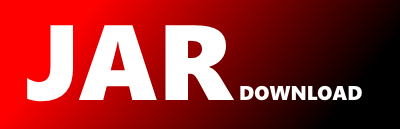
com.aliyun.dingtalkconv_file_1_0.Client Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dingtalk Show documentation
Show all versions of dingtalk Show documentation
Alibaba Cloud dingtalk SDK for Java
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.dingtalkconv_file_1_0;
import com.aliyun.tea.*;
import com.aliyun.dingtalkconv_file_1_0.models.*;
public class Client extends com.aliyun.teaopenapi.Client {
public Client(com.aliyun.teaopenapi.models.Config config) throws Exception {
super(config);
com.aliyun.gateway.dingtalk.Client gatewayClient = new com.aliyun.gateway.dingtalk.Client();
this._spi = gatewayClient;
this._endpointRule = "";
if (com.aliyun.teautil.Common.empty(_endpoint)) {
this._endpoint = "api.dingtalk.com";
}
}
/**
* summary :
* 获取IM会话存储空间信息
*
* @param request GetSpaceRequest
* @param headers GetSpaceHeaders
* @param runtime runtime options for this request RuntimeOptions
* @return GetSpaceResponse
*/
public GetSpaceResponse getSpaceWithOptions(GetSpaceRequest request, GetSpaceHeaders headers, com.aliyun.teautil.models.RuntimeOptions runtime) throws Exception {
com.aliyun.teautil.Common.validateModel(request);
java.util.Map query = new java.util.HashMap<>();
if (!com.aliyun.teautil.Common.isUnset(request.unionId)) {
query.put("unionId", request.unionId);
}
java.util.Map body = new java.util.HashMap<>();
if (!com.aliyun.teautil.Common.isUnset(request.openConversationId)) {
body.put("openConversationId", request.openConversationId);
}
java.util.Map realHeaders = new java.util.HashMap<>();
if (!com.aliyun.teautil.Common.isUnset(headers.commonHeaders)) {
realHeaders = headers.commonHeaders;
}
if (!com.aliyun.teautil.Common.isUnset(headers.xAcsDingtalkAccessToken)) {
realHeaders.put("x-acs-dingtalk-access-token", com.aliyun.teautil.Common.toJSONString(headers.xAcsDingtalkAccessToken));
}
com.aliyun.teaopenapi.models.OpenApiRequest req = com.aliyun.teaopenapi.models.OpenApiRequest.build(TeaConverter.buildMap(
new TeaPair("headers", realHeaders),
new TeaPair("query", com.aliyun.openapiutil.Client.query(query)),
new TeaPair("body", com.aliyun.openapiutil.Client.parseToMap(body))
));
com.aliyun.teaopenapi.models.Params params = com.aliyun.teaopenapi.models.Params.build(TeaConverter.buildMap(
new TeaPair("action", "GetSpace"),
new TeaPair("version", "convFile_1.0"),
new TeaPair("protocol", "HTTP"),
new TeaPair("pathname", "/v1.0/convFile/conversations/spaces/query"),
new TeaPair("method", "POST"),
new TeaPair("authType", "AK"),
new TeaPair("style", "ROA"),
new TeaPair("reqBodyType", "none"),
new TeaPair("bodyType", "json")
));
return TeaModel.toModel(this.execute(params, req, runtime), new GetSpaceResponse());
}
/**
* summary :
* 获取IM会话存储空间信息
*
* @param request GetSpaceRequest
* @return GetSpaceResponse
*/
public GetSpaceResponse getSpace(GetSpaceRequest request) throws Exception {
com.aliyun.teautil.models.RuntimeOptions runtime = new com.aliyun.teautil.models.RuntimeOptions();
GetSpaceHeaders headers = new GetSpaceHeaders();
return this.getSpaceWithOptions(request, headers, runtime);
}
/**
* summary :
* 发送文件到指定会话
*
* @param request SendRequest
* @param headers SendHeaders
* @param runtime runtime options for this request RuntimeOptions
* @return SendResponse
*/
public SendResponse sendWithOptions(SendRequest request, SendHeaders headers, com.aliyun.teautil.models.RuntimeOptions runtime) throws Exception {
com.aliyun.teautil.Common.validateModel(request);
java.util.Map query = new java.util.HashMap<>();
if (!com.aliyun.teautil.Common.isUnset(request.unionId)) {
query.put("unionId", request.unionId);
}
java.util.Map body = new java.util.HashMap<>();
if (!com.aliyun.teautil.Common.isUnset(request.dentryId)) {
body.put("dentryId", request.dentryId);
}
if (!com.aliyun.teautil.Common.isUnset(request.openConversationId)) {
body.put("openConversationId", request.openConversationId);
}
if (!com.aliyun.teautil.Common.isUnset(request.spaceId)) {
body.put("spaceId", request.spaceId);
}
java.util.Map realHeaders = new java.util.HashMap<>();
if (!com.aliyun.teautil.Common.isUnset(headers.commonHeaders)) {
realHeaders = headers.commonHeaders;
}
if (!com.aliyun.teautil.Common.isUnset(headers.xAcsDingtalkAccessToken)) {
realHeaders.put("x-acs-dingtalk-access-token", com.aliyun.teautil.Common.toJSONString(headers.xAcsDingtalkAccessToken));
}
com.aliyun.teaopenapi.models.OpenApiRequest req = com.aliyun.teaopenapi.models.OpenApiRequest.build(TeaConverter.buildMap(
new TeaPair("headers", realHeaders),
new TeaPair("query", com.aliyun.openapiutil.Client.query(query)),
new TeaPair("body", com.aliyun.openapiutil.Client.parseToMap(body))
));
com.aliyun.teaopenapi.models.Params params = com.aliyun.teaopenapi.models.Params.build(TeaConverter.buildMap(
new TeaPair("action", "Send"),
new TeaPair("version", "convFile_1.0"),
new TeaPair("protocol", "HTTP"),
new TeaPair("pathname", "/v1.0/convFile/conversations/files/send"),
new TeaPair("method", "POST"),
new TeaPair("authType", "AK"),
new TeaPair("style", "ROA"),
new TeaPair("reqBodyType", "none"),
new TeaPair("bodyType", "json")
));
return TeaModel.toModel(this.execute(params, req, runtime), new SendResponse());
}
/**
* summary :
* 发送文件到指定会话
*
* @param request SendRequest
* @return SendResponse
*/
public SendResponse send(SendRequest request) throws Exception {
com.aliyun.teautil.models.RuntimeOptions runtime = new com.aliyun.teautil.models.RuntimeOptions();
SendHeaders headers = new SendHeaders();
return this.sendWithOptions(request, headers, runtime);
}
/**
* summary :
* 以应用身份发送文件给自己
*
* @param request SendByAppRequest
* @param headers SendByAppHeaders
* @param runtime runtime options for this request RuntimeOptions
* @return SendByAppResponse
*/
public SendByAppResponse sendByAppWithOptions(SendByAppRequest request, SendByAppHeaders headers, com.aliyun.teautil.models.RuntimeOptions runtime) throws Exception {
com.aliyun.teautil.Common.validateModel(request);
java.util.Map query = new java.util.HashMap<>();
if (!com.aliyun.teautil.Common.isUnset(request.unionId)) {
query.put("unionId", request.unionId);
}
java.util.Map body = new java.util.HashMap<>();
if (!com.aliyun.teautil.Common.isUnset(request.dentryId)) {
body.put("dentryId", request.dentryId);
}
if (!com.aliyun.teautil.Common.isUnset(request.spaceId)) {
body.put("spaceId", request.spaceId);
}
java.util.Map realHeaders = new java.util.HashMap<>();
if (!com.aliyun.teautil.Common.isUnset(headers.commonHeaders)) {
realHeaders = headers.commonHeaders;
}
if (!com.aliyun.teautil.Common.isUnset(headers.xAcsDingtalkAccessToken)) {
realHeaders.put("x-acs-dingtalk-access-token", com.aliyun.teautil.Common.toJSONString(headers.xAcsDingtalkAccessToken));
}
com.aliyun.teaopenapi.models.OpenApiRequest req = com.aliyun.teaopenapi.models.OpenApiRequest.build(TeaConverter.buildMap(
new TeaPair("headers", realHeaders),
new TeaPair("query", com.aliyun.openapiutil.Client.query(query)),
new TeaPair("body", com.aliyun.openapiutil.Client.parseToMap(body))
));
