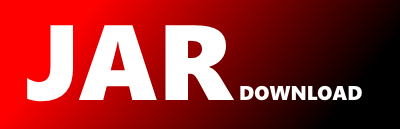
com.aliyun.dingtalkapaas_1_0.models.BatchQueryByTemplateKeyResponseBody Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dingtalk Show documentation
Show all versions of dingtalk Show documentation
Alibaba Cloud dingtalk SDK for Java
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.dingtalkapaas_1_0.models;
import com.aliyun.tea.*;
public class BatchQueryByTemplateKeyResponseBody extends TeaModel {
/**
* This parameter is required.
*/
@NameInMap("templateList")
public java.util.List templateList;
public static BatchQueryByTemplateKeyResponseBody build(java.util.Map map) throws Exception {
BatchQueryByTemplateKeyResponseBody self = new BatchQueryByTemplateKeyResponseBody();
return TeaModel.build(map, self);
}
public BatchQueryByTemplateKeyResponseBody setTemplateList(java.util.List templateList) {
this.templateList = templateList;
return this;
}
public java.util.List getTemplateList() {
return this.templateList;
}
public static class BatchQueryByTemplateKeyResponseBodyTemplateList extends TeaModel {
/**
* This parameter is required.
*/
@NameInMap("adaptEnv")
public java.util.List adaptEnv;
/**
* This parameter is required.
*
* example:
* 测试用
*/
@NameInMap("appDesc")
public String appDesc;
/**
* This parameter is required.
*
* example:
* @lALPDe7s2JOuoyjNBaDNCgA
*/
@NameInMap("appIcon")
public String appIcon;
/**
* This parameter is required.
*/
@NameInMap("caseVideoList")
public java.util.List caseVideoList;
/**
* This parameter is required.
*
* example:
* template_category
*/
@NameInMap("category")
public String category;
/**
* This parameter is required.
*/
@NameInMap("coverImgList")
public java.util.List coverImgList;
/**
* This parameter is required.
*
* example:
*
*/
@NameInMap("expUrl")
public String expUrl;
/**
* This parameter is required.
*/
@NameInMap("industryLabelList")
public java.util.List industryLabelList;
/**
* This parameter is required.
*
* example:
* 100
*/
@NameInMap("installTimes")
public Float installTimes;
/**
* This parameter is required.
*/
@NameInMap("mobilePreviewMediaList")
public java.util.List mobilePreviewMediaList;
/**
* This parameter is required.
*
* example:
* 测试用
*/
@NameInMap("name")
public String name;
/**
* This parameter is required.
*/
@NameInMap("previewMediaList")
public java.util.List previewMediaList;
/**
* This parameter is required.
*
* example:
* 小明
*/
@NameInMap("providerName")
public String providerName;
/**
* This parameter is required.
*/
@NameInMap("roleLabelList")
public java.util.List roleLabelList;
/**
* This parameter is required.
*
* example:
* 测试用
*/
@NameInMap("simpleDesc")
public String simpleDesc;
/**
* This parameter is required.
*
* example:
* template_key
*/
@NameInMap("templateKey")
public String templateKey;
/**
* This parameter is required.
*/
@NameInMap("useCasesMediaList")
public java.util.List useCasesMediaList;
public static BatchQueryByTemplateKeyResponseBodyTemplateList build(java.util.Map map) throws Exception {
BatchQueryByTemplateKeyResponseBodyTemplateList self = new BatchQueryByTemplateKeyResponseBodyTemplateList();
return TeaModel.build(map, self);
}
public BatchQueryByTemplateKeyResponseBodyTemplateList setAdaptEnv(java.util.List adaptEnv) {
this.adaptEnv = adaptEnv;
return this;
}
public java.util.List getAdaptEnv() {
return this.adaptEnv;
}
public BatchQueryByTemplateKeyResponseBodyTemplateList setAppDesc(String appDesc) {
this.appDesc = appDesc;
return this;
}
public String getAppDesc() {
return this.appDesc;
}
public BatchQueryByTemplateKeyResponseBodyTemplateList setAppIcon(String appIcon) {
this.appIcon = appIcon;
return this;
}
public String getAppIcon() {
return this.appIcon;
}
public BatchQueryByTemplateKeyResponseBodyTemplateList setCaseVideoList(java.util.List caseVideoList) {
this.caseVideoList = caseVideoList;
return this;
}
public java.util.List getCaseVideoList() {
return this.caseVideoList;
}
public BatchQueryByTemplateKeyResponseBodyTemplateList setCategory(String category) {
this.category = category;
return this;
}
public String getCategory() {
return this.category;
}
public BatchQueryByTemplateKeyResponseBodyTemplateList setCoverImgList(java.util.List coverImgList) {
this.coverImgList = coverImgList;
return this;
}
public java.util.List getCoverImgList() {
return this.coverImgList;
}
public BatchQueryByTemplateKeyResponseBodyTemplateList setExpUrl(String expUrl) {
this.expUrl = expUrl;
return this;
}
public String getExpUrl() {
return this.expUrl;
}
public BatchQueryByTemplateKeyResponseBodyTemplateList setIndustryLabelList(java.util.List industryLabelList) {
this.industryLabelList = industryLabelList;
return this;
}
public java.util.List getIndustryLabelList() {
return this.industryLabelList;
}
public BatchQueryByTemplateKeyResponseBodyTemplateList setInstallTimes(Float installTimes) {
this.installTimes = installTimes;
return this;
}
public Float getInstallTimes() {
return this.installTimes;
}
public BatchQueryByTemplateKeyResponseBodyTemplateList setMobilePreviewMediaList(java.util.List mobilePreviewMediaList) {
this.mobilePreviewMediaList = mobilePreviewMediaList;
return this;
}
public java.util.List getMobilePreviewMediaList() {
return this.mobilePreviewMediaList;
}
public BatchQueryByTemplateKeyResponseBodyTemplateList setName(String name) {
this.name = name;
return this;
}
public String getName() {
return this.name;
}
public BatchQueryByTemplateKeyResponseBodyTemplateList setPreviewMediaList(java.util.List previewMediaList) {
this.previewMediaList = previewMediaList;
return this;
}
public java.util.List getPreviewMediaList() {
return this.previewMediaList;
}
public BatchQueryByTemplateKeyResponseBodyTemplateList setProviderName(String providerName) {
this.providerName = providerName;
return this;
}
public String getProviderName() {
return this.providerName;
}
public BatchQueryByTemplateKeyResponseBodyTemplateList setRoleLabelList(java.util.List roleLabelList) {
this.roleLabelList = roleLabelList;
return this;
}
public java.util.List getRoleLabelList() {
return this.roleLabelList;
}
public BatchQueryByTemplateKeyResponseBodyTemplateList setSimpleDesc(String simpleDesc) {
this.simpleDesc = simpleDesc;
return this;
}
public String getSimpleDesc() {
return this.simpleDesc;
}
public BatchQueryByTemplateKeyResponseBodyTemplateList setTemplateKey(String templateKey) {
this.templateKey = templateKey;
return this;
}
public String getTemplateKey() {
return this.templateKey;
}
public BatchQueryByTemplateKeyResponseBodyTemplateList setUseCasesMediaList(java.util.List useCasesMediaList) {
this.useCasesMediaList = useCasesMediaList;
return this;
}
public java.util.List getUseCasesMediaList() {
return this.useCasesMediaList;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy