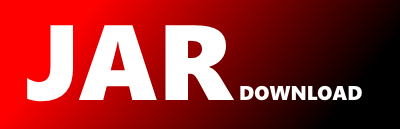
com.aliyun.dingtalkexclusive_1_0.models.SaveAndSubmitAuthInfoRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dingtalk Show documentation
Show all versions of dingtalk Show documentation
Alibaba Cloud dingtalk SDK for Java
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.dingtalkexclusive_1_0.models;
import com.aliyun.tea.*;
public class SaveAndSubmitAuthInfoRequest extends TeaModel {
/**
* example:
* XXX组织申请高级认证
*/
@NameInMap("applyRemark")
public String applyRemark;
/**
* example:
* @lQLxxxxxxxxVvjg8zImwm6t1BYIUNv0Cas0x7UA-AA
*/
@NameInMap("authorizeMediaId")
public String authorizeMediaId;
/**
* This parameter is required.
*
* example:
* 计算机
*/
@NameInMap("industry")
public String industry;
/**
* This parameter is required.
*
* example:
* 钉三多
*/
@NameInMap("legalPerson")
public String legalPerson;
/**
* This parameter is required.
*
* example:
* 3301XX1997XXXXXXXXX
*/
@NameInMap("legalPersonIdCard")
public String legalPersonIdCard;
/**
* example:
* @lQLxxxxxxxxVvjg8zImwm6t1BYIUNv0Cas0x7UA-AA
*/
@NameInMap("licenseMediaId")
public String licenseMediaId;
/**
* This parameter is required.
*
* example:
* 330100
*/
@NameInMap("locCity")
public Long locCity;
/**
* This parameter is required.
*
* example:
* 杭州
*/
@NameInMap("locCityName")
public String locCityName;
/**
* This parameter is required.
*
* example:
* 330000
*/
@NameInMap("locProvince")
public Long locProvince;
/**
* This parameter is required.
*
* example:
* 浙江
*/
@NameInMap("locProvinceName")
public String locProvinceName;
/**
* This parameter is required.
*
* example:
* 15869110714
*/
@NameInMap("mobileNum")
public String mobileNum;
/**
* This parameter is required.
*
* example:
* 测试组织
*/
@NameInMap("orgName")
public String orgName;
/**
* example:
* 11111111-1
*/
@NameInMap("organizationCode")
public String organizationCode;
/**
* example:
* @lQLxxxxxxxxVvjg8zImwm6t1BYIUNv0Cas0x7UA-AA
*/
@NameInMap("organizationCodeMediaId")
public String organizationCodeMediaId;
/**
* This parameter is required.
*
* example:
* 余杭区文一西路XX号
*/
@NameInMap("registLocation")
public String registLocation;
/**
* example:
* 1111111111111111
*/
@NameInMap("registNum")
public String registNum;
/**
* This parameter is required.
*
* example:
* dingxxxxxxxxxxxx
*/
@NameInMap("targetCorpId")
public String targetCorpId;
/**
* example:
* 9111111XX2957XX4X
*/
@NameInMap("unifiedSocialCredit")
public String unifiedSocialCredit;
public static SaveAndSubmitAuthInfoRequest build(java.util.Map map) throws Exception {
SaveAndSubmitAuthInfoRequest self = new SaveAndSubmitAuthInfoRequest();
return TeaModel.build(map, self);
}
public SaveAndSubmitAuthInfoRequest setApplyRemark(String applyRemark) {
this.applyRemark = applyRemark;
return this;
}
public String getApplyRemark() {
return this.applyRemark;
}
public SaveAndSubmitAuthInfoRequest setAuthorizeMediaId(String authorizeMediaId) {
this.authorizeMediaId = authorizeMediaId;
return this;
}
public String getAuthorizeMediaId() {
return this.authorizeMediaId;
}
public SaveAndSubmitAuthInfoRequest setIndustry(String industry) {
this.industry = industry;
return this;
}
public String getIndustry() {
return this.industry;
}
public SaveAndSubmitAuthInfoRequest setLegalPerson(String legalPerson) {
this.legalPerson = legalPerson;
return this;
}
public String getLegalPerson() {
return this.legalPerson;
}
public SaveAndSubmitAuthInfoRequest setLegalPersonIdCard(String legalPersonIdCard) {
this.legalPersonIdCard = legalPersonIdCard;
return this;
}
public String getLegalPersonIdCard() {
return this.legalPersonIdCard;
}
public SaveAndSubmitAuthInfoRequest setLicenseMediaId(String licenseMediaId) {
this.licenseMediaId = licenseMediaId;
return this;
}
public String getLicenseMediaId() {
return this.licenseMediaId;
}
public SaveAndSubmitAuthInfoRequest setLocCity(Long locCity) {
this.locCity = locCity;
return this;
}
public Long getLocCity() {
return this.locCity;
}
public SaveAndSubmitAuthInfoRequest setLocCityName(String locCityName) {
this.locCityName = locCityName;
return this;
}
public String getLocCityName() {
return this.locCityName;
}
public SaveAndSubmitAuthInfoRequest setLocProvince(Long locProvince) {
this.locProvince = locProvince;
return this;
}
public Long getLocProvince() {
return this.locProvince;
}
public SaveAndSubmitAuthInfoRequest setLocProvinceName(String locProvinceName) {
this.locProvinceName = locProvinceName;
return this;
}
public String getLocProvinceName() {
return this.locProvinceName;
}
public SaveAndSubmitAuthInfoRequest setMobileNum(String mobileNum) {
this.mobileNum = mobileNum;
return this;
}
public String getMobileNum() {
return this.mobileNum;
}
public SaveAndSubmitAuthInfoRequest setOrgName(String orgName) {
this.orgName = orgName;
return this;
}
public String getOrgName() {
return this.orgName;
}
public SaveAndSubmitAuthInfoRequest setOrganizationCode(String organizationCode) {
this.organizationCode = organizationCode;
return this;
}
public String getOrganizationCode() {
return this.organizationCode;
}
public SaveAndSubmitAuthInfoRequest setOrganizationCodeMediaId(String organizationCodeMediaId) {
this.organizationCodeMediaId = organizationCodeMediaId;
return this;
}
public String getOrganizationCodeMediaId() {
return this.organizationCodeMediaId;
}
public SaveAndSubmitAuthInfoRequest setRegistLocation(String registLocation) {
this.registLocation = registLocation;
return this;
}
public String getRegistLocation() {
return this.registLocation;
}
public SaveAndSubmitAuthInfoRequest setRegistNum(String registNum) {
this.registNum = registNum;
return this;
}
public String getRegistNum() {
return this.registNum;
}
public SaveAndSubmitAuthInfoRequest setTargetCorpId(String targetCorpId) {
this.targetCorpId = targetCorpId;
return this;
}
public String getTargetCorpId() {
return this.targetCorpId;
}
public SaveAndSubmitAuthInfoRequest setUnifiedSocialCredit(String unifiedSocialCredit) {
this.unifiedSocialCredit = unifiedSocialCredit;
return this;
}
public String getUnifiedSocialCredit() {
return this.unifiedSocialCredit;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy