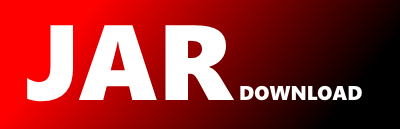
com.aliyun.cs20151215.models.DescribeKubernetesVersionMetadataRequest Maven / Gradle / Ivy
Show all versions of cs20151215 Show documentation
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.cs20151215.models;
import com.aliyun.tea.*;
public class DescribeKubernetesVersionMetadataRequest extends TeaModel {
/**
* The cluster type that you want to use. Valid values:
*
* Kubernetes
: ACK dedicated cluster.
* ManagedKubernetes
: ACK managed cluster. ACK managed clusters include ACK Pro clusters, ACK Basic clusters, ACK Serverless Pro clusters, ACK Serverless Basic clusters, ACK Edge Pro clusters, and ACK Edge Basic clusters.
* ExternalKubernetes
: registered cluster.
*
* This parameter is required.
*
* example:
* Kubernetes
*/
@NameInMap("ClusterType")
public String clusterType;
/**
* The Kubernetes version of the cluster. The Kubernetes versions supported by ACK are the same as the Kubernetes versions supported by open source Kubernetes. We recommend that you specify the latest Kubernetes version. If you do not set this parameter, the latest Kubernetes version is used.
* You can create ACK clusters of the latest two Kubernetes versions in the ACK console. You can call the specific ACK API operation to create clusters of other Kubernetes versions. For more information about the Kubernetes versions supported by ACK, see Release notes for Kubernetes versions.
*
* example:
* 1.16.9-aliyun.1
*/
@NameInMap("KubernetesVersion")
public String kubernetesVersion;
/**
* The query mode. Valid values:
*
* supported
: queries all supported versions.
* creatable
: queries only versions that allow you to create clusters.
*
* If you specify KubernetesVersion
, this parameter does not take effect.
* Default value: creatable.
*
* example:
* supported
*/
@NameInMap("Mode")
public String mode;
/**
* The scenario where clusters are used. Valid values:
*
* Default
: non-edge computing scenarios
* Edge
: edge computing scenarios
* Serverless
: serverless scenarios.
*
* Default value: Default
.
*
* example:
* Default
*/
@NameInMap("Profile")
public String profile;
/**
* Specify whether to query the Kubernetes versions available for updates. This parameter takes effect only when the KubernetesVersion parameter is specified.
*
* example:
* 1.30.1-aliyun.1
*/
@NameInMap("QueryUpgradableVersion")
public Boolean queryUpgradableVersion;
/**
* The region ID of the cluster.
* This parameter is required.
*
* example:
* cn-beijing
*/
@NameInMap("Region")
public String region;
/**
* The container runtime type that you want to use. You can specify a runtime type to query only OS images that support the runtime type. Valid values:
*
* docker
: Docker
* containerd
: containerd
* Sandboxed-Container.runv
: Sandboxed-Container
*
* If you specify a runtime type, only the OS images that support the specified runtime type are returned.
* Otherwise, all OS images are returned.
*
* example:
* docker
*/
@NameInMap("runtime")
public String runtime;
public static DescribeKubernetesVersionMetadataRequest build(java.util.Map map) throws Exception {
DescribeKubernetesVersionMetadataRequest self = new DescribeKubernetesVersionMetadataRequest();
return TeaModel.build(map, self);
}
public DescribeKubernetesVersionMetadataRequest setClusterType(String clusterType) {
this.clusterType = clusterType;
return this;
}
public String getClusterType() {
return this.clusterType;
}
public DescribeKubernetesVersionMetadataRequest setKubernetesVersion(String kubernetesVersion) {
this.kubernetesVersion = kubernetesVersion;
return this;
}
public String getKubernetesVersion() {
return this.kubernetesVersion;
}
public DescribeKubernetesVersionMetadataRequest setMode(String mode) {
this.mode = mode;
return this;
}
public String getMode() {
return this.mode;
}
public DescribeKubernetesVersionMetadataRequest setProfile(String profile) {
this.profile = profile;
return this;
}
public String getProfile() {
return this.profile;
}
public DescribeKubernetesVersionMetadataRequest setQueryUpgradableVersion(Boolean queryUpgradableVersion) {
this.queryUpgradableVersion = queryUpgradableVersion;
return this;
}
public Boolean getQueryUpgradableVersion() {
return this.queryUpgradableVersion;
}
public DescribeKubernetesVersionMetadataRequest setRegion(String region) {
this.region = region;
return this;
}
public String getRegion() {
return this.region;
}
public DescribeKubernetesVersionMetadataRequest setRuntime(String runtime) {
this.runtime = runtime;
return this;
}
public String getRuntime() {
return this.runtime;
}
}