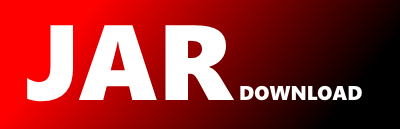
com.aliyun.dingtalkedu_1_0.models.CardBatchQueryCardsResponseBody Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dingtalk Show documentation
Show all versions of dingtalk Show documentation
Alibaba Cloud dingtalk SDK for Java
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.dingtalkedu_1_0.models;
import com.aliyun.tea.*;
public class CardBatchQueryCardsResponseBody extends TeaModel {
@NameInMap("result")
public CardBatchQueryCardsResponseBodyResult result;
@NameInMap("success")
public Boolean success;
public static CardBatchQueryCardsResponseBody build(java.util.Map map) throws Exception {
CardBatchQueryCardsResponseBody self = new CardBatchQueryCardsResponseBody();
return TeaModel.build(map, self);
}
public CardBatchQueryCardsResponseBody setResult(CardBatchQueryCardsResponseBodyResult result) {
this.result = result;
return this;
}
public CardBatchQueryCardsResponseBodyResult getResult() {
return this.result;
}
public CardBatchQueryCardsResponseBody setSuccess(Boolean success) {
this.success = success;
return this;
}
public Boolean getSuccess() {
return this.success;
}
public static class CardBatchQueryCardsResponseBodyResultCards extends TeaModel {
/**
* example:
* industry_center
*/
@NameInMap("cardBizCode")
public String cardBizCode;
/**
* example:
* 234234234
*/
@NameInMap("cardId")
public Long cardId;
/**
* example:
* 2
*/
@NameInMap("cardStatus")
public Integer cardStatus;
/**
* example:
* 打卡任务
*/
@NameInMap("content")
public String content;
/**
* example:
* dingdf19b4ee0d73233735c2f4657eb6378f
*/
@NameInMap("corpId")
public String corpId;
/**
* example:
* 2023-11-16
*/
@NameInMap("effectTime")
public String effectTime;
@NameInMap("finished")
public Boolean finished;
/**
* example:
* 2023-11-19
*/
@NameInMap("gmtCreate")
public String gmtCreate;
/**
* example:
* 2023-11-16
*/
@NameInMap("optEndTime")
public String optEndTime;
/**
* example:
* 234234234
*/
@NameInMap("optEndUserId")
public String optEndUserId;
/**
* example:
* 张三
*/
@NameInMap("optEndUserName")
public String optEndUserName;
/**
* example:
* 2023-11-16
*/
@NameInMap("sendTime")
public String sendTime;
/**
* example:
* 2023-11-16
*/
@NameInMap("startTime")
public String startTime;
/**
* example:
* 2
*/
@NameInMap("status")
public Integer status;
/**
* example:
* 23234234
*/
@NameInMap("teacherId")
public String teacherId;
/**
* example:
* 张三
*/
@NameInMap("teacherName")
public String teacherName;
/**
* example:
* 每日锻炼
*/
@NameInMap("title")
public String title;
/**
* example:
* 1
*/
@NameInMap("type")
public Integer type;
public static CardBatchQueryCardsResponseBodyResultCards build(java.util.Map map) throws Exception {
CardBatchQueryCardsResponseBodyResultCards self = new CardBatchQueryCardsResponseBodyResultCards();
return TeaModel.build(map, self);
}
public CardBatchQueryCardsResponseBodyResultCards setCardBizCode(String cardBizCode) {
this.cardBizCode = cardBizCode;
return this;
}
public String getCardBizCode() {
return this.cardBizCode;
}
public CardBatchQueryCardsResponseBodyResultCards setCardId(Long cardId) {
this.cardId = cardId;
return this;
}
public Long getCardId() {
return this.cardId;
}
public CardBatchQueryCardsResponseBodyResultCards setCardStatus(Integer cardStatus) {
this.cardStatus = cardStatus;
return this;
}
public Integer getCardStatus() {
return this.cardStatus;
}
public CardBatchQueryCardsResponseBodyResultCards setContent(String content) {
this.content = content;
return this;
}
public String getContent() {
return this.content;
}
public CardBatchQueryCardsResponseBodyResultCards setCorpId(String corpId) {
this.corpId = corpId;
return this;
}
public String getCorpId() {
return this.corpId;
}
public CardBatchQueryCardsResponseBodyResultCards setEffectTime(String effectTime) {
this.effectTime = effectTime;
return this;
}
public String getEffectTime() {
return this.effectTime;
}
public CardBatchQueryCardsResponseBodyResultCards setFinished(Boolean finished) {
this.finished = finished;
return this;
}
public Boolean getFinished() {
return this.finished;
}
public CardBatchQueryCardsResponseBodyResultCards setGmtCreate(String gmtCreate) {
this.gmtCreate = gmtCreate;
return this;
}
public String getGmtCreate() {
return this.gmtCreate;
}
public CardBatchQueryCardsResponseBodyResultCards setOptEndTime(String optEndTime) {
this.optEndTime = optEndTime;
return this;
}
public String getOptEndTime() {
return this.optEndTime;
}
public CardBatchQueryCardsResponseBodyResultCards setOptEndUserId(String optEndUserId) {
this.optEndUserId = optEndUserId;
return this;
}
public String getOptEndUserId() {
return this.optEndUserId;
}
public CardBatchQueryCardsResponseBodyResultCards setOptEndUserName(String optEndUserName) {
this.optEndUserName = optEndUserName;
return this;
}
public String getOptEndUserName() {
return this.optEndUserName;
}
public CardBatchQueryCardsResponseBodyResultCards setSendTime(String sendTime) {
this.sendTime = sendTime;
return this;
}
public String getSendTime() {
return this.sendTime;
}
public CardBatchQueryCardsResponseBodyResultCards setStartTime(String startTime) {
this.startTime = startTime;
return this;
}
public String getStartTime() {
return this.startTime;
}
public CardBatchQueryCardsResponseBodyResultCards setStatus(Integer status) {
this.status = status;
return this;
}
public Integer getStatus() {
return this.status;
}
public CardBatchQueryCardsResponseBodyResultCards setTeacherId(String teacherId) {
this.teacherId = teacherId;
return this;
}
public String getTeacherId() {
return this.teacherId;
}
public CardBatchQueryCardsResponseBodyResultCards setTeacherName(String teacherName) {
this.teacherName = teacherName;
return this;
}
public String getTeacherName() {
return this.teacherName;
}
public CardBatchQueryCardsResponseBodyResultCards setTitle(String title) {
this.title = title;
return this;
}
public String getTitle() {
return this.title;
}
public CardBatchQueryCardsResponseBodyResultCards setType(Integer type) {
this.type = type;
return this;
}
public Integer getType() {
return this.type;
}
}
public static class CardBatchQueryCardsResponseBodyResult extends TeaModel {
@NameInMap("cards")
public java.util.List cards;
public static CardBatchQueryCardsResponseBodyResult build(java.util.Map map) throws Exception {
CardBatchQueryCardsResponseBodyResult self = new CardBatchQueryCardsResponseBodyResult();
return TeaModel.build(map, self);
}
public CardBatchQueryCardsResponseBodyResult setCards(java.util.List cards) {
this.cards = cards;
return this;
}
public java.util.List getCards() {
return this.cards;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy