
* https://g.console.aliyun.com/dashboards/f/xxx/yyyy
*/
@NameInMap("GrafanaFolderUrl")
public String grafanaFolderUrl;
/**
* The ID of the Grafana workspace.
*
* example:
* grafana-cn-27a3m8eem0a
*/
@NameInMap("GrafanaWorkspaceId")
public String grafanaWorkspaceId;
/**
* managed type:
*
* - none: unmanaged. The default value for ACK clusters.
* - agent: managed agent (including KSM). The default values for ASK, ACS, and AckOne clusters.
* - agent-exporter: managed agent and exporters. The default value for the cloud service type.
*
*
* example:
* none
*/
@NameInMap("ManagedType")
public String managedType;
/**
* The ID of the Prometheus instance.
*
* example:
* xxxxyyyyyzzzzz
*/
@NameInMap("PrometheusInstanceId")
public String prometheusInstanceId;
/**
* The name of the Prometheus instance.
*
* example:
* name1
*/
@NameInMap("PrometheusInstanceName")
public String prometheusInstanceName;
/**
* The region ID.
*
* example:
* cn-hangzhou
*/
@NameInMap("RegionId")
public String regionId;
/**
* The ID of the resource group.
*
* example:
* rg-aek2vezare****
*/
@NameInMap("ResourceGroupId")
public String resourceGroupId;
/**
* The ID of the security group associated with the environment.
*
* example:
* sg-8vbdgmf4nraiqa9bx0jo
*/
@NameInMap("SecurityGroupId")
public String securityGroupId;
/**
* The tags.
*/
@NameInMap("Tags")
public java.util.List tags;
/**
* The user ID.
*
* example:
* 13002222xxxx
*/
@NameInMap("UserId")
public String userId;
/**
* The VPC ID.
*
* example:
* vpc-8vb02uk57qbcktqcvqqqj
*/
@NameInMap("VpcId")
public String vpcId;
/**
* The ID of the vSwitch associated with the environment.
*
* example:
* vsw-2ze7yr3f1x8snryaioo7u
*/
@NameInMap("VswitchId")
public String vswitchId;
public static DescribeEnvironmentResponseBodyData build(java.util.Map map) throws Exception {
DescribeEnvironmentResponseBodyData self = new DescribeEnvironmentResponseBodyData();
return TeaModel.build(map, self);
}
public DescribeEnvironmentResponseBodyData setBindResourceId(String bindResourceId) {
this.bindResourceId = bindResourceId;
return this;
}
public String getBindResourceId() {
return this.bindResourceId;
}
public DescribeEnvironmentResponseBodyData setBindResourceProfile(String bindResourceProfile) {
this.bindResourceProfile = bindResourceProfile;
return this;
}
public String getBindResourceProfile() {
return this.bindResourceProfile;
}
public DescribeEnvironmentResponseBodyData setBindResourceStatus(String bindResourceStatus) {
this.bindResourceStatus = bindResourceStatus;
return this;
}
public String getBindResourceStatus() {
return this.bindResourceStatus;
}
public DescribeEnvironmentResponseBodyData setBindResourceStoreDuration(String bindResourceStoreDuration) {
this.bindResourceStoreDuration = bindResourceStoreDuration;
return this;
}
public String getBindResourceStoreDuration() {
return this.bindResourceStoreDuration;
}
public DescribeEnvironmentResponseBodyData setBindResourceType(String bindResourceType) {
this.bindResourceType = bindResourceType;
return this;
}
public String getBindResourceType() {
return this.bindResourceType;
}
public DescribeEnvironmentResponseBodyData setBindVpcCidr(String bindVpcCidr) {
this.bindVpcCidr = bindVpcCidr;
return this;
}
public String getBindVpcCidr() {
return this.bindVpcCidr;
}
public DescribeEnvironmentResponseBodyData setDbInstanceStatus(String dbInstanceStatus) {
this.dbInstanceStatus = dbInstanceStatus;
return this;
}
public String getDbInstanceStatus() {
return this.dbInstanceStatus;
}
public DescribeEnvironmentResponseBodyData setEnvironmentId(String environmentId) {
this.environmentId = environmentId;
return this;
}
public String getEnvironmentId() {
return this.environmentId;
}
public DescribeEnvironmentResponseBodyData setEnvironmentName(String environmentName) {
this.environmentName = environmentName;
return this;
}
public String getEnvironmentName() {
return this.environmentName;
}
