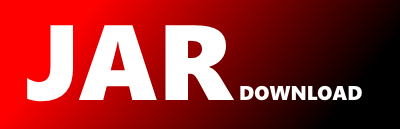
com.aliyun.ens20171110.models.DescribeStorageGatewayResponseBody Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ens20171110 Show documentation
Show all versions of ens20171110 Show documentation
Alibaba Cloud edge node service (20171110) SDK for Java
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.ens20171110.models;
import com.aliyun.tea.*;
public class DescribeStorageGatewayResponseBody extends TeaModel {
/**
* The page number. Default value: 1.
*
* example:
* 1
*/
@NameInMap("PageNumber")
public Integer pageNumber;
/**
* The number of entries per page.
* Default value: 10.
*
* example:
* 10
*/
@NameInMap("PageSize")
public Integer pageSize;
/**
* Id of the request
*
* example:
* 6666C5A5-75ED-422E-A022-7121FA18C968
*/
@NameInMap("RequestId")
public String requestId;
/**
* The list information.
*/
@NameInMap("StorageGateways")
public java.util.List storageGateways;
/**
* The total number of entries returned.
*
* example:
* 16
*/
@NameInMap("TotalCount")
public Integer totalCount;
public static DescribeStorageGatewayResponseBody build(java.util.Map map) throws Exception {
DescribeStorageGatewayResponseBody self = new DescribeStorageGatewayResponseBody();
return TeaModel.build(map, self);
}
public DescribeStorageGatewayResponseBody setPageNumber(Integer pageNumber) {
this.pageNumber = pageNumber;
return this;
}
public Integer getPageNumber() {
return this.pageNumber;
}
public DescribeStorageGatewayResponseBody setPageSize(Integer pageSize) {
this.pageSize = pageSize;
return this;
}
public Integer getPageSize() {
return this.pageSize;
}
public DescribeStorageGatewayResponseBody setRequestId(String requestId) {
this.requestId = requestId;
return this;
}
public String getRequestId() {
return this.requestId;
}
public DescribeStorageGatewayResponseBody setStorageGateways(java.util.List storageGateways) {
this.storageGateways = storageGateways;
return this;
}
public java.util.List getStorageGateways() {
return this.storageGateways;
}
public DescribeStorageGatewayResponseBody setTotalCount(Integer totalCount) {
this.totalCount = totalCount;
return this;
}
public Integer getTotalCount() {
return this.totalCount;
}
public static class DescribeStorageGatewayResponseBodyStorageGateways extends TeaModel {
/**
* The internal CIDR block.
*
* example:
* 192.168.2.0/24
*/
@NameInMap("CidrBlock")
public String cidrBlock;
/**
* The time when the storage gateway was created. The time is displayed in UTC.
*
* example:
* 2024-05-14T03:07:47Z
*/
@NameInMap("CreationTime")
public String creationTime;
/**
* The description of the storage gateway.
*
* example:
* testDescription
*/
@NameInMap("Description")
public String description;
/**
* The ID of the node.
*
* example:
* cn-beijing-cmcc
*/
@NameInMap("EnsRegionId")
public String ensRegionId;
/**
* The IP address of the service.
*
* example:
* ...
*/
@NameInMap("ServiceIp")
public String serviceIp;
/**
* The status of the storage gateway. Valid values:
*
* - creating
* - available
* - deleting
* - deleted
*
*
* example:
* available
*/
@NameInMap("Status")
public String status;
/**
* The ID of the storage gateway.
*
* example:
* sgw-***
*/
@NameInMap("StorageGatewayId")
public String storageGatewayId;
/**
* The name of the storage gateway.
*
* example:
* testGateway
*/
@NameInMap("StorageGatewayName")
public String storageGatewayName;
/**
* The type of the storage gateway. Default value: 1, which indicates iSCSI.
*
* example:
* 1
*/
@NameInMap("StorageGatewayType")
public Integer storageGatewayType;
/**
* The ID of the VPC.
*
* example:
* n-***
*/
@NameInMap("VpcId")
public String vpcId;
public static DescribeStorageGatewayResponseBodyStorageGateways build(java.util.Map map) throws Exception {
DescribeStorageGatewayResponseBodyStorageGateways self = new DescribeStorageGatewayResponseBodyStorageGateways();
return TeaModel.build(map, self);
}
public DescribeStorageGatewayResponseBodyStorageGateways setCidrBlock(String cidrBlock) {
this.cidrBlock = cidrBlock;
return this;
}
public String getCidrBlock() {
return this.cidrBlock;
}
public DescribeStorageGatewayResponseBodyStorageGateways setCreationTime(String creationTime) {
this.creationTime = creationTime;
return this;
}
public String getCreationTime() {
return this.creationTime;
}
public DescribeStorageGatewayResponseBodyStorageGateways setDescription(String description) {
this.description = description;
return this;
}
public String getDescription() {
return this.description;
}
public DescribeStorageGatewayResponseBodyStorageGateways setEnsRegionId(String ensRegionId) {
this.ensRegionId = ensRegionId;
return this;
}
public String getEnsRegionId() {
return this.ensRegionId;
}
public DescribeStorageGatewayResponseBodyStorageGateways setServiceIp(String serviceIp) {
this.serviceIp = serviceIp;
return this;
}
public String getServiceIp() {
return this.serviceIp;
}
public DescribeStorageGatewayResponseBodyStorageGateways setStatus(String status) {
this.status = status;
return this;
}
public String getStatus() {
return this.status;
}
public DescribeStorageGatewayResponseBodyStorageGateways setStorageGatewayId(String storageGatewayId) {
this.storageGatewayId = storageGatewayId;
return this;
}
public String getStorageGatewayId() {
return this.storageGatewayId;
}
public DescribeStorageGatewayResponseBodyStorageGateways setStorageGatewayName(String storageGatewayName) {
this.storageGatewayName = storageGatewayName;
return this;
}
public String getStorageGatewayName() {
return this.storageGatewayName;
}
public DescribeStorageGatewayResponseBodyStorageGateways setStorageGatewayType(Integer storageGatewayType) {
this.storageGatewayType = storageGatewayType;
return this;
}
public Integer getStorageGatewayType() {
return this.storageGatewayType;
}
public DescribeStorageGatewayResponseBodyStorageGateways setVpcId(String vpcId) {
this.vpcId = vpcId;
return this;
}
public String getVpcId() {
return this.vpcId;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy