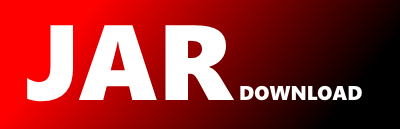
com.aliyun.ens20171110.models.DescribeSnapshotsResponseBody Maven / Gradle / Ivy
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.ens20171110.models;
import com.aliyun.tea.*;
public class DescribeSnapshotsResponseBody extends TeaModel {
/**
* The page number of the returned page.
*
* example:
* 1
*/
@NameInMap("PageNumber")
public Integer pageNumber;
/**
* The number of entries per page.
*
* example:
* 10
*/
@NameInMap("PageSize")
public Integer pageSize;
/**
* The request ID.
*
* example:
* 9635ED2E-3103-1606-84D4-9F8E816B19F9
*/
@NameInMap("RequestId")
public String requestId;
/**
* The information about the snapshots.
*/
@NameInMap("Snapshots")
public java.util.List snapshots;
/**
* The total number of snapshots.
*
* example:
* 1
*/
@NameInMap("TotalCount")
public Integer totalCount;
public static DescribeSnapshotsResponseBody build(java.util.Map map) throws Exception {
DescribeSnapshotsResponseBody self = new DescribeSnapshotsResponseBody();
return TeaModel.build(map, self);
}
public DescribeSnapshotsResponseBody setPageNumber(Integer pageNumber) {
this.pageNumber = pageNumber;
return this;
}
public Integer getPageNumber() {
return this.pageNumber;
}
public DescribeSnapshotsResponseBody setPageSize(Integer pageSize) {
this.pageSize = pageSize;
return this;
}
public Integer getPageSize() {
return this.pageSize;
}
public DescribeSnapshotsResponseBody setRequestId(String requestId) {
this.requestId = requestId;
return this;
}
public String getRequestId() {
return this.requestId;
}
public DescribeSnapshotsResponseBody setSnapshots(java.util.List snapshots) {
this.snapshots = snapshots;
return this;
}
public java.util.List getSnapshots() {
return this.snapshots;
}
public DescribeSnapshotsResponseBody setTotalCount(Integer totalCount) {
this.totalCount = totalCount;
return this;
}
public Integer getTotalCount() {
return this.totalCount;
}
public static class DescribeSnapshotsResponseBodySnapshots extends TeaModel {
/**
* The creation time. The time follows the ISO 8601 standard in the yyyy-MM-ddTHH:mm:ssZ format. The time is displayed in UTC.
*
* example:
* 2020-08-20T14:52:28Z
*/
@NameInMap("CreationTime")
public String creationTime;
/**
* The description of the snapshot.
*
* example:
* testDescription
*/
@NameInMap("Description")
public String description;
/**
* The ID of the edge node.
*
* example:
* cn-beijing-15
*/
@NameInMap("EnsRegionId")
public String ensRegionId;
/**
* The capacity of the disk. Unit: MiB.
*
* example:
* 40
*/
@NameInMap("Size")
public String size;
/**
* The ID of the snapshot.
*
* example:
* s-bp67acfmxazb4p****
*/
@NameInMap("SnapshotId")
public String snapshotId;
/**
* The name of the snapshot. This parameter is returned only if a snapshot name was specified when the snapshot was created.
*
* example:
* testSnapshotName
*/
@NameInMap("SnapshotName")
public String snapshotName;
/**
* The type of the disk. Valid value:
*
* - cloud_efficiency: ultra disk
* - cloud_ssd: all-flash disk
* - local_hdd: local HDD
* - local_ssd: local SSD
*
*
* example:
* cloud_efficiency
*/
@NameInMap("SourceDiskCategory")
public String sourceDiskCategory;
/**
* The ID of the source disk. This parameter is retained even after the source disk for which the snapshot was created is released.
*
* example:
* d-bp67acfmxazb4ph****
*/
@NameInMap("SourceDiskId")
public String sourceDiskId;
/**
* The type of the disk. Valid value:
*
* - 1: system disk
* - 2: data disk
*
*
* example:
* 1
*/
@NameInMap("SourceDiskType")
public String sourceDiskType;
/**
* The ID of the source edge node.
*
* example:
* cn-hangzhou-27
*/
@NameInMap("SourceEnsRegionId")
public String sourceEnsRegionId;
/**
* The ID of the source snapshot.
*
* example:
* s-bpdfer893jfkdqe****
*/
@NameInMap("SourceSnapshotId")
public String sourceSnapshotId;
/**
* The status of the snapshot. Valid value:
*
* - creating: The snapshot is being created.
* - Available: The snapshot is available.
* - deleting: The snapshot is being deleted.
* - error: An error occurred on the snapshot.
*
*
* example:
* available
*/
@NameInMap("Status")
public String status;
public static DescribeSnapshotsResponseBodySnapshots build(java.util.Map map) throws Exception {
DescribeSnapshotsResponseBodySnapshots self = new DescribeSnapshotsResponseBodySnapshots();
return TeaModel.build(map, self);
}
public DescribeSnapshotsResponseBodySnapshots setCreationTime(String creationTime) {
this.creationTime = creationTime;
return this;
}
public String getCreationTime() {
return this.creationTime;
}
public DescribeSnapshotsResponseBodySnapshots setDescription(String description) {
this.description = description;
return this;
}
public String getDescription() {
return this.description;
}
public DescribeSnapshotsResponseBodySnapshots setEnsRegionId(String ensRegionId) {
this.ensRegionId = ensRegionId;
return this;
}
public String getEnsRegionId() {
return this.ensRegionId;
}
public DescribeSnapshotsResponseBodySnapshots setSize(String size) {
this.size = size;
return this;
}
public String getSize() {
return this.size;
}
public DescribeSnapshotsResponseBodySnapshots setSnapshotId(String snapshotId) {
this.snapshotId = snapshotId;
return this;
}
public String getSnapshotId() {
return this.snapshotId;
}
public DescribeSnapshotsResponseBodySnapshots setSnapshotName(String snapshotName) {
this.snapshotName = snapshotName;
return this;
}
public String getSnapshotName() {
return this.snapshotName;
}
public DescribeSnapshotsResponseBodySnapshots setSourceDiskCategory(String sourceDiskCategory) {
this.sourceDiskCategory = sourceDiskCategory;
return this;
}
public String getSourceDiskCategory() {
return this.sourceDiskCategory;
}
public DescribeSnapshotsResponseBodySnapshots setSourceDiskId(String sourceDiskId) {
this.sourceDiskId = sourceDiskId;
return this;
}
public String getSourceDiskId() {
return this.sourceDiskId;
}
public DescribeSnapshotsResponseBodySnapshots setSourceDiskType(String sourceDiskType) {
this.sourceDiskType = sourceDiskType;
return this;
}
public String getSourceDiskType() {
return this.sourceDiskType;
}
public DescribeSnapshotsResponseBodySnapshots setSourceEnsRegionId(String sourceEnsRegionId) {
this.sourceEnsRegionId = sourceEnsRegionId;
return this;
}
public String getSourceEnsRegionId() {
return this.sourceEnsRegionId;
}
public DescribeSnapshotsResponseBodySnapshots setSourceSnapshotId(String sourceSnapshotId) {
this.sourceSnapshotId = sourceSnapshotId;
return this;
}
public String getSourceSnapshotId() {
return this.sourceSnapshotId;
}
public DescribeSnapshotsResponseBodySnapshots setStatus(String status) {
this.status = status;
return this;
}
public String getStatus() {
return this.status;
}
}
}