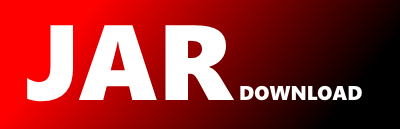
com.aliyun.dingtalkedu_1_0.models.UpdateCoursesOfClassRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dingtalk Show documentation
Show all versions of dingtalk Show documentation
Alibaba Cloud dingtalk SDK for Java
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.dingtalkedu_1_0.models;
import com.aliyun.tea.*;
public class UpdateCoursesOfClassRequest extends TeaModel {
/**
* This parameter is required.
*/
@NameInMap("courses")
public java.util.List courses;
/**
* This parameter is required.
*/
@NameInMap("sectionConfig")
public UpdateCoursesOfClassRequestSectionConfig sectionConfig;
/**
* This parameter is required.
*
* example:
* 234536346
*/
@NameInMap("opUserId")
public String opUserId;
public static UpdateCoursesOfClassRequest build(java.util.Map map) throws Exception {
UpdateCoursesOfClassRequest self = new UpdateCoursesOfClassRequest();
return TeaModel.build(map, self);
}
public UpdateCoursesOfClassRequest setCourses(java.util.List courses) {
this.courses = courses;
return this;
}
public java.util.List getCourses() {
return this.courses;
}
public UpdateCoursesOfClassRequest setSectionConfig(UpdateCoursesOfClassRequestSectionConfig sectionConfig) {
this.sectionConfig = sectionConfig;
return this;
}
public UpdateCoursesOfClassRequestSectionConfig getSectionConfig() {
return this.sectionConfig;
}
public UpdateCoursesOfClassRequest setOpUserId(String opUserId) {
this.opUserId = opUserId;
return this;
}
public String getOpUserId() {
return this.opUserId;
}
public static class UpdateCoursesOfClassRequestCoursesDateModel extends TeaModel {
/**
* This parameter is required.
*
* example:
* 9
*/
@NameInMap("dayOfMonth")
public Integer dayOfMonth;
/**
* This parameter is required.
*
* example:
* 11
*/
@NameInMap("month")
public Integer month;
/**
* This parameter is required.
*
* example:
* 2020
*/
@NameInMap("year")
public Integer year;
public static UpdateCoursesOfClassRequestCoursesDateModel build(java.util.Map map) throws Exception {
UpdateCoursesOfClassRequestCoursesDateModel self = new UpdateCoursesOfClassRequestCoursesDateModel();
return TeaModel.build(map, self);
}
public UpdateCoursesOfClassRequestCoursesDateModel setDayOfMonth(Integer dayOfMonth) {
this.dayOfMonth = dayOfMonth;
return this;
}
public Integer getDayOfMonth() {
return this.dayOfMonth;
}
public UpdateCoursesOfClassRequestCoursesDateModel setMonth(Integer month) {
this.month = month;
return this;
}
public Integer getMonth() {
return this.month;
}
public UpdateCoursesOfClassRequestCoursesDateModel setYear(Integer year) {
this.year = year;
return this;
}
public Integer getYear() {
return this.year;
}
}
public static class UpdateCoursesOfClassRequestCoursesSectionModel extends TeaModel {
/**
* This parameter is required.
*
* example:
* 1
*/
@NameInMap("sectionIndex")
public Integer sectionIndex;
/**
* This parameter is required.
*
* example:
* 第一节/午休
*/
@NameInMap("sectionName")
public String sectionName;
@NameInMap("sectionType")
public String sectionType;
public static UpdateCoursesOfClassRequestCoursesSectionModel build(java.util.Map map) throws Exception {
UpdateCoursesOfClassRequestCoursesSectionModel self = new UpdateCoursesOfClassRequestCoursesSectionModel();
return TeaModel.build(map, self);
}
public UpdateCoursesOfClassRequestCoursesSectionModel setSectionIndex(Integer sectionIndex) {
this.sectionIndex = sectionIndex;
return this;
}
public Integer getSectionIndex() {
return this.sectionIndex;
}
public UpdateCoursesOfClassRequestCoursesSectionModel setSectionName(String sectionName) {
this.sectionName = sectionName;
return this;
}
public String getSectionName() {
return this.sectionName;
}
public UpdateCoursesOfClassRequestCoursesSectionModel setSectionType(String sectionType) {
this.sectionType = sectionType;
return this;
}
public String getSectionType() {
return this.sectionType;
}
}
public static class UpdateCoursesOfClassRequestCourses extends TeaModel {
@NameInMap("courseCode")
public String courseCode;
/**
* This parameter is required.
*/
@NameInMap("courseGroupCode")
public String courseGroupCode;
/**
* This parameter is required.
*
* example:
* 语文
*/
@NameInMap("courseName")
public String courseName;
/**
* example:
* 李老师
*/
@NameInMap("creatorName")
public String creatorName;
/**
* This parameter is required.
*/
@NameInMap("dateModel")
public UpdateCoursesOfClassRequestCoursesDateModel dateModel;
@NameInMap("deleteTag")
public Boolean deleteTag;
/**
* example:
* 正心楼1-1
*/
@NameInMap("location")
public String location;
/**
* This parameter is required.
*/
@NameInMap("sectionModel")
public UpdateCoursesOfClassRequestCoursesSectionModel sectionModel;
@NameInMap("teacherStaffIds")
public java.util.List teacherStaffIds;
public static UpdateCoursesOfClassRequestCourses build(java.util.Map map) throws Exception {
UpdateCoursesOfClassRequestCourses self = new UpdateCoursesOfClassRequestCourses();
return TeaModel.build(map, self);
}
public UpdateCoursesOfClassRequestCourses setCourseCode(String courseCode) {
this.courseCode = courseCode;
return this;
}
public String getCourseCode() {
return this.courseCode;
}
public UpdateCoursesOfClassRequestCourses setCourseGroupCode(String courseGroupCode) {
this.courseGroupCode = courseGroupCode;
return this;
}
public String getCourseGroupCode() {
return this.courseGroupCode;
}
public UpdateCoursesOfClassRequestCourses setCourseName(String courseName) {
this.courseName = courseName;
return this;
}
public String getCourseName() {
return this.courseName;
}
public UpdateCoursesOfClassRequestCourses setCreatorName(String creatorName) {
this.creatorName = creatorName;
return this;
}
public String getCreatorName() {
return this.creatorName;
}
public UpdateCoursesOfClassRequestCourses setDateModel(UpdateCoursesOfClassRequestCoursesDateModel dateModel) {
this.dateModel = dateModel;
return this;
}
public UpdateCoursesOfClassRequestCoursesDateModel getDateModel() {
return this.dateModel;
}
public UpdateCoursesOfClassRequestCourses setDeleteTag(Boolean deleteTag) {
this.deleteTag = deleteTag;
return this;
}
public Boolean getDeleteTag() {
return this.deleteTag;
}
public UpdateCoursesOfClassRequestCourses setLocation(String location) {
this.location = location;
return this;
}
public String getLocation() {
return this.location;
}
public UpdateCoursesOfClassRequestCourses setSectionModel(UpdateCoursesOfClassRequestCoursesSectionModel sectionModel) {
this.sectionModel = sectionModel;
return this;
}
public UpdateCoursesOfClassRequestCoursesSectionModel getSectionModel() {
return this.sectionModel;
}
public UpdateCoursesOfClassRequestCourses setTeacherStaffIds(java.util.List teacherStaffIds) {
this.teacherStaffIds = teacherStaffIds;
return this;
}
public java.util.List getTeacherStaffIds() {
return this.teacherStaffIds;
}
}
public static class UpdateCoursesOfClassRequestSectionConfigSectionModelsEnd extends TeaModel {
/**
* This parameter is required.
*
* example:
* 10
*/
@NameInMap("hour")
public Integer hour;
/**
* This parameter is required.
