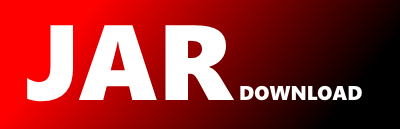
com.aliyun.dingtalkbizfinance_1_0.models.QueryCustomerInfoResponseBody Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dingtalk Show documentation
Show all versions of dingtalk Show documentation
Alibaba Cloud dingtalk SDK for Java
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.dingtalkbizfinance_1_0.models;
import com.aliyun.tea.*;
public class QueryCustomerInfoResponseBody extends TeaModel {
/**
* example:
* true
*/
@NameInMap("hasMore")
public Boolean hasMore;
@NameInMap("list")
public java.util.List list;
/**
* example:
* 500
*/
@NameInMap("totalCount")
public Long totalCount;
public static QueryCustomerInfoResponseBody build(java.util.Map map) throws Exception {
QueryCustomerInfoResponseBody self = new QueryCustomerInfoResponseBody();
return TeaModel.build(map, self);
}
public QueryCustomerInfoResponseBody setHasMore(Boolean hasMore) {
this.hasMore = hasMore;
return this;
}
public Boolean getHasMore() {
return this.hasMore;
}
public QueryCustomerInfoResponseBody setList(java.util.List list) {
this.list = list;
return this;
}
public java.util.List getList() {
return this.list;
}
public QueryCustomerInfoResponseBody setTotalCount(Long totalCount) {
this.totalCount = totalCount;
return this;
}
public Long getTotalCount() {
return this.totalCount;
}
public static class QueryCustomerInfoResponseBodyList extends TeaModel {
/**
* example:
* CUS_xxxxxxxx
*/
@NameInMap("code")
public String code;
@NameInMap("contactAddress")
public String contactAddress;
@NameInMap("contactCompanyTelephone")
public String contactCompanyTelephone;
@NameInMap("contactEmail")
public String contactEmail;
@NameInMap("contactName")
public String contactName;
@NameInMap("contactTelephone")
public String contactTelephone;
/**
* example:
* abc
*/
@NameInMap("description")
public String description;
/**
* example:
*
*/
@NameInMap("drawerEmail")
public String drawerEmail;
/**
* example:
* 12345678901
*/
@NameInMap("drawerTelephone")
public String drawerTelephone;
/**
* example:
* 张三
*/
@NameInMap("name")
public String name;
/**
* example:
* abc
*/
@NameInMap("purchaserAccount")
public String purchaserAccount;
/**
* example:
* 杭州市
*/
@NameInMap("purchaserAddress")
public String purchaserAddress;
/**
* example:
* abc
*/
@NameInMap("purchaserName")
public String purchaserName;
/**
* example:
* 123
*/
@NameInMap("purchaserTaxNo")
public String purchaserTaxNo;
/**
* example:
* 13333333333
*/
@NameInMap("purchaserTel")
public String purchaserTel;
/**
* example:
* 建行
*/
@NameInMap("purchaserrBankName")
public String purchaserrBankName;
/**
* example:
* valid
*/
@NameInMap("status")
public String status;
/**
* example:
* 199200
*/
@NameInMap("userDefineCode")
public String userDefineCode;
public static QueryCustomerInfoResponseBodyList build(java.util.Map map) throws Exception {
QueryCustomerInfoResponseBodyList self = new QueryCustomerInfoResponseBodyList();
return TeaModel.build(map, self);
}
public QueryCustomerInfoResponseBodyList setCode(String code) {
this.code = code;
return this;
}
public String getCode() {
return this.code;
}
public QueryCustomerInfoResponseBodyList setContactAddress(String contactAddress) {
this.contactAddress = contactAddress;
return this;
}
public String getContactAddress() {
return this.contactAddress;
}
public QueryCustomerInfoResponseBodyList setContactCompanyTelephone(String contactCompanyTelephone) {
this.contactCompanyTelephone = contactCompanyTelephone;
return this;
}
public String getContactCompanyTelephone() {
return this.contactCompanyTelephone;
}
public QueryCustomerInfoResponseBodyList setContactEmail(String contactEmail) {
this.contactEmail = contactEmail;
return this;
}
public String getContactEmail() {
return this.contactEmail;
}
public QueryCustomerInfoResponseBodyList setContactName(String contactName) {
this.contactName = contactName;
return this;
}
public String getContactName() {
return this.contactName;
}
public QueryCustomerInfoResponseBodyList setContactTelephone(String contactTelephone) {
this.contactTelephone = contactTelephone;
return this;
}
public String getContactTelephone() {
return this.contactTelephone;
}
public QueryCustomerInfoResponseBodyList setDescription(String description) {
this.description = description;
return this;
}
public String getDescription() {
return this.description;
}
public QueryCustomerInfoResponseBodyList setDrawerEmail(String drawerEmail) {
this.drawerEmail = drawerEmail;
return this;
}
public String getDrawerEmail() {
return this.drawerEmail;
}
public QueryCustomerInfoResponseBodyList setDrawerTelephone(String drawerTelephone) {
this.drawerTelephone = drawerTelephone;
return this;
}
public String getDrawerTelephone() {
return this.drawerTelephone;
}
public QueryCustomerInfoResponseBodyList setName(String name) {
this.name = name;
return this;
}
public String getName() {
return this.name;
}
public QueryCustomerInfoResponseBodyList setPurchaserAccount(String purchaserAccount) {
this.purchaserAccount = purchaserAccount;
return this;
}
public String getPurchaserAccount() {
return this.purchaserAccount;
}
public QueryCustomerInfoResponseBodyList setPurchaserAddress(String purchaserAddress) {
this.purchaserAddress = purchaserAddress;
return this;
}
public String getPurchaserAddress() {
return this.purchaserAddress;
}
public QueryCustomerInfoResponseBodyList setPurchaserName(String purchaserName) {
this.purchaserName = purchaserName;
return this;
}
public String getPurchaserName() {
return this.purchaserName;
}
public QueryCustomerInfoResponseBodyList setPurchaserTaxNo(String purchaserTaxNo) {
this.purchaserTaxNo = purchaserTaxNo;
return this;
}
public String getPurchaserTaxNo() {
return this.purchaserTaxNo;
}
public QueryCustomerInfoResponseBodyList setPurchaserTel(String purchaserTel) {
this.purchaserTel = purchaserTel;
return this;
}
public String getPurchaserTel() {
return this.purchaserTel;
}
public QueryCustomerInfoResponseBodyList setPurchaserrBankName(String purchaserrBankName) {
this.purchaserrBankName = purchaserrBankName;
return this;
}
public String getPurchaserrBankName() {
return this.purchaserrBankName;
}
public QueryCustomerInfoResponseBodyList setStatus(String status) {
this.status = status;
return this;
}
public String getStatus() {
return this.status;
}
public QueryCustomerInfoResponseBodyList setUserDefineCode(String userDefineCode) {
this.userDefineCode = userDefineCode;
return this;
}
public String getUserDefineCode() {
return this.userDefineCode;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy