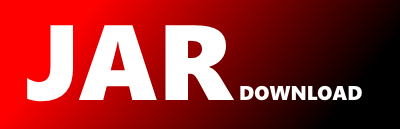
com.aliyun.ens20171110.models.DescribeLoadBalancerAttributeResponseBody Maven / Gradle / Ivy
Show all versions of ens20171110 Show documentation
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.ens20171110.models;
import com.aliyun.tea.*;
public class DescribeLoadBalancerAttributeResponseBody extends TeaModel {
/**
* The IP address that the Edge Load Balancer (ELB) instance uses to provide services.
*
* example:
* 192.168XX.XX
*/
@NameInMap("Address")
public String address;
/**
* The IP version of the ELB instance. Valid values: ipv4 and ipv6.
*
* example:
* ipv4
*/
@NameInMap("AddressIPVersion")
public String addressIPVersion;
/**
* The list of backend servers.
*/
@NameInMap("BackendServers")
public java.util.List backendServers;
/**
* The peak bandwidth of the ELB. The default value is -1, which indicates that the bandwidth is unlimited.
*
* example:
* -1
*/
@NameInMap("Bandwidth")
public Integer bandwidth;
/**
* The time when the ELB instance was created. The time is displayed in UTC.
*
* example:
* 2019-05-21T12:22:00Z
*/
@NameInMap("CreateTime")
public String createTime;
/**
* The time when the ELB instance was disabled.
*
* example:
* 2020-05-21T12:22:00Z
*/
@NameInMap("EndTime")
public String endTime;
/**
* The ID of the ENS node.
*
* example:
* cn-chengdu-telecom
*/
@NameInMap("EnsRegionId")
public String ensRegionId;
/**
* The frontend ports that are used by the ELB instance.
*/
@NameInMap("ListenerPorts")
public java.util.List listenerPorts;
/**
* The frontend ports and protocols that are used by the ELB instance.
*/
@NameInMap("ListenerPortsAndProtocols")
public java.util.List listenerPortsAndProtocols;
/**
* The ID of the ELB instance.
*
* example:
* lb-5rcvo1n1t3hykfhhjwjgqp****
*/
@NameInMap("LoadBalancerId")
public String loadBalancerId;
/**
* The name of the ELB instance.
*
* example:
* example
*/
@NameInMap("LoadBalancerName")
public String loadBalancerName;
/**
* The specifications of the ELB instance.
*
* example:
* elb.s2.medium
*/
@NameInMap("LoadBalancerSpec")
public String loadBalancerSpec;
/**
* The status of the ELB instance. Valid values:
*
* - Active (default): The listener for the instance can forward the received traffic based on the rule.
* - InActive: The listener for the instance does not forward the received traffic.
*
*
* example:
* Active
*/
@NameInMap("LoadBalancerStatus")
public String loadBalancerStatus;
/**
* The ID of the network.
*
* example:
* n-5rwbi3g9zvgxcsiufwhw8****
*/
@NameInMap("NetworkId")
public String networkId;
/**
* The billing method. Valid values:
*
* - PrePaid: subscription.
* - PostPaid: pay-as-you-go. Only this billing method is supported.
*
*
* example:
* PostPaid
*/
@NameInMap("PayType")
public String payType;
/**
* The ID of the request.
*
* example:
* 473469C7-AA6F-4DC5-B3DB-A3DC0DE3C83E
*/
@NameInMap("RequestId")
public String requestId;
/**
* The ID of the vSwitch.
*
* example:
* vsw-5s78m2pdr9osa0j64bn78****
*/
@NameInMap("VSwitchId")
public String vSwitchId;
public static DescribeLoadBalancerAttributeResponseBody build(java.util.Map map) throws Exception {
DescribeLoadBalancerAttributeResponseBody self = new DescribeLoadBalancerAttributeResponseBody();
return TeaModel.build(map, self);
}
public DescribeLoadBalancerAttributeResponseBody setAddress(String address) {
this.address = address;
return this;
}
public String getAddress() {
return this.address;
}
public DescribeLoadBalancerAttributeResponseBody setAddressIPVersion(String addressIPVersion) {
this.addressIPVersion = addressIPVersion;
return this;
}
public String getAddressIPVersion() {
return this.addressIPVersion;
}
public DescribeLoadBalancerAttributeResponseBody setBackendServers(java.util.List backendServers) {
this.backendServers = backendServers;
return this;
}
public java.util.List getBackendServers() {
return this.backendServers;
}
public DescribeLoadBalancerAttributeResponseBody setBandwidth(Integer bandwidth) {
this.bandwidth = bandwidth;
return this;
}
public Integer getBandwidth() {
return this.bandwidth;
}
public DescribeLoadBalancerAttributeResponseBody setCreateTime(String createTime) {
this.createTime = createTime;
return this;
}
public String getCreateTime() {
return this.createTime;
}
public DescribeLoadBalancerAttributeResponseBody setEndTime(String endTime) {
this.endTime = endTime;
return this;
}
public String getEndTime() {
return this.endTime;
}
public DescribeLoadBalancerAttributeResponseBody setEnsRegionId(String ensRegionId) {
this.ensRegionId = ensRegionId;
return this;
}
public String getEnsRegionId() {
return this.ensRegionId;
}
public DescribeLoadBalancerAttributeResponseBody setListenerPorts(java.util.List listenerPorts) {
this.listenerPorts = listenerPorts;
return this;
}
public java.util.List getListenerPorts() {
return this.listenerPorts;
}
public DescribeLoadBalancerAttributeResponseBody setListenerPortsAndProtocols(java.util.List listenerPortsAndProtocols) {
this.listenerPortsAndProtocols = listenerPortsAndProtocols;
return this;
}
public java.util.List getListenerPortsAndProtocols() {
return this.listenerPortsAndProtocols;
}
public DescribeLoadBalancerAttributeResponseBody setLoadBalancerId(String loadBalancerId) {
this.loadBalancerId = loadBalancerId;
return this;
}
public String getLoadBalancerId() {
return this.loadBalancerId;
}
public DescribeLoadBalancerAttributeResponseBody setLoadBalancerName(String loadBalancerName) {
this.loadBalancerName = loadBalancerName;
return this;
}
public String getLoadBalancerName() {
return this.loadBalancerName;
}
public DescribeLoadBalancerAttributeResponseBody setLoadBalancerSpec(String loadBalancerSpec) {
this.loadBalancerSpec = loadBalancerSpec;
return this;
}
public String getLoadBalancerSpec() {
return this.loadBalancerSpec;
}
public DescribeLoadBalancerAttributeResponseBody setLoadBalancerStatus(String loadBalancerStatus) {
this.loadBalancerStatus = loadBalancerStatus;
return this;
}
public String getLoadBalancerStatus() {
return this.loadBalancerStatus;
}
public DescribeLoadBalancerAttributeResponseBody setNetworkId(String networkId) {
this.networkId = networkId;
return this;
}
public String getNetworkId() {
return this.networkId;
}
public DescribeLoadBalancerAttributeResponseBody setPayType(String payType) {
this.payType = payType;
return this;
}
public String getPayType() {
return this.payType;
}
public DescribeLoadBalancerAttributeResponseBody setRequestId(String requestId) {
this.requestId = requestId;
return this;
}
public String getRequestId() {
return this.requestId;
}
public DescribeLoadBalancerAttributeResponseBody setVSwitchId(String vSwitchId) {
this.vSwitchId = vSwitchId;
return this;
}
public String getVSwitchId() {
return this.vSwitchId;
}
public static class DescribeLoadBalancerAttributeResponseBodyBackendServers extends TeaModel {
/**
* The IP address of the backend server.
*
* example:
* 192.168.XX.XX
*/
@NameInMap("Ip")
public String ip;
/**
* The port that is used by the backend server.
*
* example:
* 0
*/
@NameInMap("Port")
public String port;
/**
* The ID of the backend server.
*
* example:
* i-5vb5h5njxiuhn48a****
*/
@NameInMap("ServerId")
public String serverId;
/**
* The type of backend server.
*
* example:
* ens
*/
@NameInMap("Type")
public String type;
/**
* The weight of the backend server.
*
* example:
* 100
*/
@NameInMap("Weight")
public Integer weight;
public static DescribeLoadBalancerAttributeResponseBodyBackendServers build(java.util.Map map) throws Exception {
DescribeLoadBalancerAttributeResponseBodyBackendServers self = new DescribeLoadBalancerAttributeResponseBodyBackendServers();
return TeaModel.build(map, self);
}
public DescribeLoadBalancerAttributeResponseBodyBackendServers setIp(String ip) {
this.ip = ip;
return this;
}
public String getIp() {
return this.ip;
}
public DescribeLoadBalancerAttributeResponseBodyBackendServers setPort(String port) {
this.port = port;
return this;
}
public String getPort() {
return this.port;
}
public DescribeLoadBalancerAttributeResponseBodyBackendServers setServerId(String serverId) {
this.serverId = serverId;
return this;
}
public String getServerId() {
return this.serverId;
}
public DescribeLoadBalancerAttributeResponseBodyBackendServers setType(String type) {
this.type = type;
return this;
}
public String getType() {
return this.type;
}
public DescribeLoadBalancerAttributeResponseBodyBackendServers setWeight(Integer weight) {
this.weight = weight;
return this;
}
public Integer getWeight() {
return this.weight;
}
}
public static class DescribeLoadBalancerAttributeResponseBodyListenerPortsAndProtocols extends TeaModel {
/**
* The description of the listener.
*
* example:
* test
*/
@NameInMap("Description")
public String description;
/**
* The destination listening port to which requests are forwarded.
*
* example:
* 0
*/
@NameInMap("ForwardPort")
public Integer forwardPort;
/**
* Indicates whether the listener is enabled.
*
* example:
* off
*/
@NameInMap("ListenerForward")
public String listenerForward;
/**
* The listener port of the instance.
*
* example:
* 8080
*/
@NameInMap("ListenerPort")
public Integer listenerPort;
/**
* The listener protocol of the instance.
*
* example:
* tcp
*/
@NameInMap("ListenerProtocol")
public String listenerProtocol;
public static DescribeLoadBalancerAttributeResponseBodyListenerPortsAndProtocols build(java.util.Map map) throws Exception {
DescribeLoadBalancerAttributeResponseBodyListenerPortsAndProtocols self = new DescribeLoadBalancerAttributeResponseBodyListenerPortsAndProtocols();
return TeaModel.build(map, self);
}
public DescribeLoadBalancerAttributeResponseBodyListenerPortsAndProtocols setDescription(String description) {
this.description = description;
return this;
}
public String getDescription() {
return this.description;
}
public DescribeLoadBalancerAttributeResponseBodyListenerPortsAndProtocols setForwardPort(Integer forwardPort) {
this.forwardPort = forwardPort;
return this;
}
public Integer getForwardPort() {
return this.forwardPort;
}
public DescribeLoadBalancerAttributeResponseBodyListenerPortsAndProtocols setListenerForward(String listenerForward) {
this.listenerForward = listenerForward;
return this;
}
public String getListenerForward() {
return this.listenerForward;
}
public DescribeLoadBalancerAttributeResponseBodyListenerPortsAndProtocols setListenerPort(Integer listenerPort) {
this.listenerPort = listenerPort;
return this;
}
public Integer getListenerPort() {
return this.listenerPort;
}
public DescribeLoadBalancerAttributeResponseBodyListenerPortsAndProtocols setListenerProtocol(String listenerProtocol) {
this.listenerProtocol = listenerProtocol;
return this;
}
public String getListenerProtocol() {
return this.listenerProtocol;
}
}
}