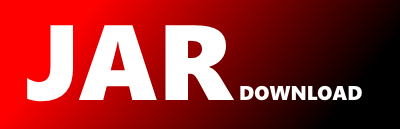
com.aliyun.dingtalkalitrip_1_0.models.GetHotelExceedApplyResponseBody Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dingtalk Show documentation
Show all versions of dingtalk Show documentation
Alibaba Cloud dingtalk SDK for Java
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.dingtalkalitrip_1_0.models;
import com.aliyun.tea.*;
public class GetHotelExceedApplyResponseBody extends TeaModel {
/**
* This parameter is required.
*
* example:
* 12345
*/
@NameInMap("applyId")
public Long applyId;
/**
* This parameter is required.
*/
@NameInMap("applyIntentionInfoDO")
public GetHotelExceedApplyResponseBodyApplyIntentionInfoDO applyIntentionInfoDO;
/**
* This parameter is required.
*
* example:
* 出差
*/
@NameInMap("btripCause")
public String btripCause;
/**
* This parameter is required.
*
* example:
* ding12345
*/
@NameInMap("corpId")
public String corpId;
/**
* This parameter is required.
*
* example:
* 出差
*/
@NameInMap("exceedReason")
public String exceedReason;
/**
* This parameter is required.
*
* example:
* 16
*/
@NameInMap("exceedType")
public Integer exceedType;
/**
* This parameter is required.
*
* example:
* 210000
*/
@NameInMap("originStandard")
public String originStandard;
/**
* This parameter is required.
*
* example:
* 0
*/
@NameInMap("status")
public Integer status;
/**
* This parameter is required.
*
* example:
* 2021-07-08 15:23:56
*/
@NameInMap("submitTime")
public String submitTime;
/**
* This parameter is required.
*
* example:
* 0001A1100000007EX08O
*/
@NameInMap("thirdpartApplyId")
public String thirdpartApplyId;
/**
* This parameter is required.
*
* example:
* weifeng
*/
@NameInMap("userId")
public String userId;
public static GetHotelExceedApplyResponseBody build(java.util.Map map) throws Exception {
GetHotelExceedApplyResponseBody self = new GetHotelExceedApplyResponseBody();
return TeaModel.build(map, self);
}
public GetHotelExceedApplyResponseBody setApplyId(Long applyId) {
this.applyId = applyId;
return this;
}
public Long getApplyId() {
return this.applyId;
}
public GetHotelExceedApplyResponseBody setApplyIntentionInfoDO(GetHotelExceedApplyResponseBodyApplyIntentionInfoDO applyIntentionInfoDO) {
this.applyIntentionInfoDO = applyIntentionInfoDO;
return this;
}
public GetHotelExceedApplyResponseBodyApplyIntentionInfoDO getApplyIntentionInfoDO() {
return this.applyIntentionInfoDO;
}
public GetHotelExceedApplyResponseBody setBtripCause(String btripCause) {
this.btripCause = btripCause;
return this;
}
public String getBtripCause() {
return this.btripCause;
}
public GetHotelExceedApplyResponseBody setCorpId(String corpId) {
this.corpId = corpId;
return this;
}
public String getCorpId() {
return this.corpId;
}
public GetHotelExceedApplyResponseBody setExceedReason(String exceedReason) {
this.exceedReason = exceedReason;
return this;
}
public String getExceedReason() {
return this.exceedReason;
}
public GetHotelExceedApplyResponseBody setExceedType(Integer exceedType) {
this.exceedType = exceedType;
return this;
}
public Integer getExceedType() {
return this.exceedType;
}
public GetHotelExceedApplyResponseBody setOriginStandard(String originStandard) {
this.originStandard = originStandard;
return this;
}
public String getOriginStandard() {
return this.originStandard;
}
public GetHotelExceedApplyResponseBody setStatus(Integer status) {
this.status = status;
return this;
}
public Integer getStatus() {
return this.status;
}
public GetHotelExceedApplyResponseBody setSubmitTime(String submitTime) {
this.submitTime = submitTime;
return this;
}
public String getSubmitTime() {
return this.submitTime;
}
public GetHotelExceedApplyResponseBody setThirdpartApplyId(String thirdpartApplyId) {
this.thirdpartApplyId = thirdpartApplyId;
return this;
}
public String getThirdpartApplyId() {
return this.thirdpartApplyId;
}
public GetHotelExceedApplyResponseBody setUserId(String userId) {
this.userId = userId;
return this;
}
public String getUserId() {
return this.userId;
}
public static class GetHotelExceedApplyResponseBodyApplyIntentionInfoDO extends TeaModel {
/**
* This parameter is required.
*
* example:
* 2021-07-08
*/
@NameInMap("checkIn")
public String checkIn;
/**
* This parameter is required.
*
* example:
* 2021-07-08
*/
@NameInMap("checkOut")
public String checkOut;
/**
* This parameter is required.
*
* example:
* SHA
*/
@NameInMap("cityCode")
public String cityCode;
/**
* This parameter is required.
*
* example:
* 上海
*/
@NameInMap("cityName")
public String cityName;
/**
* This parameter is required.
*
* example:
* 10000
*/
@NameInMap("price")
public Long price;
/**
* This parameter is required.
*
* example:
* true
*/
@NameInMap("together")
public Boolean together;
/**
* This parameter is required.
*
* example:
* 16
*/
@NameInMap("type")
public Integer type;
public static GetHotelExceedApplyResponseBodyApplyIntentionInfoDO build(java.util.Map map) throws Exception {
GetHotelExceedApplyResponseBodyApplyIntentionInfoDO self = new GetHotelExceedApplyResponseBodyApplyIntentionInfoDO();
return TeaModel.build(map, self);
}
public GetHotelExceedApplyResponseBodyApplyIntentionInfoDO setCheckIn(String checkIn) {
this.checkIn = checkIn;
return this;
}
public String getCheckIn() {
return this.checkIn;
}
public GetHotelExceedApplyResponseBodyApplyIntentionInfoDO setCheckOut(String checkOut) {
this.checkOut = checkOut;
return this;
}
public String getCheckOut() {
return this.checkOut;
}
public GetHotelExceedApplyResponseBodyApplyIntentionInfoDO setCityCode(String cityCode) {
this.cityCode = cityCode;
return this;
}
public String getCityCode() {
return this.cityCode;
}
public GetHotelExceedApplyResponseBodyApplyIntentionInfoDO setCityName(String cityName) {
this.cityName = cityName;
return this;
}
public String getCityName() {
return this.cityName;
}
public GetHotelExceedApplyResponseBodyApplyIntentionInfoDO setPrice(Long price) {
this.price = price;
return this;
}
public Long getPrice() {
return this.price;
}
public GetHotelExceedApplyResponseBodyApplyIntentionInfoDO setTogether(Boolean together) {
this.together = together;
return this;
}
public Boolean getTogether() {
return this.together;
}
public GetHotelExceedApplyResponseBodyApplyIntentionInfoDO setType(Integer type) {
this.type = type;
return this;
}
public Integer getType() {
return this.type;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy