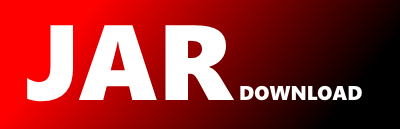
com.aliyun.ens20171110.models.DescribeSnatAttributeResponseBody Maven / Gradle / Ivy
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.ens20171110.models;
import com.aliyun.tea.*;
public class DescribeSnatAttributeResponseBody extends TeaModel {
/**
* The time when the entry was created. The time is displayed in UTC.
*
* example:
* 2020-04-26T15:38:27Z
*/
@NameInMap("CreationTime")
public String creationTime;
/**
* The destination CIDR block. The rule takes effect only on requests that access the destination CIDR block.
*
* example:
* 101.10. XX.XX/24
*/
@NameInMap("DestCIDR")
public String destCIDR;
/**
* The timeout period. Unit: seconds.
*
* example:
* 10
*/
@NameInMap("IdleTimeout")
public Integer idleTimeout;
@NameInMap("IspAffinity")
public Boolean ispAffinity;
/**
* The ID of the Network Address Translation (NAT) gateway.
*
* example:
* nat-5t7nh1cfm6kxiszlttr38****
*/
@NameInMap("NatGatewayId")
public String natGatewayId;
/**
* The ID of the request.
*
* example:
* 473469C7-AA6F-4DC5-B3DB-A3DC0DE3C83E
*/
@NameInMap("RequestId")
public String requestId;
/**
* The ID of the SNAT entry.
*
* example:
* snat-5tfi6f8gds82mjmlofeym****
*/
@NameInMap("SnatEntryId")
public String snatEntryId;
/**
* The name of the SNAT entry.
*
* example:
* test0
*/
@NameInMap("SnatEntryName")
public String snatEntryName;
/**
* The EIP specified in the SNAT entry. Multiple EIPs are separated by commas (,).
*
* example:
* 120.72.XX.XX
*/
@NameInMap("SnatIp")
public String snatIp;
/**
* The information about the EIP specified in the SNAT entry.
*/
@NameInMap("SnatIps")
public java.util.List snatIps;
/**
* The source CIDR block specified in the SNAT entry.
*
* example:
* 10.0.XX.XX/24
*/
@NameInMap("SourceCIDR")
public String sourceCIDR;
/**
* The secondary EIP specified in the SNAT entry. Multiple secondary EIPs are separated by commas (,).
*
* example:
* 101.23. XX.XX
*/
@NameInMap("StandbySnatIp")
public String standbySnatIp;
/**
* The status of the secondary EIP.
*
* - Running
* - Stopping
* - Stopped
* - Starting
*
*
* example:
* Stopped
*/
@NameInMap("StandbyStatus")
public String standbyStatus;
/**
* The status of the SNAT entry.
*
* - Pending: The SNAT entry is being created or modified.
* - Available: The SNAT entry is available.
* - Deleting: The SNAT entry is being deleted.
*
*
* example:
* Available
*/
@NameInMap("Status")
public String status;
/**
* The type of the NAT.
*
* - Empty: symmetric NAT.
* - FullCone: full cone NAT.
*
*
* example:
* FullCone
*/
@NameInMap("Type")
public String type;
public static DescribeSnatAttributeResponseBody build(java.util.Map map) throws Exception {
DescribeSnatAttributeResponseBody self = new DescribeSnatAttributeResponseBody();
return TeaModel.build(map, self);
}
public DescribeSnatAttributeResponseBody setCreationTime(String creationTime) {
this.creationTime = creationTime;
return this;
}
public String getCreationTime() {
return this.creationTime;
}
public DescribeSnatAttributeResponseBody setDestCIDR(String destCIDR) {
this.destCIDR = destCIDR;
return this;
}
public String getDestCIDR() {
return this.destCIDR;
}
public DescribeSnatAttributeResponseBody setIdleTimeout(Integer idleTimeout) {
this.idleTimeout = idleTimeout;
return this;
}
public Integer getIdleTimeout() {
return this.idleTimeout;
}
public DescribeSnatAttributeResponseBody setIspAffinity(Boolean ispAffinity) {
this.ispAffinity = ispAffinity;
return this;
}
public Boolean getIspAffinity() {
return this.ispAffinity;
}
public DescribeSnatAttributeResponseBody setNatGatewayId(String natGatewayId) {
this.natGatewayId = natGatewayId;
return this;
}
public String getNatGatewayId() {
return this.natGatewayId;
}
public DescribeSnatAttributeResponseBody setRequestId(String requestId) {
this.requestId = requestId;
return this;
}
public String getRequestId() {
return this.requestId;
}
public DescribeSnatAttributeResponseBody setSnatEntryId(String snatEntryId) {
this.snatEntryId = snatEntryId;
return this;
}
public String getSnatEntryId() {
return this.snatEntryId;
}
public DescribeSnatAttributeResponseBody setSnatEntryName(String snatEntryName) {
this.snatEntryName = snatEntryName;
return this;
}
public String getSnatEntryName() {
return this.snatEntryName;
}
public DescribeSnatAttributeResponseBody setSnatIp(String snatIp) {
this.snatIp = snatIp;
return this;
}
public String getSnatIp() {
return this.snatIp;
}
public DescribeSnatAttributeResponseBody setSnatIps(java.util.List snatIps) {
this.snatIps = snatIps;
return this;
}
public java.util.List getSnatIps() {
return this.snatIps;
}
public DescribeSnatAttributeResponseBody setSourceCIDR(String sourceCIDR) {
this.sourceCIDR = sourceCIDR;
return this;
}
public String getSourceCIDR() {
return this.sourceCIDR;
}
public DescribeSnatAttributeResponseBody setStandbySnatIp(String standbySnatIp) {
this.standbySnatIp = standbySnatIp;
return this;
}
public String getStandbySnatIp() {
return this.standbySnatIp;
}
public DescribeSnatAttributeResponseBody setStandbyStatus(String standbyStatus) {
this.standbyStatus = standbyStatus;
return this;
}
public String getStandbyStatus() {
return this.standbyStatus;
}
public DescribeSnatAttributeResponseBody setStatus(String status) {
this.status = status;
return this;
}
public String getStatus() {
return this.status;
}
public DescribeSnatAttributeResponseBody setType(String type) {
this.type = type;
return this;
}
public String getType() {
return this.type;
}
public static class DescribeSnatAttributeResponseBodySnatIps extends TeaModel {
/**
* The time when the IP address was created. The time is displayed in UTC.
*
* example:
* 2020-04-26T15:38:27Z
*/
@NameInMap("CreationTime")
public String creationTime;
/**
* The IP address.
*
* example:
* 203.132.XX.XX
*/
@NameInMap("Ip")
public String ip;
/**
* The status of the IP address.
*
* - Running
* - Stopping
* - Stopped
* - Starting
* - Releasing
*
*
* example:
* Running
*/
@NameInMap("Status")
public String status;
public static DescribeSnatAttributeResponseBodySnatIps build(java.util.Map map) throws Exception {
DescribeSnatAttributeResponseBodySnatIps self = new DescribeSnatAttributeResponseBodySnatIps();
return TeaModel.build(map, self);
}
public DescribeSnatAttributeResponseBodySnatIps setCreationTime(String creationTime) {
this.creationTime = creationTime;
return this;
}
public String getCreationTime() {
return this.creationTime;
}
public DescribeSnatAttributeResponseBodySnatIps setIp(String ip) {
this.ip = ip;
return this;
}
public String getIp() {
return this.ip;
}
public DescribeSnatAttributeResponseBodySnatIps setStatus(String status) {
this.status = status;
return this;
}
public String getStatus() {
return this.status;
}
}
}