public DescribeEnvironmentResponseBodyData setEnvironmentSubType(String environmentSubType) {
this.environmentSubType = environmentSubType;
return this;
}
public String getEnvironmentSubType() {
return this.environmentSubType;
}
public DescribeEnvironmentResponseBodyData setEnvironmentType(String environmentType) {
this.environmentType = environmentType;
return this;
}
public String getEnvironmentType() {
return this.environmentType;
}
public DescribeEnvironmentResponseBodyData setFeePackage(String feePackage) {
this.feePackage = feePackage;
return this;
}
public String getFeePackage() {
return this.feePackage;
}
public DescribeEnvironmentResponseBodyData setGrafaDataSourceName(String grafaDataSourceName) {
this.grafaDataSourceName = grafaDataSourceName;
return this;
}
public String getGrafaDataSourceName() {
return this.grafaDataSourceName;
}
public DescribeEnvironmentResponseBodyData setGrafanaDatasourceUid(String grafanaDatasourceUid) {
this.grafanaDatasourceUid = grafanaDatasourceUid;
return this;
}
public String getGrafanaDatasourceUid() {
return this.grafanaDatasourceUid;
}
public DescribeEnvironmentResponseBodyData setGrafanaFolderTitle(String grafanaFolderTitle) {
this.grafanaFolderTitle = grafanaFolderTitle;
return this;
}
public String getGrafanaFolderTitle() {
return this.grafanaFolderTitle;
}
public DescribeEnvironmentResponseBodyData setGrafanaFolderUid(String grafanaFolderUid) {
this.grafanaFolderUid = grafanaFolderUid;
return this;
}
public String getGrafanaFolderUid() {
return this.grafanaFolderUid;
}
public DescribeEnvironmentResponseBodyData setGrafanaFolderUrl(String grafanaFolderUrl) {
this.grafanaFolderUrl = grafanaFolderUrl;
return this;
}
public String getGrafanaFolderUrl() {
return this.grafanaFolderUrl;
}
public DescribeEnvironmentResponseBodyData setGrafanaWorkspaceId(String grafanaWorkspaceId) {
this.grafanaWorkspaceId = grafanaWorkspaceId;
return this;
}
public String getGrafanaWorkspaceId() {
return this.grafanaWorkspaceId;
}
public DescribeEnvironmentResponseBodyData setManagedType(String managedType) {
this.managedType = managedType;
return this;
}
public String getManagedType() {
return this.managedType;
}
public DescribeEnvironmentResponseBodyData setPrometheusInstanceId(String prometheusInstanceId) {
this.prometheusInstanceId = prometheusInstanceId;
return this;
}
public String getPrometheusInstanceId() {
return this.prometheusInstanceId;
}
public DescribeEnvironmentResponseBodyData setPrometheusInstanceName(String prometheusInstanceName) {
this.prometheusInstanceName = prometheusInstanceName;
return this;
}
public String getPrometheusInstanceName() {
return this.prometheusInstanceName;
}
public DescribeEnvironmentResponseBodyData setRegionId(String regionId) {
this.regionId = regionId;
return this;
}
public String getRegionId() {
return this.regionId;
}
public DescribeEnvironmentResponseBodyData setResourceGroupId(String resourceGroupId) {
this.resourceGroupId = resourceGroupId;
return this;
}
public String getResourceGroupId() {
return this.resourceGroupId;
}
public DescribeEnvironmentResponseBodyData setSecurityGroupId(String securityGroupId) {
this.securityGroupId = securityGroupId;
return this;
}
public String getSecurityGroupId() {
return this.securityGroupId;
}
public DescribeEnvironmentResponseBodyData setTags(java.util.List tags) {
this.tags = tags;
return this;
}
public java.util.List getTags() {
return this.tags;
}
public DescribeEnvironmentResponseBodyData setUserId(String userId) {
this.userId = userId;
return this;
}
public String getUserId() {
return this.userId;
}
public DescribeEnvironmentResponseBodyData setVpcId(String vpcId) {
this.vpcId = vpcId;
return this;
}
public String getVpcId() {
return this.vpcId;
}
public DescribeEnvironmentResponseBodyData setVswitchId(String vswitchId) {
this.vswitchId = vswitchId;
return this;
}
public String getVswitchId() {
return this.vswitchId;
}
}
}