*
* example:
* 45
*/
@NameInMap("min")
public Integer min;
public static UpdateCoursesOfClassRequestSectionConfigSectionModelsEnd build(java.util.Map map) throws Exception {
UpdateCoursesOfClassRequestSectionConfigSectionModelsEnd self = new UpdateCoursesOfClassRequestSectionConfigSectionModelsEnd();
return TeaModel.build(map, self);
}
public UpdateCoursesOfClassRequestSectionConfigSectionModelsEnd setHour(Integer hour) {
this.hour = hour;
return this;
}
public Integer getHour() {
return this.hour;
}
public UpdateCoursesOfClassRequestSectionConfigSectionModelsEnd setMin(Integer min) {
this.min = min;
return this;
}
public Integer getMin() {
return this.min;
}
}
public static class UpdateCoursesOfClassRequestSectionConfigSectionModelsStart extends TeaModel {
/**
* This parameter is required.
*
* example:
* 10
*/
@NameInMap("hour")
public Integer hour;
/**
* This parameter is required.
*
* example:
* 0
*/
@NameInMap("min")
public Integer min;
public static UpdateCoursesOfClassRequestSectionConfigSectionModelsStart build(java.util.Map map) throws Exception {
UpdateCoursesOfClassRequestSectionConfigSectionModelsStart self = new UpdateCoursesOfClassRequestSectionConfigSectionModelsStart();
return TeaModel.build(map, self);
}
public UpdateCoursesOfClassRequestSectionConfigSectionModelsStart setHour(Integer hour) {
this.hour = hour;
return this;
}
public Integer getHour() {
return this.hour;
}
public UpdateCoursesOfClassRequestSectionConfigSectionModelsStart setMin(Integer min) {
this.min = min;
return this;
}
public Integer getMin() {
return this.min;
}
}
public static class UpdateCoursesOfClassRequestSectionConfigSectionModels extends TeaModel {
/**
* This parameter is required.
*/
@NameInMap("end")
public UpdateCoursesOfClassRequestSectionConfigSectionModelsEnd end;
/**
* This parameter is required.
*
* example:
* 1
*/
@NameInMap("sectionIndex")
public Integer sectionIndex;
/**
* example:
* COURSE:上课节次 REST:休息节次
*/
@NameInMap("sectionType")
public String sectionType;
/**
* This parameter is required.
*/
@NameInMap("start")
public UpdateCoursesOfClassRequestSectionConfigSectionModelsStart start;
public static UpdateCoursesOfClassRequestSectionConfigSectionModels build(java.util.Map map) throws Exception {
UpdateCoursesOfClassRequestSectionConfigSectionModels self = new UpdateCoursesOfClassRequestSectionConfigSectionModels();
return TeaModel.build(map, self);
}
public UpdateCoursesOfClassRequestSectionConfigSectionModels setEnd(UpdateCoursesOfClassRequestSectionConfigSectionModelsEnd end) {
this.end = end;
return this;
}
public UpdateCoursesOfClassRequestSectionConfigSectionModelsEnd getEnd() {
return this.end;
}
public UpdateCoursesOfClassRequestSectionConfigSectionModels setSectionIndex(Integer sectionIndex) {
this.sectionIndex = sectionIndex;
return this;
}
public Integer getSectionIndex() {
return this.sectionIndex;
}
public UpdateCoursesOfClassRequestSectionConfigSectionModels setSectionType(String sectionType) {
this.sectionType = sectionType;
return this;
}
public String getSectionType() {
return this.sectionType;
}
public UpdateCoursesOfClassRequestSectionConfigSectionModels setStart(UpdateCoursesOfClassRequestSectionConfigSectionModelsStart start) {
this.start = start;
return this;
}
public UpdateCoursesOfClassRequestSectionConfigSectionModelsStart getStart() {
return this.start;
}
}
public static class UpdateCoursesOfClassRequestSectionConfig extends TeaModel {
/**
* This parameter is required.
*/
@NameInMap("sectionModels")
public java.util.List sectionModels;
public static UpdateCoursesOfClassRequestSectionConfig build(java.util.Map map) throws Exception {
UpdateCoursesOfClassRequestSectionConfig self = new UpdateCoursesOfClassRequestSectionConfig();
return TeaModel.build(map, self);
}
public UpdateCoursesOfClassRequestSectionConfig setSectionModels(java.util.List sectionModels) {
this.sectionModels = sectionModels;
return this;
}
public java.util.List getSectionModels() {
return this.sectionModels;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy