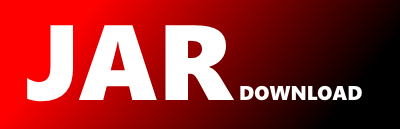
com.aliyun.ens20171110.models.DescribeEpnInstancesResponseBody Maven / Gradle / Ivy
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.ens20171110.models;
import com.aliyun.tea.*;
public class DescribeEpnInstancesResponseBody extends TeaModel {
/**
* Details of EPN instances.
*/
@NameInMap("EPNInstances")
public DescribeEpnInstancesResponseBodyEPNInstances EPNInstances;
/**
* The page number.
*
* example:
* 1
*/
@NameInMap("PageNumber")
public Integer pageNumber;
/**
* The number of entries per page.
*
* example:
* 40
*/
@NameInMap("PageSize")
public Integer pageSize;
/**
* The request ID.
*
* example:
* A1707FC0-430C-423A-B624-284046B20399
*/
@NameInMap("RequestId")
public String requestId;
/**
* The total number of pages returned.
*
* example:
* 1
*/
@NameInMap("TotalCount")
public Integer totalCount;
public static DescribeEpnInstancesResponseBody build(java.util.Map map) throws Exception {
DescribeEpnInstancesResponseBody self = new DescribeEpnInstancesResponseBody();
return TeaModel.build(map, self);
}
public DescribeEpnInstancesResponseBody setEPNInstances(DescribeEpnInstancesResponseBodyEPNInstances EPNInstances) {
this.EPNInstances = EPNInstances;
return this;
}
public DescribeEpnInstancesResponseBodyEPNInstances getEPNInstances() {
return this.EPNInstances;
}
public DescribeEpnInstancesResponseBody setPageNumber(Integer pageNumber) {
this.pageNumber = pageNumber;
return this;
}
public Integer getPageNumber() {
return this.pageNumber;
}
public DescribeEpnInstancesResponseBody setPageSize(Integer pageSize) {
this.pageSize = pageSize;
return this;
}
public Integer getPageSize() {
return this.pageSize;
}
public DescribeEpnInstancesResponseBody setRequestId(String requestId) {
this.requestId = requestId;
return this;
}
public String getRequestId() {
return this.requestId;
}
public DescribeEpnInstancesResponseBody setTotalCount(Integer totalCount) {
this.totalCount = totalCount;
return this;
}
public Integer getTotalCount() {
return this.totalCount;
}
public static class DescribeEpnInstancesResponseBodyEPNInstancesEPNInstance extends TeaModel {
/**
* The time when the instance was created. The time is displayed in UTC.
*
* example:
* 2019-11-01T06:08:46Z
*/
@NameInMap("CreationTime")
public String creationTime;
/**
* The ID of the EPN instance.
*
* example:
* epn****
*/
@NameInMap("EPNInstanceId")
public String EPNInstanceId;
/**
* The name of the EPN instance.
*
* example:
* ens_test_epn
*/
@NameInMap("EPNInstanceName")
public String EPNInstanceName;
/**
* Set the value to EdgeToEdge.
*
* example:
* EdgeToEdge
*/
@NameInMap("EPNInstanceType")
public String EPNInstanceType;
/**
* The end of the time range during which the data was queried. The time is displayed in UTC.
*
* example:
* 2019-11-01T06:08:46Z
*/
@NameInMap("EndTime")
public String endTime;
/**
* The inbound bandwidth. Unit: Mbit/s.
*
* example:
* 40
*/
@NameInMap("InternetMaxBandwidthOut")
public Integer internetMaxBandwidthOut;
/**
* The time when the instance was last modified. The time is displayed in UTC.
*
* example:
* 2019-11-01T06:08:46Z
*/
@NameInMap("ModifyTime")
public String modifyTime;
/**
* The networking mode. Valid values:
*
* - SpeedUp: intelligent acceleration network (Internet)
* - Connection: internal network
* - SpeedUpAndConnection: intelligent acceleration network and internal network
*
*
* example:
* SpeedUp
*/
@NameInMap("NetworkingModel")
public String networkingModel;
/**
* The beginning of the time range during which the data was queried. The time is displayed in UTC.
*
* example:
* 2019-11-01T06:08:46Z
*/
@NameInMap("StartTime")
public String startTime;
/**
* The status of the instance. Valid values:
*
* - Running
* - Excuting
* - Stopped
*
*
* example:
* Running
*/
@NameInMap("Status")
public String status;
public static DescribeEpnInstancesResponseBodyEPNInstancesEPNInstance build(java.util.Map map) throws Exception {
DescribeEpnInstancesResponseBodyEPNInstancesEPNInstance self = new DescribeEpnInstancesResponseBodyEPNInstancesEPNInstance();
return TeaModel.build(map, self);
}
public DescribeEpnInstancesResponseBodyEPNInstancesEPNInstance setCreationTime(String creationTime) {
this.creationTime = creationTime;
return this;
}
public String getCreationTime() {
return this.creationTime;
}
public DescribeEpnInstancesResponseBodyEPNInstancesEPNInstance setEPNInstanceId(String EPNInstanceId) {
this.EPNInstanceId = EPNInstanceId;
return this;
}
public String getEPNInstanceId() {
return this.EPNInstanceId;
}
public DescribeEpnInstancesResponseBodyEPNInstancesEPNInstance setEPNInstanceName(String EPNInstanceName) {
this.EPNInstanceName = EPNInstanceName;
return this;
}
public String getEPNInstanceName() {
return this.EPNInstanceName;
}
public DescribeEpnInstancesResponseBodyEPNInstancesEPNInstance setEPNInstanceType(String EPNInstanceType) {
this.EPNInstanceType = EPNInstanceType;
return this;
}
public String getEPNInstanceType() {
return this.EPNInstanceType;
}
public DescribeEpnInstancesResponseBodyEPNInstancesEPNInstance setEndTime(String endTime) {
this.endTime = endTime;
return this;
}
public String getEndTime() {
return this.endTime;
}
public DescribeEpnInstancesResponseBodyEPNInstancesEPNInstance setInternetMaxBandwidthOut(Integer internetMaxBandwidthOut) {
this.internetMaxBandwidthOut = internetMaxBandwidthOut;
return this;
}
public Integer getInternetMaxBandwidthOut() {
return this.internetMaxBandwidthOut;
}
public DescribeEpnInstancesResponseBodyEPNInstancesEPNInstance setModifyTime(String modifyTime) {
this.modifyTime = modifyTime;
return this;
}
public String getModifyTime() {
return this.modifyTime;
}
public DescribeEpnInstancesResponseBodyEPNInstancesEPNInstance setNetworkingModel(String networkingModel) {
this.networkingModel = networkingModel;
return this;
}
public String getNetworkingModel() {
return this.networkingModel;
}
public DescribeEpnInstancesResponseBodyEPNInstancesEPNInstance setStartTime(String startTime) {
this.startTime = startTime;
return this;
}
public String getStartTime() {
return this.startTime;
}
public DescribeEpnInstancesResponseBodyEPNInstancesEPNInstance setStatus(String status) {
this.status = status;
return this;
}
public String getStatus() {
return this.status;
}
}
public static class DescribeEpnInstancesResponseBodyEPNInstances extends TeaModel {
@NameInMap("EPNInstance")
public java.util.List EPNInstance;
public static DescribeEpnInstancesResponseBodyEPNInstances build(java.util.Map map) throws Exception {
DescribeEpnInstancesResponseBodyEPNInstances self = new DescribeEpnInstancesResponseBodyEPNInstances();
return TeaModel.build(map, self);
}
public DescribeEpnInstancesResponseBodyEPNInstances setEPNInstance(java.util.List EPNInstance) {
this.EPNInstance = EPNInstance;
return this;
}
public java.util.List getEPNInstance() {
return this.EPNInstance;
}
}
}