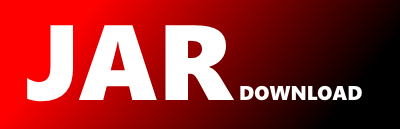
com.aliyun.goodstech.Client Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of goodstech Show documentation
Show all versions of goodstech Show documentation
Aliyun GOODSTECH SDK for Java
Copyright (C) Alibaba Cloud Computing
All rights reserved.
版权所有 (C)阿里云计算有限公司
http://www.aliyun.com
// This file is auto-generated, don't edit it. Thanks.
package com.aliyun.goodstech;
import com.aliyun.tea.*;
import com.aliyun.goodstech.models.*;
public class Client {
public String _endpoint;
public String _regionId;
public String _protocol;
public String _userAgent;
public String _endpointType;
public Integer _readTimeout;
public Integer _connectTimeout;
public String _httpProxy;
public String _httpsProxy;
public String _socks5Proxy;
public String _socks5NetWork;
public String _noProxy;
public Integer _maxIdleConns;
public String _openPlatformEndpoint;
public com.aliyun.credentials.Client _credential;
public Client(Config config) throws Exception {
if (com.aliyun.teautil.Common.isUnset(TeaModel.buildMap(config))) {
throw new TeaException(TeaConverter.buildMap(
new TeaPair("name", "ParameterMissing"),
new TeaPair("message", "'config' can not be unset")
));
}
if (com.aliyun.teautil.Common.empty(config.regionId)) {
throw new TeaException(TeaConverter.buildMap(
new TeaPair("name", "ParameterMissing"),
new TeaPair("message", "'config.regionId' can not be empty")
));
}
if (com.aliyun.teautil.Common.empty(config.endpoint)) {
throw new TeaException(TeaConverter.buildMap(
new TeaPair("name", "ParameterMissing"),
new TeaPair("message", "'config.endpoint' can not be empty")
));
}
if (com.aliyun.teautil.Common.empty(config.type)) {
config.type = "access_key";
}
com.aliyun.credentials.models.Config credentialConfig = com.aliyun.credentials.models.Config.build(TeaConverter.buildMap(
new TeaPair("accessKeyId", config.accessKeyId),
new TeaPair("type", config.type),
new TeaPair("accessKeySecret", config.accessKeySecret),
new TeaPair("securityToken", config.securityToken)
));
this._credential = new com.aliyun.credentials.Client(credentialConfig);
this._endpoint = config.endpoint;
this._protocol = config.protocol;
this._regionId = config.regionId;
this._userAgent = config.userAgent;
this._readTimeout = config.readTimeout;
this._connectTimeout = config.connectTimeout;
this._httpProxy = config.httpProxy;
this._httpsProxy = config.httpsProxy;
this._noProxy = config.noProxy;
this._socks5Proxy = config.socks5Proxy;
this._socks5NetWork = config.socks5NetWork;
this._maxIdleConns = config.maxIdleConns;
this._endpointType = config.endpointType;
this._openPlatformEndpoint = config.openPlatformEndpoint;
}
public java.util.Map _request(String action, String protocol, String method, java.util.Map request, com.aliyun.teautil.models.RuntimeOptions runtime) throws Exception {
java.util.Map runtime_ = TeaConverter.buildMap(
new TeaPair("timeouted", "retry"),
new TeaPair("readTimeout", com.aliyun.teautil.Common.defaultNumber(runtime.readTimeout, _readTimeout)),
new TeaPair("connectTimeout", com.aliyun.teautil.Common.defaultNumber(runtime.connectTimeout, _connectTimeout)),
new TeaPair("httpProxy", com.aliyun.teautil.Common.defaultString(runtime.httpProxy, _httpProxy)),
new TeaPair("httpsProxy", com.aliyun.teautil.Common.defaultString(runtime.httpsProxy, _httpsProxy)),
new TeaPair("noProxy", com.aliyun.teautil.Common.defaultString(runtime.noProxy, _noProxy)),
new TeaPair("maxIdleConns", com.aliyun.teautil.Common.defaultNumber(runtime.maxIdleConns, _maxIdleConns)),
new TeaPair("retry", TeaConverter.buildMap(
new TeaPair("retryable", runtime.autoretry),
new TeaPair("maxAttempts", com.aliyun.teautil.Common.defaultNumber(runtime.maxAttempts, 3))
)),
new TeaPair("backoff", TeaConverter.buildMap(
new TeaPair("policy", com.aliyun.teautil.Common.defaultString(runtime.backoffPolicy, "no")),
new TeaPair("period", com.aliyun.teautil.Common.defaultNumber(runtime.backoffPeriod, 1))
)),
new TeaPair("ignoreSSL", runtime.ignoreSSL)
);
TeaRequest _lastRequest = null;
long _now = System.currentTimeMillis();
int _retryTimes = 0;
while (Tea.allowRetry((java.util.Map) runtime_.get("retry"), _retryTimes, _now)) {
if (_retryTimes > 0) {
int backoffTime = Tea.getBackoffTime(runtime_.get("backoff"), _retryTimes);
if (backoffTime > 0) {
Tea.sleep(backoffTime);
}
}
_retryTimes = _retryTimes + 1;
try {
TeaRequest request_ = new TeaRequest();
request_.protocol = com.aliyun.teautil.Common.defaultString(_protocol, protocol);
request_.method = method;
request_.pathname = "/";
String accessKeyId = this.getAccessKeyId();
String accessKeySecret = this.getAccessKeySecret();
request_.query = com.aliyun.common.Common.query(TeaConverter.merge(Object.class,
TeaConverter.buildMap(
new TeaPair("Action", action),
new TeaPair("Format", "json"),
new TeaPair("RegionId", _regionId),
new TeaPair("Timestamp", com.aliyun.common.Common.getTimestamp()),
new TeaPair("Version", "2019-12-30"),
new TeaPair("SignatureMethod", "HMAC-SHA1"),
new TeaPair("SignatureVersion", "1.0"),
new TeaPair("SignatureNonce", com.aliyun.teautil.Common.getNonce()),
new TeaPair("AccessKeyId", accessKeyId)
),
request
));
request_.headers = TeaConverter.buildMap(
new TeaPair("host", com.aliyun.common.Common.getHost("goodstech", _regionId, _endpoint)),
new TeaPair("user-agent", this.getUserAgent())
);
request_.query.put("Signature", com.aliyun.common.Common.getSignature(request_, accessKeySecret));
_lastRequest = request_;
TeaResponse response_ = Tea.doAction(request_, runtime_);
Object obj = com.aliyun.teautil.Common.readAsJSON(response_.body);
java.util.Map body = com.aliyun.teautil.Common.assertAsMap(obj);
if (com.aliyun.teautil.Common.is4xx(response_.statusCode) || com.aliyun.teautil.Common.is5xx(response_.statusCode)) {
throw new TeaException(TeaConverter.buildMap(
new TeaPair("message", body.get("Message")),
new TeaPair("data", body),
new TeaPair("code", body.get("Code"))
));
}
return body;
} catch (Exception e) {
if (Tea.isRetryable(e)) {
continue;
}
throw e;
}
}
throw new TeaUnretryableException(_lastRequest);
}
public ClassifyCommodityResponse classifyCommodity(ClassifyCommodityRequest request, com.aliyun.teautil.models.RuntimeOptions runtime) throws Exception {
return TeaModel.toModel(this._request("ClassifyCommodity", "HTTPS", "GET", TeaModel.buildMap(request), runtime), new ClassifyCommodityResponse());
}
public ClassifyCommodityResponse classifyCommodityAdvance(ClassifyCommodityAdvanceRequest request, com.aliyun.teautil.models.RuntimeOptions runtime) throws Exception {
String accessKeyId = _credential.getAccessKeyId();
String accessKeySecret = _credential.getAccessKeySecret();
com.aliyun.openplatform.models.Config authConfig = com.aliyun.openplatform.models.Config.build(TeaConverter.buildMap(
new TeaPair("accessKeyId", accessKeyId),
new TeaPair("accessKeySecret", accessKeySecret),
new TeaPair("type", "access_key"),
new TeaPair("endpoint", "openplatform.aliyuncs.com"),
new TeaPair("protocol", _protocol),
new TeaPair("regionId", _regionId)
));
com.aliyun.openplatform.Client authClient = new com.aliyun.openplatform.Client(authConfig);
com.aliyun.openplatform.models.AuthorizeFileUploadRequest authRequest = com.aliyun.openplatform.models.AuthorizeFileUploadRequest.build(TeaConverter.buildMap(
new TeaPair("product", "goodstech"),
new TeaPair("regionId", _regionId)
));
com.aliyun.openplatform.models.AuthorizeFileUploadResponse authResponse = authClient.authorizeFileUpload(authRequest, runtime);
com.aliyun.oss.models.Config ossConfig = com.aliyun.oss.models.Config.build(TeaConverter.buildMap(
new TeaPair("accessKeyId", authResponse.accessKeyId),
new TeaPair("accessKeySecret", accessKeySecret),
new TeaPair("type", "access_key"),
new TeaPair("endpoint", com.aliyun.common.Common.getEndpoint(authResponse.endpoint, authResponse.useAccelerate, _endpointType)),
new TeaPair("protocol", _protocol),
new TeaPair("regionId", _regionId)
));
com.aliyun.oss.Client ossClient = new com.aliyun.oss.Client(ossConfig);
com.aliyun.fileform.models.FileField fileObj = com.aliyun.fileform.models.FileField.build(TeaConverter.buildMap(
new TeaPair("filename", authResponse.objectKey),
new TeaPair("content", request.imageURLObject),
new TeaPair("contentType", "")
));
com.aliyun.oss.models.PostObjectRequest.PostObjectRequestHeader ossHeader = com.aliyun.oss.models.PostObjectRequest.PostObjectRequestHeader.build(TeaConverter.buildMap(
new TeaPair("accessKeyId", authResponse.accessKeyId),
new TeaPair("policy", authResponse.encodedPolicy),
new TeaPair("signature", authResponse.signature),
new TeaPair("key", authResponse.objectKey),
new TeaPair("file", fileObj),
new TeaPair("successActionStatus", "201")
));
com.aliyun.oss.models.PostObjectRequest uploadRequest = com.aliyun.oss.models.PostObjectRequest.build(TeaConverter.buildMap(
new TeaPair("bucketName", authResponse.bucket),
new TeaPair("header", ossHeader)
));
com.aliyun.ossutil.models.RuntimeOptions ossRuntime = new com.aliyun.ossutil.models.RuntimeOptions();
com.aliyun.common.Common.convert(runtime, ossRuntime);
ossClient.postObject(uploadRequest, ossRuntime);
ClassifyCommodityRequest classifyCommodityreq = new ClassifyCommodityRequest();
com.aliyun.common.Common.convert(request, classifyCommodityreq);
classifyCommodityreq.imageURL = "http://" + authResponse.bucket + "." + authResponse.endpoint + "/" + authResponse.objectKey + "";
ClassifyCommodityResponse classifyCommodityResp = this.classifyCommodity(classifyCommodityreq, runtime);
return classifyCommodityResp;
}
public String getUserAgent() throws Exception {
String userAgent = com.aliyun.teautil.Common.getUserAgent(_userAgent);
return userAgent;
}
public String getAccessKeyId() throws Exception {
if (com.aliyun.teautil.Common.isUnset(_credential)) {
return "";
}
String accessKeyId = _credential.getAccessKeyId();
return accessKeyId;
}
public String getAccessKeySecret() throws Exception {
if (com.aliyun.teautil.Common.isUnset(_credential)) {
return "";
}
String secret = _credential.getAccessKeySecret();
return secret;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy