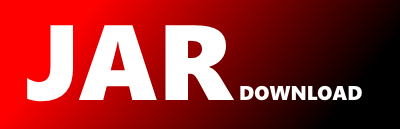
com.aliyun.dataphin_public20230630.models.UpdateBatchTaskRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dataphin_public20230630 Show documentation
Show all versions of dataphin_public20230630 Show documentation
Alibaba Cloud dataphin-public (20230630) SDK for Java
The newest version!
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.dataphin_public20230630.models;
import com.aliyun.tea.*;
public class UpdateBatchTaskRequest extends TeaModel {
/**
* This parameter is required.
*
* example:
* 30001011
*/
@NameInMap("OpTenantId")
public Long opTenantId;
/**
* This parameter is required.
*/
@NameInMap("UpdateCommand")
public UpdateBatchTaskRequestUpdateCommand updateCommand;
public static UpdateBatchTaskRequest build(java.util.Map map) throws Exception {
UpdateBatchTaskRequest self = new UpdateBatchTaskRequest();
return TeaModel.build(map, self);
}
public UpdateBatchTaskRequest setOpTenantId(Long opTenantId) {
this.opTenantId = opTenantId;
return this;
}
public Long getOpTenantId() {
return this.opTenantId;
}
public UpdateBatchTaskRequest setUpdateCommand(UpdateBatchTaskRequestUpdateCommand updateCommand) {
this.updateCommand = updateCommand;
return this;
}
public UpdateBatchTaskRequestUpdateCommand getUpdateCommand() {
return this.updateCommand;
}
public static class UpdateBatchTaskRequestUpdateCommandCustomScheduleConfig extends TeaModel {
/**
* This parameter is required.
*
* example:
* 20:59
*/
@NameInMap("EndTime")
public String endTime;
/**
* This parameter is required.
*
* example:
* 1
*/
@NameInMap("Interval")
public Integer interval;
/**
* This parameter is required.
*
* example:
* HOUR
*/
@NameInMap("IntervalUnit")
public String intervalUnit;
/**
* This parameter is required.
*
* example:
* DAILY
*/
@NameInMap("SchedulePeriod")
public String schedulePeriod;
/**
* This parameter is required.
*
* example:
* 08:00
*/
@NameInMap("StartTime")
public String startTime;
public static UpdateBatchTaskRequestUpdateCommandCustomScheduleConfig build(java.util.Map map) throws Exception {
UpdateBatchTaskRequestUpdateCommandCustomScheduleConfig self = new UpdateBatchTaskRequestUpdateCommandCustomScheduleConfig();
return TeaModel.build(map, self);
}
public UpdateBatchTaskRequestUpdateCommandCustomScheduleConfig setEndTime(String endTime) {
this.endTime = endTime;
return this;
}
public String getEndTime() {
return this.endTime;
}
public UpdateBatchTaskRequestUpdateCommandCustomScheduleConfig setInterval(Integer interval) {
this.interval = interval;
return this;
}
public Integer getInterval() {
return this.interval;
}
public UpdateBatchTaskRequestUpdateCommandCustomScheduleConfig setIntervalUnit(String intervalUnit) {
this.intervalUnit = intervalUnit;
return this;
}
public String getIntervalUnit() {
return this.intervalUnit;
}
public UpdateBatchTaskRequestUpdateCommandCustomScheduleConfig setSchedulePeriod(String schedulePeriod) {
this.schedulePeriod = schedulePeriod;
return this;
}
public String getSchedulePeriod() {
return this.schedulePeriod;
}
public UpdateBatchTaskRequestUpdateCommandCustomScheduleConfig setStartTime(String startTime) {
this.startTime = startTime;
return this;
}
public String getStartTime() {
return this.startTime;
}
}
public static class UpdateBatchTaskRequestUpdateCommandParamList extends TeaModel {
/**
* This parameter is required.
*
* example:
* key
*/
@NameInMap("Key")
public String key;
/**
* This parameter is required.
*
* example:
* value
*/
@NameInMap("Value")
public String value;
public static UpdateBatchTaskRequestUpdateCommandParamList build(java.util.Map map) throws Exception {
UpdateBatchTaskRequestUpdateCommandParamList self = new UpdateBatchTaskRequestUpdateCommandParamList();
return TeaModel.build(map, self);
}
public UpdateBatchTaskRequestUpdateCommandParamList setKey(String key) {
this.key = key;
return this;
}
public String getKey() {
return this.key;
}
public UpdateBatchTaskRequestUpdateCommandParamList setValue(String value) {
this.value = value;
return this;
}
public String getValue() {
return this.value;
}
}
public static class UpdateBatchTaskRequestUpdateCommandSparkClientInfo extends TeaModel {
/**
* This parameter is required.
*
* example:
* abc
*/
@NameInMap("SparkClientVersion")
public String sparkClientVersion;
public static UpdateBatchTaskRequestUpdateCommandSparkClientInfo build(java.util.Map map) throws Exception {
UpdateBatchTaskRequestUpdateCommandSparkClientInfo self = new UpdateBatchTaskRequestUpdateCommandSparkClientInfo();
return TeaModel.build(map, self);
}
public UpdateBatchTaskRequestUpdateCommandSparkClientInfo setSparkClientVersion(String sparkClientVersion) {
this.sparkClientVersion = sparkClientVersion;
return this;
}
public String getSparkClientVersion() {
return this.sparkClientVersion;
}
}
public static class UpdateBatchTaskRequestUpdateCommandUpStreamListDependPeriod extends TeaModel {
/**
* example:
* 1
*/
@NameInMap("PeriodOffset")
public Integer periodOffset;
/**
* This parameter is required.
*
* example:
* CURRENT_PERIOD
*/
@NameInMap("PeriodType")
public String periodType;
public static UpdateBatchTaskRequestUpdateCommandUpStreamListDependPeriod build(java.util.Map map) throws Exception {
UpdateBatchTaskRequestUpdateCommandUpStreamListDependPeriod self = new UpdateBatchTaskRequestUpdateCommandUpStreamListDependPeriod();
return TeaModel.build(map, self);
}
public UpdateBatchTaskRequestUpdateCommandUpStreamListDependPeriod setPeriodOffset(Integer periodOffset) {
this.periodOffset = periodOffset;
return this;
}
public Integer getPeriodOffset() {
return this.periodOffset;
}
public UpdateBatchTaskRequestUpdateCommandUpStreamListDependPeriod setPeriodType(String periodType) {
this.periodType = periodType;
return this;
}
public String getPeriodType() {
return this.periodType;
}
}
public static class UpdateBatchTaskRequestUpdateCommandUpStreamList extends TeaModel {
@NameInMap("DependPeriod")
public UpdateBatchTaskRequestUpdateCommandUpStreamListDependPeriod dependPeriod;
/**
* example:
* LAST
*/
@NameInMap("DependStrategy")
public String dependStrategy;
@NameInMap("FieldList")
public java.util.List fieldList;
/**
* example:
* PHYSICAL
*/
@NameInMap("NodeType")
public String nodeType;
/**
* This parameter is required.
*
* example:
* 1
*/
@NameInMap("PeriodDiff")
public Integer periodDiff;
@NameInMap("SourceNodeEnabled")
public Boolean sourceNodeEnabled;
/**
* example:
* n_2001
*/
@NameInMap("SourceNodeId")
public String sourceNodeId;
/**
* This parameter is required.
*
* example:
* t_input1
*/
@NameInMap("SourceNodeOutputName")
public String sourceNodeOutputName;
/**
* example:
* t_input1
*/
@NameInMap("SourceTableName")
public String sourceTableName;
public static UpdateBatchTaskRequestUpdateCommandUpStreamList build(java.util.Map map) throws Exception {
UpdateBatchTaskRequestUpdateCommandUpStreamList self = new UpdateBatchTaskRequestUpdateCommandUpStreamList();
return TeaModel.build(map, self);
}
public UpdateBatchTaskRequestUpdateCommandUpStreamList setDependPeriod(UpdateBatchTaskRequestUpdateCommandUpStreamListDependPeriod dependPeriod) {
this.dependPeriod = dependPeriod;
return this;
}
public UpdateBatchTaskRequestUpdateCommandUpStreamListDependPeriod getDependPeriod() {
return this.dependPeriod;
}
public UpdateBatchTaskRequestUpdateCommandUpStreamList setDependStrategy(String dependStrategy) {
this.dependStrategy = dependStrategy;
return this;
}
public String getDependStrategy() {
return this.dependStrategy;
}
public UpdateBatchTaskRequestUpdateCommandUpStreamList setFieldList(java.util.List fieldList) {
this.fieldList = fieldList;
return this;
}
public java.util.List getFieldList() {
return this.fieldList;
}
public UpdateBatchTaskRequestUpdateCommandUpStreamList setNodeType(String nodeType) {
this.nodeType = nodeType;
return this;
}
public String getNodeType() {
return this.nodeType;
}
public UpdateBatchTaskRequestUpdateCommandUpStreamList setPeriodDiff(Integer periodDiff) {
this.periodDiff = periodDiff;
return this;
}
public Integer getPeriodDiff() {
return this.periodDiff;
}
public UpdateBatchTaskRequestUpdateCommandUpStreamList setSourceNodeEnabled(Boolean sourceNodeEnabled) {
this.sourceNodeEnabled = sourceNodeEnabled;
return this;
}
public Boolean getSourceNodeEnabled() {
return this.sourceNodeEnabled;
}
public UpdateBatchTaskRequestUpdateCommandUpStreamList setSourceNodeId(String sourceNodeId) {
this.sourceNodeId = sourceNodeId;
return this;
}
public String getSourceNodeId() {
return this.sourceNodeId;
}
public UpdateBatchTaskRequestUpdateCommandUpStreamList setSourceNodeOutputName(String sourceNodeOutputName) {
this.sourceNodeOutputName = sourceNodeOutputName;
return this;
}
public String getSourceNodeOutputName() {
return this.sourceNodeOutputName;
}
public UpdateBatchTaskRequestUpdateCommandUpStreamList setSourceTableName(String sourceTableName) {
this.sourceTableName = sourceTableName;
return this;
}
public String getSourceTableName() {
return this.sourceTableName;
}
}
public static class UpdateBatchTaskRequestUpdateCommand extends TeaModel {
/**
* This parameter is required.
*
* example:
* show tables;
*/
@NameInMap("Code")
public String code;
/**
* example:
* 0 0 1 * * ?
*/
@NameInMap("CronExpression")
public String cronExpression;
@NameInMap("CustomScheduleConfig")
public UpdateBatchTaskRequestUpdateCommandCustomScheduleConfig customScheduleConfig;
/**
* example:
* mysql_catalog
*/
@NameInMap("DataSourceCatalog")
public String dataSourceCatalog;
/**
* example:
* 12131111
*/
@NameInMap("DataSourceId")
public String dataSourceId;
/**
* example:
* erp
*/
@NameInMap("DataSourceSchema")
public String dataSourceSchema;
/**
* example:
* PYTHON3_7
*/
@NameInMap("Engine")
public String engine;
/**
* This parameter is required.
*
* example:
* 12113111
*/
@NameInMap("FileId")
public Long fileId;
/**
* This parameter is required.
*
* example:
* test111
*/
@NameInMap("Name")
public String name;
/**
* example:
* xx测试
*/
@NameInMap("NodeDescription")
public String nodeDescription;
@NameInMap("NodeOutputNameList")
public java.util.List nodeOutputNameList;
/**
* example:
* 1
*/
@NameInMap("NodeStatus")
public Integer nodeStatus;
@NameInMap("ParamList")
public java.util.List paramList;
/**
* example:
* 1
*/
@NameInMap("Priority")
public Integer priority;
/**
* This parameter is required.
*
* example:
* 10121101
*/
@NameInMap("ProjectId")
public Long projectId;
@NameInMap("PythonModuleList")
public java.util.List pythonModuleList;
/**
* example:
* DAILY
*/
@NameInMap("SchedulePeriod")
public String schedulePeriod;
@NameInMap("SparkClientInfo")
public UpdateBatchTaskRequestUpdateCommandSparkClientInfo sparkClientInfo;
/**
* This parameter is required.
*
* example:
* 21
*/
@NameInMap("TaskType")
public Integer taskType;
@NameInMap("UpStreamList")
public java.util.List upStreamList;
public static UpdateBatchTaskRequestUpdateCommand build(java.util.Map map) throws Exception {
UpdateBatchTaskRequestUpdateCommand self = new UpdateBatchTaskRequestUpdateCommand();
return TeaModel.build(map, self);
}
public UpdateBatchTaskRequestUpdateCommand setCode(String code) {
this.code = code;
return this;
}
public String getCode() {
return this.code;
}
public UpdateBatchTaskRequestUpdateCommand setCronExpression(String cronExpression) {
this.cronExpression = cronExpression;
return this;
}
public String getCronExpression() {
return this.cronExpression;
}
public UpdateBatchTaskRequestUpdateCommand setCustomScheduleConfig(UpdateBatchTaskRequestUpdateCommandCustomScheduleConfig customScheduleConfig) {
this.customScheduleConfig = customScheduleConfig;
return this;
}
public UpdateBatchTaskRequestUpdateCommandCustomScheduleConfig getCustomScheduleConfig() {
return this.customScheduleConfig;
}
public UpdateBatchTaskRequestUpdateCommand setDataSourceCatalog(String dataSourceCatalog) {
this.dataSourceCatalog = dataSourceCatalog;
return this;
}
public String getDataSourceCatalog() {
return this.dataSourceCatalog;
}
public UpdateBatchTaskRequestUpdateCommand setDataSourceId(String dataSourceId) {
this.dataSourceId = dataSourceId;
return this;
}
public String getDataSourceId() {
return this.dataSourceId;
}
public UpdateBatchTaskRequestUpdateCommand setDataSourceSchema(String dataSourceSchema) {
this.dataSourceSchema = dataSourceSchema;
return this;
}
public String getDataSourceSchema() {
return this.dataSourceSchema;
}
public UpdateBatchTaskRequestUpdateCommand setEngine(String engine) {
this.engine = engine;
return this;
}
public String getEngine() {
return this.engine;
}
public UpdateBatchTaskRequestUpdateCommand setFileId(Long fileId) {
this.fileId = fileId;
return this;
}
public Long getFileId() {
return this.fileId;
}
public UpdateBatchTaskRequestUpdateCommand setName(String name) {
this.name = name;
return this;
}
public String getName() {
return this.name;
}
public UpdateBatchTaskRequestUpdateCommand setNodeDescription(String nodeDescription) {
this.nodeDescription = nodeDescription;
return this;
}
public String getNodeDescription() {
return this.nodeDescription;
}
public UpdateBatchTaskRequestUpdateCommand setNodeOutputNameList(java.util.List nodeOutputNameList) {
this.nodeOutputNameList = nodeOutputNameList;
return this;
}
public java.util.List getNodeOutputNameList() {
return this.nodeOutputNameList;
}
public UpdateBatchTaskRequestUpdateCommand setNodeStatus(Integer nodeStatus) {
this.nodeStatus = nodeStatus;
return this;
}
public Integer getNodeStatus() {
return this.nodeStatus;
}
public UpdateBatchTaskRequestUpdateCommand setParamList(java.util.List paramList) {
this.paramList = paramList;
return this;
}
public java.util.List getParamList() {
return this.paramList;
}
public UpdateBatchTaskRequestUpdateCommand setPriority(Integer priority) {
this.priority = priority;
return this;
}
public Integer getPriority() {
return this.priority;
}
public UpdateBatchTaskRequestUpdateCommand setProjectId(Long projectId) {
this.projectId = projectId;
return this;
}
public Long getProjectId() {
return this.projectId;
}
public UpdateBatchTaskRequestUpdateCommand setPythonModuleList(java.util.List pythonModuleList) {
this.pythonModuleList = pythonModuleList;
return this;
}
public java.util.List getPythonModuleList() {
return this.pythonModuleList;
}
public UpdateBatchTaskRequestUpdateCommand setSchedulePeriod(String schedulePeriod) {
this.schedulePeriod = schedulePeriod;
return this;
}
public String getSchedulePeriod() {
return this.schedulePeriod;
}
public UpdateBatchTaskRequestUpdateCommand setSparkClientInfo(UpdateBatchTaskRequestUpdateCommandSparkClientInfo sparkClientInfo) {
this.sparkClientInfo = sparkClientInfo;
return this;
}
public UpdateBatchTaskRequestUpdateCommandSparkClientInfo getSparkClientInfo() {
return this.sparkClientInfo;
}
public UpdateBatchTaskRequestUpdateCommand setTaskType(Integer taskType) {
this.taskType = taskType;
return this;
}
public Integer getTaskType() {
return this.taskType;
}
public UpdateBatchTaskRequestUpdateCommand setUpStreamList(java.util.List upStreamList) {
this.upStreamList = upStreamList;
return this;
}
public java.util.List getUpStreamList() {
return this.upStreamList;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy