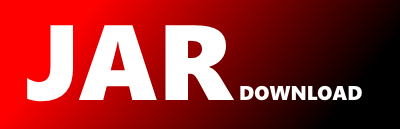
com.aliyun.ens20171110.models.CreateSnatEntryRequest Maven / Gradle / Ivy
Show all versions of ens20171110 Show documentation
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.ens20171110.models;
import com.aliyun.tea.*;
public class CreateSnatEntryRequest extends TeaModel {
/**
* The timeout period for idle connections. Valid values: 1 to 86400. Unit: seconds.
*
* example:
* 15
*/
@NameInMap("IdleTimeout")
public Integer idleTimeout;
@NameInMap("IspAffinity")
public Boolean ispAffinity;
/**
* The ID of the Network Address Translation (NAT) gateway.
* This parameter is required.
*
* example:
* nat-5tawjw5j7sgd2deujxuk0****
*/
@NameInMap("NatGatewayId")
public String natGatewayId;
/**
* The name of the SNAT entry. The name must be 1 to 128 characters in length. The name cannot start with http://
or https://
.
*
* example:
* test0
*/
@NameInMap("SnatEntryName")
public String snatEntryName;
/**
* The elastic IP address (EIP) in the SNAT entry. Separate multiple EIPs with commas (,).
* This parameter is required.
*
* example:
* 120.XXX.XXX.71
*/
@NameInMap("SnatIp")
public String snatIp;
/**
* The CIDR block. You can specify the CIDR block of a network, a vSwitch, or an instance. You can also specify a custom CIDR block. All instances within the CIDR block can access the Internet or external networks by using SNAT.
*
* If you specify SourceVSwitchId and SourceCIDR, SourceVSwitchId does not take effect. The value that you specified for SourceCIDR takes precedence.
*
*
* example:
* 10.0.0.0/24
*/
@NameInMap("SourceCIDR")
public String sourceCIDR;
/**
* The ID of the network. This parameter specifies that all ENS instances in the network can use the SNAT entry to access the Internet.
*
* If you specify SourceNetworkId and SourceVSwitchId or SourceCIDR, SourceNetworkId does not take effect. The value that you specified for SourceCIDR takes precedence. Priority: SourceCIDR > SourceVSwitchId > SourceNetworkId.
*
*
* example:
* n-2zeuphj08tt7q3brd****
*/
@NameInMap("SourceNetworkId")
public String sourceNetworkId;
/**
* The ID of the vSwitch that you need to access over the Internet. This parameter specifies that Edge Node Service (ENS) instances in the vSwitch can use the SNAT entry to access the Internet.
*
* If you specify SourceVSwitchId and SourceCIDR, SourceVSwitchId does not take effect. The value that you specified for SourceCIDR takes precedence.
*
*
* example:
* vsw-bp1hwx7gi495q260p****
*/
@NameInMap("SourceVSwitchId")
public String sourceVSwitchId;
/**
* The secondary EIP in the SNAT entry. Separate multiple secondary EIPs with commas (,).
*
* example:
* 101.XXX.XXX.7
*/
@NameInMap("StandbySnatIp")
public String standbySnatIp;
public static CreateSnatEntryRequest build(java.util.Map map) throws Exception {
CreateSnatEntryRequest self = new CreateSnatEntryRequest();
return TeaModel.build(map, self);
}
public CreateSnatEntryRequest setIdleTimeout(Integer idleTimeout) {
this.idleTimeout = idleTimeout;
return this;
}
public Integer getIdleTimeout() {
return this.idleTimeout;
}
public CreateSnatEntryRequest setIspAffinity(Boolean ispAffinity) {
this.ispAffinity = ispAffinity;
return this;
}
public Boolean getIspAffinity() {
return this.ispAffinity;
}
public CreateSnatEntryRequest setNatGatewayId(String natGatewayId) {
this.natGatewayId = natGatewayId;
return this;
}
public String getNatGatewayId() {
return this.natGatewayId;
}
public CreateSnatEntryRequest setSnatEntryName(String snatEntryName) {
this.snatEntryName = snatEntryName;
return this;
}
public String getSnatEntryName() {
return this.snatEntryName;
}
public CreateSnatEntryRequest setSnatIp(String snatIp) {
this.snatIp = snatIp;
return this;
}
public String getSnatIp() {
return this.snatIp;
}
public CreateSnatEntryRequest setSourceCIDR(String sourceCIDR) {
this.sourceCIDR = sourceCIDR;
return this;
}
public String getSourceCIDR() {
return this.sourceCIDR;
}
public CreateSnatEntryRequest setSourceNetworkId(String sourceNetworkId) {
this.sourceNetworkId = sourceNetworkId;
return this;
}
public String getSourceNetworkId() {
return this.sourceNetworkId;
}
public CreateSnatEntryRequest setSourceVSwitchId(String sourceVSwitchId) {
this.sourceVSwitchId = sourceVSwitchId;
return this;
}
public String getSourceVSwitchId() {
return this.sourceVSwitchId;
}
public CreateSnatEntryRequest setStandbySnatIp(String standbySnatIp) {
this.standbySnatIp = standbySnatIp;
return this;
}
public String getStandbySnatIp() {
return this.standbySnatIp;
}
}