com.aliyun.teaopenapi.models.Params params = com.aliyun.teaopenapi.models.Params.build(TeaConverter.buildMap(
new TeaPair("action", "SendByApp"),
new TeaPair("version", "convFile_1.0"),
new TeaPair("protocol", "HTTP"),
new TeaPair("pathname", "/v1.0/convFile/apps/conversations/files/send"),
new TeaPair("method", "POST"),
new TeaPair("authType", "AK"),
new TeaPair("style", "ROA"),
new TeaPair("reqBodyType", "none"),
new TeaPair("bodyType", "json")
));
return TeaModel.toModel(this.execute(params, req, runtime), new SendByAppResponse());
}
/**
* summary :
* 以应用身份发送文件给自己
*
* @param request SendByAppRequest
* @return SendByAppResponse
*/
public SendByAppResponse sendByApp(SendByAppRequest request) throws Exception {
com.aliyun.teautil.models.RuntimeOptions runtime = new com.aliyun.teautil.models.RuntimeOptions();
SendByAppHeaders headers = new SendByAppHeaders();
return this.sendByAppWithOptions(request, headers, runtime);
}
/**
* summary :
* 发送文件链接到指定会话
*
* @param request SendLinkRequest
* @param headers SendLinkHeaders
* @param runtime runtime options for this request RuntimeOptions
* @return SendLinkResponse
*/
public SendLinkResponse sendLinkWithOptions(SendLinkRequest request, SendLinkHeaders headers, com.aliyun.teautil.models.RuntimeOptions runtime) throws Exception {
com.aliyun.teautil.Common.validateModel(request);
java.util.Map query = new java.util.HashMap<>();
if (!com.aliyun.teautil.Common.isUnset(request.unionId)) {
query.put("unionId", request.unionId);
}
java.util.Map body = new java.util.HashMap<>();
if (!com.aliyun.teautil.Common.isUnset(request.dentryId)) {
body.put("dentryId", request.dentryId);
}
if (!com.aliyun.teautil.Common.isUnset(request.openConversationId)) {
body.put("openConversationId", request.openConversationId);
}
if (!com.aliyun.teautil.Common.isUnset(request.spaceId)) {
body.put("spaceId", request.spaceId);
}
java.util.Map realHeaders = new java.util.HashMap<>();
if (!com.aliyun.teautil.Common.isUnset(headers.commonHeaders)) {
realHeaders = headers.commonHeaders;
}
if (!com.aliyun.teautil.Common.isUnset(headers.xAcsDingtalkAccessToken)) {
realHeaders.put("x-acs-dingtalk-access-token", com.aliyun.teautil.Common.toJSONString(headers.xAcsDingtalkAccessToken));
}
com.aliyun.teaopenapi.models.OpenApiRequest req = com.aliyun.teaopenapi.models.OpenApiRequest.build(TeaConverter.buildMap(
new TeaPair("headers", realHeaders),
new TeaPair("query", com.aliyun.openapiutil.Client.query(query)),
new TeaPair("body", com.aliyun.openapiutil.Client.parseToMap(body))
));
com.aliyun.teaopenapi.models.Params params = com.aliyun.teaopenapi.models.Params.build(TeaConverter.buildMap(
new TeaPair("action", "SendLink"),
new TeaPair("version", "convFile_1.0"),
new TeaPair("protocol", "HTTP"),
new TeaPair("pathname", "/v1.0/convFile/conversations/files/links/send"),
new TeaPair("method", "POST"),
new TeaPair("authType", "AK"),
new TeaPair("style", "ROA"),
new TeaPair("reqBodyType", "none"),
new TeaPair("bodyType", "json")
));
return TeaModel.toModel(this.execute(params, req, runtime), new SendLinkResponse());
}
/**
* summary :
* 发送文件链接到指定会话
*
* @param request SendLinkRequest
* @return SendLinkResponse
*/
public SendLinkResponse sendLink(SendLinkRequest request) throws Exception {
com.aliyun.teautil.models.RuntimeOptions runtime = new com.aliyun.teautil.models.RuntimeOptions();
SendLinkHeaders headers = new SendLinkHeaders();
return this.sendLinkWithOptions(request, headers, runtime);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy