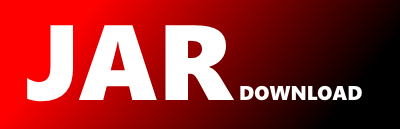
com.aliyun.cas20200630.models.DescribeClientCertificateResponseBody Maven / Gradle / Ivy
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.cas20200630.models;
import com.aliyun.tea.*;
public class DescribeClientCertificateResponseBody extends TeaModel {
/**
* The details about the client certificate or the server certificate.
*/
@NameInMap("Certificate")
public DescribeClientCertificateResponseBodyCertificate certificate;
/**
* The ID of the request.
*/
@NameInMap("RequestId")
public String requestId;
public static DescribeClientCertificateResponseBody build(java.util.Map map) throws Exception {
DescribeClientCertificateResponseBody self = new DescribeClientCertificateResponseBody();
return TeaModel.build(map, self);
}
public DescribeClientCertificateResponseBody setCertificate(DescribeClientCertificateResponseBodyCertificate certificate) {
this.certificate = certificate;
return this;
}
public DescribeClientCertificateResponseBodyCertificate getCertificate() {
return this.certificate;
}
public DescribeClientCertificateResponseBody setRequestId(String requestId) {
this.requestId = requestId;
return this;
}
public String getRequestId() {
return this.requestId;
}
public static class DescribeClientCertificateResponseBodyCertificate extends TeaModel {
/**
* The expiration date of the certificate. This value is a UNIX timestamp. Unit: milliseconds.
*/
@NameInMap("AfterDate")
public Long afterDate;
/**
* The type of the encryption algorithm of the certificate. Valid values:
*
* * **RSA**: the Rivest-Shamir-Adleman (RSA) algorithm.
* * **ECC**: the elliptic curve cryptography (ECC) algorithm.
* * **SM2**: the SM2 algorithm, which is developed and approved by the State Cryptography Administration of China.
*/
@NameInMap("Algorithm")
public String algorithm;
/**
* The issuance date of the certificate. This value is a UNIX timestamp. Unit: milliseconds.
*/
@NameInMap("BeforeDate")
public Long beforeDate;
/**
* The type of the certificate. Valid values:
*
* * **CLIENT**: client certificate
* * **SERVER**: server certificate
*/
@NameInMap("CertificateType")
public String certificateType;
/**
* The common name of the certificate.
*/
@NameInMap("CommonName")
public String commonName;
/**
* The code of the country in which the organization is located. The organization is associated with the intermediate certificate from which the certificate is issued.
*
* For more information about country codes, see the **"Country codes"** section of the [Manage company profiles](~~198289~~) topic.
*/
@NameInMap("CountryCode")
public String countryCode;
/**
* The validity period of the certificate. Unit: days.
*/
@NameInMap("Days")
public Integer days;
/**
* The unique identifier of the certificate.
*/
@NameInMap("Identifier")
public String identifier;
/**
* The key length of the certificate.
*/
@NameInMap("KeySize")
public Integer keySize;
/**
* The name of the city in which the organization is located. The organization is associated with the intermediate certificate from which the certificate is issued.
*/
@NameInMap("Locality")
public String locality;
/**
* The MD5 fingerprint of the certificate.
*/
@NameInMap("Md5")
public String md5;
/**
* The name of the organization. The organization is associated with the intermediate certificate from which the certificate is issued.
*/
@NameInMap("Organization")
public String organization;
/**
* The name of the department in the organization. The organization is associated with the intermediate certificate authority (CA) certificate from which the certificate is issued.
*/
@NameInMap("OrganizationUnit")
public String organizationUnit;
/**
* The unique identifier of the intermediate certificate from which the client certificate is issued.
*/
@NameInMap("ParentIdentifier")
public String parentIdentifier;
/**
* The subject alternative name (SAN) extension of the certificate. The value indicates additional information, including the additional domain names or IP addresses that are associated with the certificate.
*
* The value is a string that consists of JSON arrays. Each element in a JSON array is a JSON struct that corresponds to a SAN extension. A SAN extension struct contains the following parameters:
*
* * **Type**: the type of the extension. Data type: integer. Valid values:
*
* * **1**: an email address
* * **2**: a domain name
* * **6**: a Uniform Resource Identifier (URI)
* * **7**: an IP address
*
* * **Value**: the value of the extension. Data type: string.
*/
@NameInMap("Sans")
public String sans;
/**
* The serial number of the certificate.
*/
@NameInMap("SerialNumber")
public String serialNumber;
/**
* The SHA-256 fingerprint of the certificate.
*/
@NameInMap("Sha2")
public String sha2;
/**
* The signature algorithm of the certificate.
*/
@NameInMap("SignAlgorithm")
public String signAlgorithm;
/**
* The name of the province, municipality, or autonomous region in which the organization is located. The organization is associated with the intermediate certificate from which the certificate is issued.
*/
@NameInMap("State")
public String state;
/**
* The status of the certificate. Valid values:
*
* * **ISSUE**: issued
* * **REVOKE**: revoked
*/
@NameInMap("Status")
public String status;
/**
* The distinguished name (DN) extension of the certificate, which indicates the user of the certificate. The DN extension includes the following information:
*
* * **C**: the country
* * **O**: the organization
* * **OU**: the department
* * **L**: the city
* * **ST**: the province, municipality, or autonomous region
* * **CN**: the common name
*/
@NameInMap("SubjectDN")
public String subjectDN;
/**
* The content of the certificate.
*/
@NameInMap("X509Certificate")
public String x509Certificate;
public static DescribeClientCertificateResponseBodyCertificate build(java.util.Map map) throws Exception {
DescribeClientCertificateResponseBodyCertificate self = new DescribeClientCertificateResponseBodyCertificate();
return TeaModel.build(map, self);
}
public DescribeClientCertificateResponseBodyCertificate setAfterDate(Long afterDate) {
this.afterDate = afterDate;
return this;
}
public Long getAfterDate() {
return this.afterDate;
}
public DescribeClientCertificateResponseBodyCertificate setAlgorithm(String algorithm) {
this.algorithm = algorithm;
return this;
}
public String getAlgorithm() {
return this.algorithm;
}
public DescribeClientCertificateResponseBodyCertificate setBeforeDate(Long beforeDate) {
this.beforeDate = beforeDate;
return this;
}
public Long getBeforeDate() {
return this.beforeDate;
}
public DescribeClientCertificateResponseBodyCertificate setCertificateType(String certificateType) {
this.certificateType = certificateType;
return this;
}
public String getCertificateType() {
return this.certificateType;
}
public DescribeClientCertificateResponseBodyCertificate setCommonName(String commonName) {
this.commonName = commonName;
return this;
}
public String getCommonName() {
return this.commonName;
}
public DescribeClientCertificateResponseBodyCertificate setCountryCode(String countryCode) {
this.countryCode = countryCode;
return this;
}
public String getCountryCode() {
return this.countryCode;
}
public DescribeClientCertificateResponseBodyCertificate setDays(Integer days) {
this.days = days;
return this;
}
public Integer getDays() {
return this.days;
}
public DescribeClientCertificateResponseBodyCertificate setIdentifier(String identifier) {
this.identifier = identifier;
return this;
}
public String getIdentifier() {
return this.identifier;
}
public DescribeClientCertificateResponseBodyCertificate setKeySize(Integer keySize) {
this.keySize = keySize;
return this;
}
public Integer getKeySize() {
return this.keySize;
}
public DescribeClientCertificateResponseBodyCertificate setLocality(String locality) {
this.locality = locality;
return this;
}
public String getLocality() {
return this.locality;
}
public DescribeClientCertificateResponseBodyCertificate setMd5(String md5) {
this.md5 = md5;
return this;
}
public String getMd5() {
return this.md5;
}
public DescribeClientCertificateResponseBodyCertificate setOrganization(String organization) {
this.organization = organization;
return this;
}
public String getOrganization() {
return this.organization;
}
public DescribeClientCertificateResponseBodyCertificate setOrganizationUnit(String organizationUnit) {
this.organizationUnit = organizationUnit;
return this;
}
public String getOrganizationUnit() {
return this.organizationUnit;
}
public DescribeClientCertificateResponseBodyCertificate setParentIdentifier(String parentIdentifier) {
this.parentIdentifier = parentIdentifier;
return this;
}
public String getParentIdentifier() {
return this.parentIdentifier;
}
public DescribeClientCertificateResponseBodyCertificate setSans(String sans) {
this.sans = sans;
return this;
}
public String getSans() {
return this.sans;
}
public DescribeClientCertificateResponseBodyCertificate setSerialNumber(String serialNumber) {
this.serialNumber = serialNumber;
return this;
}
public String getSerialNumber() {
return this.serialNumber;
}
public DescribeClientCertificateResponseBodyCertificate setSha2(String sha2) {
this.sha2 = sha2;
return this;
}
public String getSha2() {
return this.sha2;
}
public DescribeClientCertificateResponseBodyCertificate setSignAlgorithm(String signAlgorithm) {
this.signAlgorithm = signAlgorithm;
return this;
}
public String getSignAlgorithm() {
return this.signAlgorithm;
}
public DescribeClientCertificateResponseBodyCertificate setState(String state) {
this.state = state;
return this;
}
public String getState() {
return this.state;
}
public DescribeClientCertificateResponseBodyCertificate setStatus(String status) {
this.status = status;
return this;
}
public String getStatus() {
return this.status;
}
public DescribeClientCertificateResponseBodyCertificate setSubjectDN(String subjectDN) {
this.subjectDN = subjectDN;
return this;
}
public String getSubjectDN() {
return this.subjectDN;
}
public DescribeClientCertificateResponseBodyCertificate setX509Certificate(String x509Certificate) {
this.x509Certificate = x509Certificate;
return this;
}
public String getX509Certificate() {
return this.x509Certificate;
}
}
}